【Optimization Algorithms】: Tips for Enhancing GAN Stability: Creating More Robust Generative Models
发布时间: 2024-09-15 16:56:48 阅读量: 26 订阅数: 26 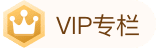
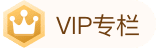
# 1. Introduction to Generative Adversarial Networks (GANs)
Generative Adversarial Networks (GANs), a groundbreaking technology in the field of deep learning, have achieved significant results in various areas, including image generation, text-to-image translation, data augmentation, and unsupervised learning. GAN consists of two key components: the Generator and the Discriminator. The Generator aims to produce data that is indistinguishable from real data, while the Discriminator's task is to differentiate between the fake data generated by the Generator and the real data. Ideally, when the GAN is trained well enough, the Generator can produce fake data that is indistinguishable from real data, and the Discriminator cannot tell the difference. This adversarial process drives the continuous improvement of the model. Understanding the basics of GANs is not only a prerequisite for in-depth study of its advanced features but also the key to solving stability issues and applying GAN technology in practice. The following chapters will provide detailed introductions to the internal structure of GANs, the challenges in the training process, and how to optimize these challenges.
# 2. Understanding Stability Issues in GANs
### 2.1 Basic Structure and Principles of GANs
#### 2.1.1 Roles of the Generator and Discriminator
Generative Adversarial Networks (GANs) consist of two main components: the Generator and the Discriminator. The Generator's task is to create fake data that is as close as possible to real data based on random noise. Meanwhile, the Discriminator's goal is to distinguish the data it receives as real or fake data generated by the Generator. This process can be compared to a game of cat and mouse between a police officer and a counterfeiter. The Generator becomes more adept at creating fake data, while the Discriminator becomes more skilled at telling the difference. When the performance of both reaches a balance, the quality of the data produced by the Generator is theoretically indistinguishable from real data.
#### 2.1.2 Loss Functions and Optimization Objectives
The training goal of GANs is to improve the performance of both the Generator and the Discriminator through the adversarial process. The Generator's loss function is typically the probability of the Discriminator making an incorrect judgment on the fake data, while the Discriminator's loss function is the negative log likelihood of its incorrect judgment on the data being real or fake. The training process involves minimizing these two loss functions through gradient descent. However, in practice, because the two loss functions are interdependent, this optimization process can easily become unstable, leading to issues such as mode collapse or training oscillation.
### 2.2 Common Problems in GAN Training
#### 2.2.1 Mode Collapse
Mode collapse is a common stability issue encountered during GAN training. It occurs when the Generator learns a few data patterns and continuously reproduces them, ignoring the existence of other patterns. This usually happens when a particular pattern is highly effective in the Discriminator's view, causing the Generator to over-rely on it. In such cases, although the Discriminator may be easily fooled, the diversity of the generated data is significantly reduced.
#### 2.2.2 Training Instability and Oscillation
Training instability and oscillation are characterized by the values of the model's loss functions fluctuating during the training process and not being able to settle at a lower level. This is usually related to incorrect choices of learning rates, gradient vanishing, or gradient explosion. Oscillation means that the GAN is constantly switching between multiple modes without converging to a stable state. The result is usually that the Generator cannot effectively learn the data distribution, and the quality of the generated data is poor.
#### 2.2.3 Gradient Vanishing and Explosion
Gradient vanishing and explosion are common problems when training deep neural networks, and GANs are no exception. When the gradient values become very small or very large, the weight updates for the Generator and Discriminator may become extremely slow (vanishing) or unstable (explosion). Gradient vanishing can cause the training to stagnate, while gradient explosion can cause model parameters to diverge to extreme values, making the model untrainable. To alleviate these issues, strategies such as gradient clipping, using more stable optimizers, and so on have been proposed and applied.
### 2.3 Stability Optimization Techniques in GANs
#### 2.3.1 Improved Gradient Update Strategies
One method to optimize the stability of GAN training is to introduce improved gradient update strategies. For example, adding momentum terms to accelerate the gradient descent process or using adaptive learning rate optimization algorithms like RMSprop and Adam to maintain training stability. In addition, some studies attempt to directly introduce constraints into the gradient update rules to prevent gradient vanishing or explosion problems.
#### 2.3.2 Data Augmentation and Regularization
Data augmentation techniques, widely used in other areas of deep learning, can also be applied to improve the stability of GAN training. By applying geometric and color transformations to the training data, the diversity of the training set can be increased, helping the Generator learn richer data patterns and reduce mode collapse. At the same time, adding regularization terms (such as L1/L2 regularization) can constrain the complexity of the model, prevent overfitting, and thus increase training stability.
```python
# Example code: Data augmentation example
from keras.preprocessing.image import ImageDataGenerator
# Create an ImageDataGenerator instance and configure data augmentation parameters
datagen = ImageDataGenerator(
rotation_range=30, # Randomly rotate images up to 30 degrees
width_shift_range=0.2, # Randomly shift images horizontally up to 20%
height_shift_range=0.2, # Randomly shift images vertically up to 20%
shear_range=0.2, # Randomly apply shearing transformations
zoom_range=0.2, # Randomly zoom in and out on images
horizontal_flip=True, # Randomly flip images horizontally
fill_mode='nearest' # Method to fill newly created pixels
)
# Use ImageDataGenerator for data augmentation
# Here we assume we have a DataFrame named train_data containing paths and labels for training images
# Assume train_generator is a custom generator function that generates augmented data based on train_data
datagen.flow_from_dataframe(
train_data, # DataFrame object
directory="path/to/train/directory", # Path to image directory
x_col='path', # Column name in DataFrame with image paths
y_col='label', # Column name in DataFrame with image labels
class_mode='binary', # Data class mode, binary
target_size=(150, 150), # Resize images
batch_size=32
)
```
In the above code, we configure a series of data augmentation parameters through the `ImageDataGenerator` class, such as rotation, translation, shearing, zooming, horizontal flipping, etc., and use the `flow_from_dataframe` method to generate augmented training data based on actual image paths and labels, enhancing the diversity of the training dataset.
# 3. GAN Stability Enhancement Strategies
## 3.1 Pattern Regularization Methods
### 3.1.1 Noise Injection
Noise injection is a technique used during GAN training to improve model stability. Injecting noise into the Generator's input can prevent the model from over-optimizing to specific samples, thus avoiding mode collapse. The noise can be random noise or Gaussian noise, depending on the specific task requirements. The amount of noise usually needs to be determined through experiments to balance between preventing mode collapse and maintaining the quality of generated samples.
Code examples and logical analysis:
```python
# Assume the model's input is Gaussian noise
import numpy as np
def generate_noise(batch_size, input_dim):
return np.random.normal(0, 1, (batch_size, input_dim))
# Inject noise into the Generator's forward propagation
def generator_forward(input_noise, generator_model):
# generator_model is the defined Generator model
generated_data = generator_model(input_noise)
return generated_data
# Assume we have a batch size of 64 and an input dimension of 100
batch_size = 64
input_dim = 100
noise = generate_noise(batch_size, input_dim)
# This is a simplified example of the Generator's forward propagation
generated_data = generator_forward(noise, generator_model)
```
In the above code, we first define a function `generate_noise` to generate noise, and then in the `generator_forward` function, we pass the noise as input to the Generator model. In practice, noise should be added to each layer or selectively added to certain layers.
Noise injection is a simple and effective technique, but controlling the amount of noise is key. If too much noise is added, it may lead to a decrease in the quality of the generated data; if too little, it may not effectively prevent mode collapse. Generally, experiments are needed to find a compromise solution.
### 3.1.2 Batch Normalization
Batch Normalization is another technique to improve model stability. It normalizes the input of each batch to address the problem of internal covariate shift, making the model less sensitive to the choice of learning rate and helping to alleviate mode collapse. Batch Normalization stabilizes the feature distribution by normalizing the mean and variance of each feature.
Code examples and logical analysis:
```python
from keras.layers import BatchNormalization
# Assuming this is a fully connected layer, we add Batch Normalization after this layer
from keras.layers import Dense
def batch_normalization_layer(input_tensor, num_units):
layer = Dense(num_units, activation=None)(input_tensor) # Linear fully connected layer
layer = BatchNormalization()(layer) # Batch Normalization layer
return layer
# Example of using a Batch Normalization layer
from keras.models import Model
from keras.layers import Input
input_tensor = Input(shape=(input_dim,))
output_tensor = batch_normalization_layer(input_tensor, num_units=100)
model = Model(inputs=input_tensor, outputs=output_tensor)
```
In the above code, we first create a fully connected layer, and then apply Batch Normalization after this layer. Thus, each time the network weights are updated, the input to this layer is normalized to ensure that its mean is close to 0 and its variance is close to 1. Batch Normalization can help the model converge faster, and when training GANs, it is usually placed in the hidden layers of the Generator.
Although Batch Normalization has many advantages, it can also cause some problems, such as gradient vanishing or gradient explosion. When using Batch Normalization, other techniques are usually used in combination, such as weight initialization strategies or learning rate adjustments, to achieve better training results.
## 3.2 Improvements in Loss Functions
### 3.2.1 Wasserstein Distance (WGAN)
The Wasserstein distance, also known as the Earth Mover's Distance (EMD), is proposed as a loss function in GANs to address the issues of training instability and mode c
0
0
相关推荐
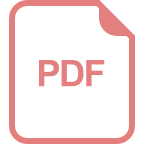
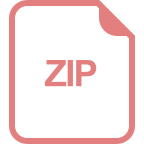
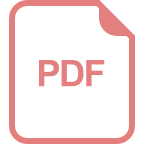
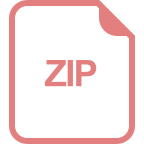
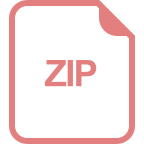
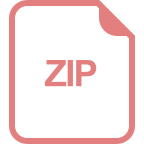
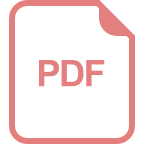
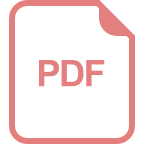
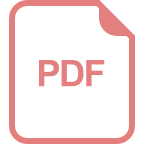