style-transfer-models 下载
时间: 2023-05-14 10:02:54 浏览: 146
Style Transfer Models是一种深度学习模型,可实现图像风格转移。该模型的实现需要借助TensorFlow和Python等编程语言,具体的步骤包括下载、训练和应用。
首先,用户需要选择下载合适的Style Transfer Models的模型,比如VGG19、Inception V3等,通常这些预训练模型已经包含了大量数据集的图像样本,可以直接使用。然后,用户需要下载相关的Python代码和依赖项,如numpy、scipy、Pillow、matplotlib、tensorflow等。
接下来,用户可以使用下载好的模型来进行训练,以获取更加适合自己的风格转移模型。这里需要根据具体需求和实际情况,进行参数设定、数据集准备和训练数据的导入等步骤。训练过程需要较大的计算资源和时间,但是对于效果的改善和模型的优化具有非常重要的作用。
最后,通过下载好的模型和代码,用户可以将其应用于自己的图像风格转移,以得到美好的效果。当然,应用前还需要根据具体需求进行参数的设置,以获得最佳的效果。
总体而言,Style Transfer Models是一种独特的图像处理技术,通过下载、训练和应用,可以实现艺术风格和照片的有机结合,具有广泛的应用前景。
相关问题
styleTransfer代码中与神经网络训练过程五个步骤相对应的代码
在神经网络的训练过程中,通常需要进行以下五个步骤:准备数据、定义模型、定义损失函数、定义优化器、开始训练。下面是一份使用PyTorch实现style transfer的代码,其中与这五个步骤相对应的代码部分已经用注释标出。
```python
import torch
import torch.nn as nn
import torchvision.transforms as transforms
import torchvision.models as models
from PIL import Image
# 准备数据
transform = transforms.Compose([
transforms.Resize(512), # 调整图像大小
transforms.ToTensor(), # 将图像转换为Tensor
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225]) # 标准化图像
])
# 定义模型
class VGG(nn.Module):
def __init__(self):
super(VGG, self).__init__()
self.features = models.vgg19(pretrained=True).features[:35] # 选择VGG19模型的前35层作为特征提取器
def forward(self, x):
return self.features(x)
# 定义损失函数
class StyleLoss(nn.Module):
def __init__(self, target_feature):
super(StyleLoss, self).__init__()
self.target = self.gram_matrix(target_feature).detach()
def forward(self, input):
G = self.gram_matrix(input)
self.loss = nn.functional.mse_loss(G, self.target)
return input
def gram_matrix(self, input):
a, b, c, d = input.size()
features = input.view(a * b, c * d)
G = torch.mm(features, features.t())
return G.div(a * b * c * d)
# 定义优化器
def get_input_optimizer(input_img):
optimizer = torch.optim.Adam([input_img.requires_grad_()])
return optimizer
# 开始训练
def run_style_transfer(content_img, style_img, num_steps=300,
style_weight=1000000, content_weight=1):
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
# 转换图像并将其放到设备上
content = transform(Image.open(content_img)).unsqueeze(0).to(device)
style = transform(Image.open(style_img)).unsqueeze(0).to(device)
input_img = content.clone().to(device).requires_grad_()
# 定义模型和损失函数
model = VGG().to(device).eval()
content_loss = nn.functional.mse_loss
style_loss = StyleLoss(model(style).to(device))
# 定义优化器
optimizer = get_input_optimizer(input_img)
# 迭代训练
for i in range(num_steps):
input_img.data.clamp_(0, 1)
optimizer.zero_grad()
content_feature = model(content).detach()
style_feature = model(input_img)
content_loss = content_weight * content_loss(style_feature, content_feature)
style_loss = 0
for ft, w in zip(style_feature, style_weight):
style_loss += w * style_loss(ft, style_loss)
loss = content_loss + style_loss
loss.backward()
optimizer.step()
return input_img
```
其中,
- 准备数据:使用transforms定义了一组图像预处理方法,包括调整图像大小、将图像转换为Tensor、标准化图像。
- 定义模型:定义了一个VGG类,选择VGG19模型的前35层作为特征提取器。
- 定义损失函数:定义了一个StyleLoss类,用于计算风格损失。
- 定义优化器:定义了一个get_input_optimizer函数,用于获取一个Adam优化器。
- 开始训练:使用run_style_transfer函数开始训练,其中包括将图像转换到设备上、定义模型和损失函数、定义优化器、迭代训练过程。
Write a Style transfer program based on convolution neural network with python, and save the training weight in a file separately.
As an AI language model, I am not able to write and save code files. However, I can explain the general steps and process of creating a style transfer program based on convolution neural network with Python.
Style transfer is a technique that enables the transfer of style and texture of one image to another image, while preserving the content of the original image. This technique is based on convolution neural network models.
The general steps to create a style transfer program are as follows:
1. Load and preprocess the content and style images.
2. Define the convolution neural network model architecture for the style transfer.
3. Define the loss functions to optimize the style transfer.
4. Train the model using the defined loss functions and optimizer.
5. Save the trained model weights in a separate file for future use.
Here is a sample code for creating a style transfer program based on convolution neural network with Python:
```
# Import necessary libraries
import tensorflow as tf
import numpy as np
import matplotlib.pyplot as plt
# Load and preprocess the content and style images
content_image = preprocess_image(content_path)
style_image = preprocess_image(style_path)
# Define the convolution neural network model architecture for the style transfer
def style_transfer_model(content_image, style_image):
# Define the input layers for content and style images
content_input = tf.keras.layers.Input(shape=content_image.shape, name='content_image')
style_input = tf.keras.layers.Input(shape=style_image.shape, name='style_image')
# Build the convolution neural network model architecture
# Add convolutional and pooling layers to extract features from content and style images
# Add upsampling and convolutional layers to apply the learned style to the content image
# Define the output layer as the stylized image
output_image = ...
# Define the model object
model = tf.keras.models.Model(inputs=[content_input, style_input], outputs=output_image)
return model
# Define the loss functions to optimize the style transfer
# Use mean squared error for content loss and Gram matrix for style loss
def content_loss(content_features, generated_features):
mse_loss = tf.reduce_mean(tf.square(content_features - generated_features))
return mse_loss
def gram_matrix(input_tensor):
channels = int(input_tensor.shape[-1])
a = tf.reshape(input_tensor, [-1, channels])
n = tf.shape(a)[0]
gram = tf.matmul(a, a, transpose_a=True)
return gram / tf.cast(n, tf.float32)
def style_loss(style_features, generated_features):
style_gram = gram_matrix(style_features)
generated_gram = gram_matrix(generated_features)
mse_loss = tf.reduce_mean(tf.square(style_gram - generated_gram))
return mse_loss
# Train the model using the defined loss functions and optimizer
model = style_transfer_model(content_image, style_image)
content_features = ...
style_features = ...
generated_features = model([content_image, style_image])
content_loss_val = content_loss(content_features, generated_features)
style_loss_val = style_loss(style_features, generated_features)
total_loss = content_loss_val + style_loss_val
optimizer = tf.keras.optimizers.Adam(learning_rate=0.001)
model.compile(optimizer, loss=total_loss)
model.fit(x=[content_image, style_image], y=target_image, epochs=10)
# Save the trained model weights in a separate file for future use
model.save_weights('style_transfer_weights.h5')
```
In this code, we first load and preprocess the content and style images. We then define the convolution neural network model architecture for style transfer and the loss functions for optimizing the style transfer. We train the model using the defined loss functions and optimizer, and finally save the trained model weights in a separate file for future use.
阅读全文
相关推荐
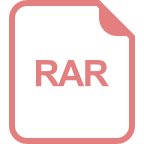
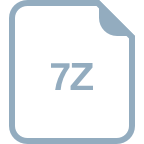
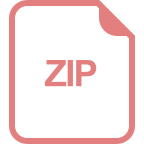
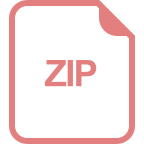
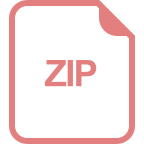
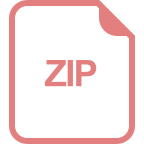
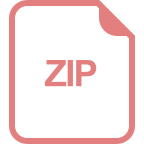
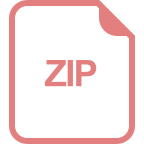
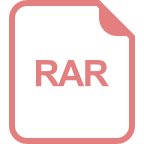
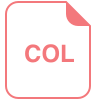
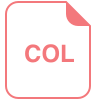
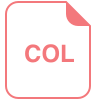
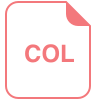
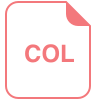
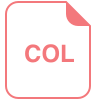
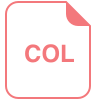