编写完整贪吃蛇游戏,使用JAVA语言,用IntelliJ IDEA 软件编写
时间: 2024-01-16 19:03:46 浏览: 104
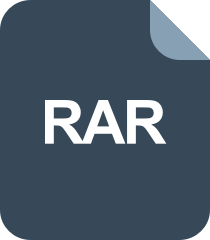
用JAVA语言开发贪吃蛇游戏
好的,我可以为您提供一个基于Java语言和IntelliJ IDEA软件的简单贪吃蛇游戏实现。下面是一个基本的实现步骤:
1. 创建一个新的Java项目,命名为SnakeGame。
2. 在src目录下创建一个名为“snake”的包,用于存放所有的Java类。
3. 创建一个名为“Snake”的Java类,该类表示贪吃蛇本身,包括其当前位置、方向和长度等属性,以及其移动、生长等行为。
```java
package snake;
public class Snake {
private int length; // 贪吃蛇长度
private int[] x; // 贪吃蛇每一节的横坐标
private int[] y; // 贪吃蛇每一节的纵坐标
private int direction; // 贪吃蛇移动的方向
public Snake(int length) {
this.length = length;
x = new int[length];
y = new int[length];
for (int i = 0; i < length; i++) {
x[i] = 0;
y[i] = i;
}
direction = 1;
}
public void move() {
for (int i = length - 1; i > 0; i--) {
x[i] = x[i - 1];
y[i] = y[i - 1];
}
switch (direction) {
case 0:
y[0]--;
break;
case 1:
x[0]++;
break;
case 2:
y[0]++;
break;
case 3:
x[0]--;
break;
}
}
public void grow() {
length++;
int[] newx = new int[length];
int[] newy = new int[length];
for (int i = 0; i < length - 1; i++) {
newx[i] = x[i];
newy[i] = y[i];
}
newx[length - 1] = x[length - 2];
newy[length - 1] = y[length - 2];
x = newx;
y = newy;
}
public boolean hitWall(int width, int height) {
if (x[0] < 0 || x[0] >= width || y[0] < 0 || y[0] >= height) {
return true;
}
return false;
}
public boolean hitBody() {
for (int i = 1; i < length; i++) {
if (x[0] == x[i] && y[0] == y[i]) {
return true;
}
}
return false;
}
public void setDirection(int direction) {
this.direction = direction;
}
public int getLength() {
return length;
}
public int getX(int index) {
return x[index];
}
public int getY(int index) {
return y[index];
}
public int getDirection() {
return direction;
}
}
```
4. 创建一个名为“Food”的Java类,该类表示食物,包括其位置和生成位置等属性、以及其随机生成的行为。
```java
package snake;
import java.util.Random;
public class Food {
private int x; // 食物的横坐标
private int y; // 食物的纵坐标
public Food(int width, int height) {
generate(width, height);
}
public void generate(int width, int height) {
Random rand = new Random();
x = rand.nextInt(width - 1);
y = rand.nextInt(height - 1);
}
public int getX() {
return x;
}
public int getY() {
return y;
}
}
```
5. 创建一个名为“GamePanel”的Java类,该类表示整个游戏界面,包括其大小、贪吃蛇和食物的显示、以及键盘事件的监听等行为。
```java
package snake;
import javax.swing.*;
import java.awt.*;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
public class GamePanel extends JPanel implements KeyListener {
private Snake snake;
private Food food;
private int width;
private int height;
private boolean gameover;
public GamePanel(int width, int height) {
this.width = width;
this.height = height;
snake = new Snake(3);
food = new Food(width, height);
gameover = false;
setPreferredSize(new Dimension(width * 10, height * 10));
setBackground(Color.WHITE);
setFocusable(true);
addKeyListener(this);
new Thread(new Runnable() {
@Override
public void run() {
while (!gameover) {
snake.move();
if (snake.getX(0) == food.getX() && snake.getY(0) == food.getY()) {
snake.grow();
food.generate(width, height);
}
if (snake.hitWall(width, height) || snake.hitBody()) {
gameover = true;
}
repaint();
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
JOptionPane.showMessageDialog(null, "Game Over!");
System.exit(0);
}
}).start();
}
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
g.setColor(Color.RED);
g.fillRect(food.getX() * 10, food.getY() * 10, 10, 10);
g.setColor(Color.BLACK);
for (int i = 0; i < snake.getLength(); i++) {
g.fillRect(snake.getX(i) * 10, snake.getY(i) * 10, 10, 10);
}
}
@Override
public void keyPressed(KeyEvent e) {
switch (e.getKeyCode()) {
case KeyEvent.VK_UP:
if (snake.getDirection() != 2) {
snake.setDirection(0);
}
break;
case KeyEvent.VK_RIGHT:
if (snake.getDirection() != 3) {
snake.setDirection(1);
}
break;
case KeyEvent.VK_DOWN:
if (snake.getDirection() != 0) {
snake.setDirection(2);
}
break;
case KeyEvent.VK_LEFT:
if (snake.getDirection() != 1) {
snake.setDirection(3);
}
break;
}
}
@Override
public void keyReleased(KeyEvent e) {
}
@Override
public void keyTyped(KeyEvent e) {
}
}
```
6. 创建一个名为“SnakeGame”的Java类,该类表示整个游戏的入口和启动。
```java
package snake;
import javax.swing.*;
public class SnakeGame {
public static void main(String[] args) {
int width = 40;
int height = 30;
JFrame frame = new JFrame("Snake Game");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new GamePanel(width, height));
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
}
```
7. 运行SnakeGame类,即可启动贪吃蛇游戏。
这份代码只是一个简单的实现,您可以根据自己的需求进行修改和完善。
阅读全文
相关推荐







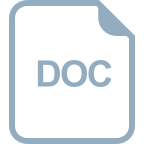
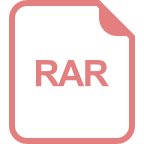
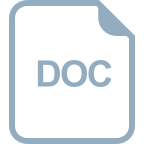


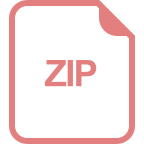
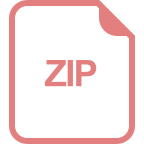
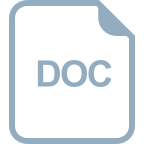
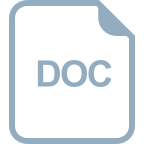