为我的nrf52832写一个事件调度驱动
时间: 2024-02-12 08:04:08 浏览: 33
好的,以下是一个简单的事件调度驱动的实现:
首先,我们需要定义一个事件结构体,用于存储事件的类型和处理函数:
```c
typedef struct {
uint8_t type;
void (*handler)();
} event_t;
```
其中,`type` 表示事件类型,`handler` 是一个指向事件处理函数的指针。
接着,我们需要定义一个事件队列,用于存储待处理的事件:
```c
#define MAX_EVENTS 16
static event_t event_queue[MAX_EVENTS];
static uint8_t event_head = 0;
static uint8_t event_tail = 0;
```
其中,`MAX_EVENTS` 表示事件队列的最大长度,`event_queue` 存储事件的数组,`event_head` 和 `event_tail` 分别表示队列的头和尾。
然后,我们需要实现两个函数,一个是用于向事件队列中添加事件的函数,另一个是用于从事件队列中取出并处理事件的函数。这两个函数可以分别命名为 `add_event()` 和 `dispatch_events()`。
`add_event()` 函数的实现如下:
```c
void add_event(uint8_t type, void (*handler)()) {
uint8_t next_tail = (event_tail + 1) % MAX_EVENTS;
if (next_tail == event_head) {
// Event queue is full
return;
}
event_queue[event_tail].type = type;
event_queue[event_tail].handler = handler;
event_tail = next_tail;
}
```
该函数接受两个参数,分别是事件类型和事件处理函数。它首先计算出下一个尾指针的位置,如果下一个尾指针的位置等于头指针的位置,说明队列已满,无法再添加新的事件,直接返回。否则,将事件类型和处理函数存储到下一个尾指针的位置,并将尾指针指向下一个位置。
`dispatch_events()` 函数的实现如下:
```c
void dispatch_events() {
while (event_head != event_tail) {
event_t event = event_queue[event_head];
event_head = (event_head + 1) % MAX_EVENTS;
event.handler();
}
}
```
该函数不接受任何参数,它通过循环遍历事件队列,将队列头指针指向下一个位置,并调用当前事件的处理函数。当头指针等于尾指针时,说明队列已经空了,函数结束。
最后,我们需要在主循环中调用 `dispatch_events()` 函数,以便及时处理事件:
```c
int main() {
// ...
while (1) {
// ...
dispatch_events();
}
return 0;
}
```
这样,我们就完成了一个简单的事件调度驱动的实现。
相关推荐
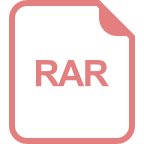
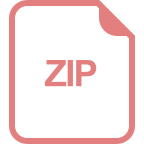














