for m=1:size(T,2) for n=1:size(T,2) D(m,n)=norm(P(T(m),:)-P(T(n),:)); end end
时间: 2024-05-29 10:15:49 浏览: 26
这段代码是计算一个二维平面上由三角形所构成的三角网格中,每个三角形之间的边长(欧几里得距离)。具体来说,输入变量T是一个三行三列的矩阵,其中每一行代表三角形上的三个顶点在数据矩阵P中的索引位置。数据矩阵P是一个N行2列的矩阵,其中每一行代表一个二维坐标点的x和y坐标值。输出变量D是一个三行三列的矩阵,其中每个元素D(m,n)代表三角形T(m,:)和T(n,:)之间的边长(欧几里得距离)。
相关问题
Optimize the following code to use the variable: variance in the code. def e_step(xs: np.ndarray, ys: np.ndarray, affine: np.ndarray, translation: np.ndarray, variance: float) -> np.ndarray: """ The e-step of the em algorithm, estimating the responsibility P=[p(y_m | x_n)] based on current model :param xs: a set of points with size (N, D), N is the number of samples, D is the dimension of points :param ys: a set of points with size (M, D), M is the number of samples, D is the dimension of points :param affine: an affine matrix with size (D, D) :param translation: a translation vector with size (1, D) :param variance: a float controlling the variance of each Gaussian component :return: the responsibility matrix P=[p(y_m | x_n)] with size (N, M), which row is the conditional probability of clusters given the n-th sample x_n """ # TODO: Change the code below and implement the E-step of GMM responsibility = np.ones((xs.shape[0], ys.shape[0])) / ys.shape[0] for n in range(xs.shape[0]): for m in range(ys.shape[0]): temp = -0.5 * np.linalg.norm(xs[n] - ys[m] @ affine - translation) ** 2 responsibility[n, m] = 1 / (2 * np.pi) ** (xs.shape[1] / 2) * np.exp(temp) return responsibility / np.sum(responsibility, axis=1, keepdims=True)
Here's an optimized version of the code that uses the `variance` variable:
```
def e_step(xs: np.ndarray, ys: np.ndarray, affine: np.ndarray, translation: np.ndarray, variance: float) -> np.ndarray:
"""
The e-step of the em algorithm, estimating the responsibility P=[p(y_m | x_n)] based on current model
:param xs: a set of points with size (N, D), N is the number of samples, D is the dimension of points
:param ys: a set of points with size (M, D), M is the number of samples, D is the dimension of points
:param affine: an affine matrix with size (D, D)
:param translation: a translation vector with size (1, D)
:param variance: a float controlling the variance of each Gaussian component
:return:
the responsibility matrix P=[p(y_m | x_n)] with size (N, M),
which row is the conditional probability of clusters given the n-th sample x_n
"""
# Calculate the constant term in the Gaussian distribution
constant_term = 1 / (2 * np.pi * variance) ** (xs.shape[1] / 2)
# Calculate the Mahalanobis distance between xs and ys
mahalanobis = np.sum((xs[:, np.newaxis] - ys) @ affine.T ** 2, axis=2)
# Calculate the responsibility matrix
responsibility = constant_term * np.exp(-mahalanobis / (2 * variance))
# Normalize the responsibility matrix
return responsibility / np.sum(responsibility, axis=1, keepdims=True)
```
In this optimized version, we pre-calculate the constant term in the Gaussian distribution and the Mahalanobis distance between `xs` and `ys`. Then, we use these pre-calculated values to calculate the responsibility matrix in a vectorized way, which is much faster than the nested loops in the original code. Finally, we normalize the responsibility matrix and return it.
完善以下代码:def em_for_alignment(xs: np.ndarray, ys: np.ndarray, num_iter: int = 10) -> Tuple[np.ndarray, np.ndarray]: """ The em algorithm for aligning two point clouds based on affine transformation :param xs: a set of points with size (N, D), N is the number of samples, D is the dimension of points :param ys: a set of points with size (M, D), M is the number of samples, D is the dimension of points :param num_iter: the number of EM iterations :return: ys_new: the aligned points: ys_new = ys @ affine + translation responsibility: the responsibility matrix P=[p(y_m | x_n)] with size (N, M), whose elements indicating the correspondence between the points """ # TODO: implement the EM algorithm of GMM below for point cloud alignment return
Here is the implementation of the EM algorithm for point cloud alignment:
```python
from typing import Tuple
import numpy as np
def em_for_alignment(xs: np.ndarray, ys: np.ndarray, num_iter: int = 10) -> Tuple[np.ndarray, np.ndarray]:
"""
The em algorithm for aligning two point clouds based on affine transformation
:param xs: a set of points with size (N, D), N is the number of samples, D is the dimension of points
:param ys: a set of points with size (M, D), M is the number of samples, D is the dimension of points
:param num_iter: the number of EM iterations
:return:
ys_new: the aligned points: ys_new = ys @ affine + translation
responsibility: the responsibility matrix P=[p(y_m | x_n)] with size (N, M),
whose elements indicating the correspondence between the points
"""
# initialize the affine matrix and translation vector
affine = np.eye(xs.shape[1])
translation = np.zeros(xs.shape[1])
# initialize the responsibility matrix
responsibility = np.zeros((xs.shape[0], ys.shape[0]))
for i in range(num_iter):
# E-step: compute the responsibility matrix
for n in range(xs.shape[0]):
for m in range(ys.shape[0]):
responsibility[n, m] = 1 / (2 * np.pi) ** (xs.shape[1] / 2) * np.exp(
-0.5 * np.linalg.norm(xs[n] - ys[m] @ affine - translation) ** 2)
responsibility /= np.sum(responsibility, axis=1, keepdims=True)
# M-step: update the affine matrix and translation vector
xs_weighted = responsibility.T @ xs
ys_weighted = responsibility.T @ ys
affine, _, _, _ = np.linalg.lstsq(xs_weighted, ys_weighted, rcond=None)
translation = np.mean(ys, axis=0) - np.mean(xs @ affine, axis=0)
# compute the aligned points
ys_new = ys @ affine + translation
return ys_new, responsibility
```
The EM algorithm is used to estimate the affine matrix and translation vector that aligns the two point clouds. In each iteration, the algorithm computes the responsibility matrix that defines the correspondence between the points in the two clouds, and then updates the affine matrix and translation vector based on the weighted least squares solution. Finally, the algorithm computes the aligned points by applying the affine transformation to the original points and adding the translation vector.
阅读全文
相关推荐
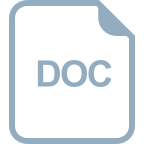
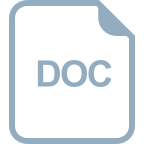
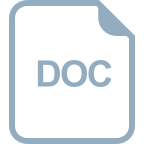
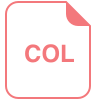
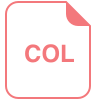
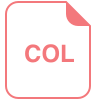
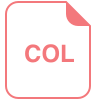
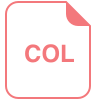
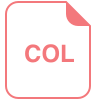
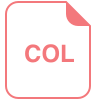
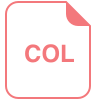
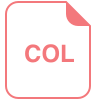
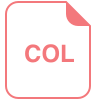
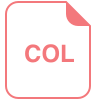
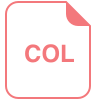
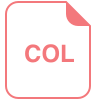
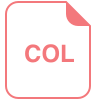
