【Interdisciplinary Applications】: The Ethical Boundaries of GAN in Artistic Creation: Exploring the Integration of AI and Human Creativity
发布时间: 2024-09-15 16:51:54 阅读量: 29 订阅数: 33 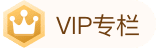
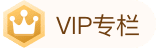
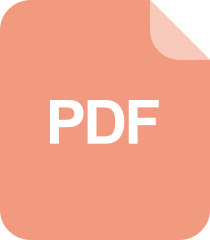
Analysis of the reliability and precision of spike timing by a single model neuron
# Introduction to Generative Adversarial Networks (GANs)
Generative Adversarial Networks (GANs) are a type of deep learning model composed of two parts: the generator and the discriminator. The generator is responsible for creating fake data that closely resembles real data, while the discriminator learns to distinguish between real data and the fake data produced by the generator. This adversarial training method is what gives GANs their name, and the core idea is to iteratively improve the quality of the data generated by the generator until it becomes difficult for the discriminator to differentiate between real and fake.
The strength of GANs lies in their powerful generative capabilities; they can learn the distribution of data in an unsupervised manner, automatically discovering key features in the data and creating new instances. This has shown tremendous potential in various fields, including image, video, music, and text generation.
However, the training process for GANs is complex and unstable, prone to issues such as mode collapse, which requires us to adopt appropriate techniques and optimization methods to overcome. For example, incorporating Wasserstein distance can improve training stability, while techniques like label smoothing and gradient penalty can prevent overfitting by the generator.
```
# Pseudocode example: A simple GAN structure
def generator(z):
# Map random noise z to the data space
return mapping_to_data_space(z)
def discriminator(X):
# Determine whether the input data is real or generated by the generator
return mapping_to_prob_space(X)
# Training process
for epoch in range(num_epochs):
for batch in data_loader:
# Train the discriminator
real_data, generated_data = get_real_and_generated_data(batch)
d_loss_real = loss_function(discriminator(real_data), 1)
d_loss_generated = loss_function(discriminator(generated_data), 0)
d_loss = d_loss_real + d_loss_generated
discriminator_optimizer.zero_grad()
d_loss.backward()
discriminator_optimizer.step()
# Train the generator
z = get_random_noise(batch_size)
generated_data = generator(z)
g_loss = loss_function(discriminator(generated_data), 1)
generator_optimizer.zero_grad()
g_loss.backward()
generator_optimizer.step()
```
With this foundational introduction, we can see how GANs stand out in the field of machine learning and open up new possibilities for AI in artistic creation. As research deepens and technology advances, the application scope of GANs is set to expand even further.
# GANs in Artistic Creation: Theoretical Aspects
## 2.1 Basic Principles and Architecture of GANs
### 2.1.1 Components of an Adversarial Network
Generative Adversarial Networks (GANs) consist of two parts: the generator and the discriminator. The generator's role is to create data; it takes a random noise vector and transforms it into fake data that closely resembles real data. The discriminator's task is to determine whether an image is real or fake, generated by the generator.
The relationship between the generator and discriminator is akin to that of a "counterfeiter" and a "policeman." The counterfeiter tries to mimic real currency as closely as possible to deceive the policeman, while the policeman endeavors to learn how to distinguish between counterfeit and real currency. The adversarial relationship between them drives the model's progress in learning.
**Parameter Explanation and Code Analysis:**
In Python, we can build GAN models using frameworks such as TensorFlow or PyTorch. Below is a simplified example of code for the generator and discriminator, along with pseudocode for the training process.
```python
import tensorflow as tf
from tensorflow.keras.layers import Dense, Conv2D, Flatten
# Generator model (simplified example)
def build_generator(z_dim):
model = tf.keras.Sequential()
# Input layer to hidden layer
model.add(Dense(128, activation='relu', input_dim=z_dim))
# Hidden layer to output layer, output image size is 64x64
model.add(Dense(64*64*1, activation='tanh'))
model.add(Reshape((64, 64, 1)))
return model
# Discriminator model (simplified example)
def build_discriminator(image_shape):
model = tf.keras.Sequential()
# Input layer, image size is 64x64
model.add(Flatten(input_shape=image_shape))
# Input layer to hidden layer
model.add(Dense(128, activation='relu'))
# Hidden layer to output layer, output decision result
model.add(Dense(1, activation='sigmoid'))
return model
```
In practical applications, more complex network structures and regularization techniques are needed to prevent model overfitting. For example, convolutional layers (Conv2D) can be used instead of fully connected layers (Dense) to adapt to the characteristics of image data.
### 2.1.2 GAN Training Process and Optimization Techniques
The GAN training process is a dynamic balancing act. If the discriminator improves too quickly, the generator will struggle to learn how to produce sufficiently realistic data; conversely, if the generator progresses too fast, the discriminator may become unable to distinguish between real and fake data. Therefore, when training GANs, it is necessary to finely tune learning rates and other hyperparameters.
**Optimization Techniques:**
1. **Learning Rate Decay:** Gradually decrease the learning rate as training progresses to allow the model to search more finely in the parameter space.
2. **Gradient Penalty (WGAN-GP):** Use gradient penalties to ensure that the data distribution generated by the model does not stray too far from the real data distribution.
3. **Batch Normalization:** Stabilize the training process and reduce the problem of vanishing gradients.
4. **Feature Matching:** Guide the generator's learning by comparing the feature statistics of real data with generated data.
**Code Example:**
```python
# GAN training pseudocode
# Define the loss function
def gan_loss(y_true, y_pred):
return tf.keras.losses.BinaryCrossentropy(from_logits=True)(y_true, y_pred)
# Define optimizers
g_optimizer = tf.keras.optimizers.Adam(learning_rate=0.0002, beta_1=0.5)
d_optimizer = tf.keras.optimizers.Adam(learning_rate=0.0002, beta_1=0.5)
# Training loop
for epoch in range(epochs):
for batch in data_loader:
# Train the discriminator
real_data = batch
fake_data = generator(tf.random.normal([batch_size, z_dim]))
with tf.GradientTape() as tape:
predictions_real = discriminator(real_data, training=True)
predictions_fake = discriminator(fake_data, training=True)
loss_real = gan_loss(tf.ones_like(predictions_real), predictions_real)
loss_fake = gan_loss(tf.zeros_like(predictions_fake), predictions_fake)
loss = (loss_real + loss_fake) / 2
gradients_of_discriminator = tape.gradient(loss, discriminator.trainable_variables)
d_optimizer.apply_gradients(zip(gradients_of_discriminator, discriminator.trainable_variables))
# Train the generator
with tf.GradientTape() as tape:
generated_data = generator(tf.random.normal([batch_size, z_dim]), training=True)
predictions = discriminator(generated_data, training=False)
gen_loss = gan_loss(tf.ones_like(predictions), predictions)
gradients_of_generator = tape.gradient(gen_loss, generator.trainable_variables)
g_optimizer.apply_gradients(zip(gradients_of_generator, generator.trainable_variables))
```
In practical applications, this code needs to be combined with specific library functions and models. Learning rate decay can typically be achieved using the optimizer's built-in decayers, while gradient penalties need to be added as additional terms in the loss function. Batch normalization is usually applied directly to each layer of the model, and feature matching requires collecting the statistical measures of real data during training, then training the generator to match these statistics with those of the generated data.
## 2.2 Artistic Expressions of GANs
### 2.2.1 Definition of Creativity and the Role of Human Artists
Creativity is at the heart of artistic creation; it refers to the ability to generate new ideas or things. In the field of artificial intelligence, creativity is often understood as the ability to recombine existing information in new or unique contexts.
In the application of GANs in art, the role of human artists is that of a guide and collaborator. They direct the model by setting initial parameters, designing network architectures, and training frameworks. At the same time, human artists can also post-process the generated results, adding personal creative elements.
### 2.2.2 Characteristics and Classification of GAN-Generated Art
GAN-generated artworks typically have the following characteristics:
- **Diversity:** GANs are capable of producing artworks in various styles and forms.
- **Rich in Detail:** With the appropriate dataset and model structure, GANs can create artworks with detailed content.
- **Novelty:** GANs are capable of creating unprecedented forms and styles of art.
The cla
0
0
相关推荐
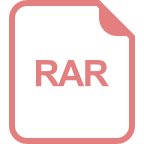
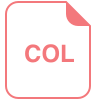
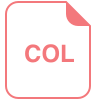
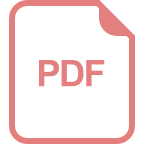
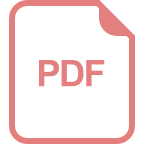
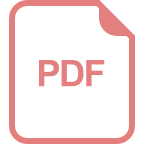
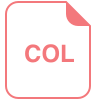
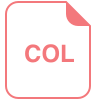
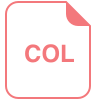