用Java语言编写贪吃蛇,用IntelliJ IDEA 软件编写,需要有开始和结束按钮
时间: 2024-01-22 16:21:13 浏览: 135
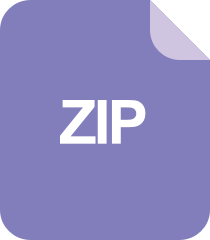
用JAVA编写的贪吃蛇小游戏 实现了开始START界面,结束GAME OVER界面,.zip
好的,下面是一个简单的Java贪吃蛇游戏实现,包含开始和结束按钮。我使用Java Swing库来实现界面,使用Java线程来控制蛇的移动和更新游戏界面。
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.ArrayList;
import java.util.Random;
public class SnakeGame extends JFrame implements ActionListener, KeyListener {
private final int WIDTH = 500; // 游戏窗口宽度
private final int HEIGHT = 500; // 游戏窗口高度
private final int CELL_SIZE = 20; // 蛇身和食物大小
private final int DELAY = 100; // 蛇移动延迟,单位: 毫秒
private final Random random = new Random(); // 生成随机数
private Timer timer; // 游戏计时器
private boolean isRunning = false; // 游戏是否正在运行
private boolean isGameOver = false; // 游戏是否结束
private int score = 0; // 得分
private Snake snake; // 蛇
private Food food; // 食物
private JButton startButton; // 开始按钮
private JButton endButton; // 结束按钮
private JLabel scoreLabel; // 显示得分的标签
public SnakeGame() {
setTitle("Java贪吃蛇游戏");
setSize(WIDTH, HEIGHT);
setLocationRelativeTo(null); // 窗口居中
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setResizable(false); // 禁止调整窗口大小
setLayout(null); // 使用绝对布局
// 添加开始和结束按钮
startButton = new JButton("开始");
startButton.setBounds(180, 400, 80, 30);
startButton.addActionListener(this);
add(startButton);
endButton = new JButton("结束");
endButton.setBounds(240, 400, 80, 30);
endButton.addActionListener(this);
add(endButton);
// 添加得分标签
scoreLabel = new JLabel("得分: " + score);
scoreLabel.setBounds(20, 20, 100, 30);
add(scoreLabel);
// 注册键盘监听器
addKeyListener(this);
setVisible(true);
}
// 开始游戏
private void startGame() {
isRunning = true;
isGameOver = false;
score = 0;
scoreLabel.setText("得分: " + score);
// 初始化蛇和食物
snake = new Snake(WIDTH / 2, HEIGHT / 2, CELL_SIZE);
food = new Food(random.nextInt(WIDTH / CELL_SIZE) * CELL_SIZE,
random.nextInt(HEIGHT / CELL_SIZE) * CELL_SIZE, CELL_SIZE);
// 启动计时器
timer = new Timer(DELAY, this);
timer.start();
}
// 结束游戏
private void endGame() {
isRunning = false;
isGameOver = true;
timer.stop();
JOptionPane.showMessageDialog(this, "游戏结束,得分为:" + score);
}
// 更新游戏界面
private void updateGame() {
// 更新蛇的位置
if (snake.move(food)) {
// 如果蛇吃到了食物,则生成新的食物
food = new Food(random.nextInt(WIDTH / CELL_SIZE) * CELL_SIZE,
random.nextInt(HEIGHT / CELL_SIZE) * CELL_SIZE, CELL_SIZE);
score += 10;
scoreLabel.setText("得分: " + score);
}
// 检查是否撞到墙或自己
if (snake.isDead(WIDTH, HEIGHT)) {
endGame();
}
// 更新游戏界面
repaint();
}
// 绘制游戏界面
@Override
public void paint(Graphics g) {
super.paint(g);
// 绘制蛇和食物
snake.draw(g);
food.draw(g);
}
// 监听按钮事件和计时器事件
@Override
public void actionPerformed(ActionEvent e) {
Object source = e.getSource();
if (source == startButton) {
if (!isRunning && isGameOver) {
startGame();
}
} else if (source == endButton) {
if (isRunning) {
endGame();
}
} else if (source == timer) {
if (isRunning) {
updateGame();
}
}
}
// 监听键盘事件
@Override
public void keyPressed(KeyEvent e) {
if (isRunning) {
int keyCode = e.getKeyCode();
switch (keyCode) {
case KeyEvent.VK_UP:
snake.setDirection(Snake.Direction.UP);
break;
case KeyEvent.VK_DOWN:
snake.setDirection(Snake.Direction.DOWN);
break;
case KeyEvent.VK_LEFT:
snake.setDirection(Snake.Direction.LEFT);
break;
case KeyEvent.VK_RIGHT:
snake.setDirection(Snake.Direction.RIGHT);
break;
}
}
}
@Override
public void keyTyped(KeyEvent e) {}
@Override
public void keyReleased(KeyEvent e) {}
// 蛇类
private static class Snake {
private ArrayList<Point> body; // 蛇身
private int cellSize; // 蛇身大小
private Direction direction; // 移动方向
public Snake(int x, int y, int cellSize) {
this.body = new ArrayList<>();
this.body.add(new Point(x, y));
this.cellSize = cellSize;
this.direction = Direction.RIGHT;
}
// 设置移动方向
public void setDirection(Direction direction) {
if (this.direction == Direction.UP && direction == Direction.DOWN ||
this.direction == Direction.DOWN && direction == Direction.UP ||
this.direction == Direction.LEFT && direction == Direction.RIGHT ||
this.direction == Direction.RIGHT && direction == Direction.LEFT) {
return;
}
this.direction = direction;
}
// 移动蛇
public boolean move(Food food) {
Point head = body.get(0);
int x = head.x;
int y = head.y;
switch (direction) {
case UP:
y -= cellSize;
break;
case DOWN:
y += cellSize;
break;
case LEFT:
x -= cellSize;
break;
case RIGHT:
x += cellSize;
break;
}
Point newHead = new Point(x, y);
// 检查是否吃到食物
if (newHead.equals(food.getPosition())) {
body.add(0, newHead);
return true;
}
// 移动蛇身
for (int i = body.size() - 1; i > 0; i--) {
body.set(i, body.get(i - 1));
}
body.set(0, newHead);
return false;
}
// 检查是否撞到墙或自己
public boolean isDead(int width, int height) {
Point head = body.get(0);
if (head.x < 0 || head.x >= width || head.y < 0 || head.y >= height) {
return true;
}
for (int i = 1; i < body.size(); i++) {
if (head.equals(body.get(i))) {
return true;
}
}
return false;
}
// 绘制蛇
public void draw(Graphics g) {
g.setColor(Color.GREEN);
for (Point point : body) {
g.fillRect(point.x, point.y, cellSize, cellSize);
}
}
// 移动方向枚举类
public enum Direction {
UP, DOWN, LEFT, RIGHT
}
}
// 食物类
private static class Food {
private Point position; // 食物位置
private int cellSize; // 食物大小
public Food(int x, int y, int cellSize) {
this.position = new Point(x, y);
this.cellSize = cellSize;
}
// 获取食物位置
public Point getPosition() {
return position;
}
// 绘制食物
public void draw(Graphics g) {
g.setColor(Color.RED);
g.fillOval(position.x, position.y, cellSize, cellSize);
}
}
public static void main(String[] args) {
new SnakeGame();
}
}
```
阅读全文
相关推荐

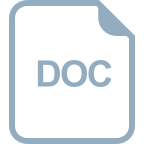
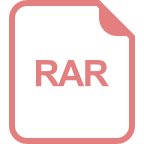
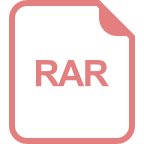
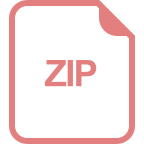
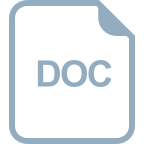
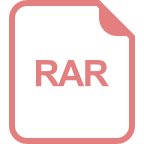
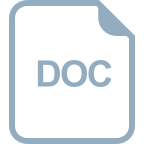
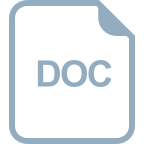
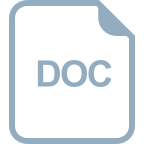
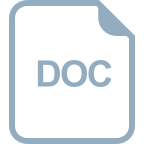
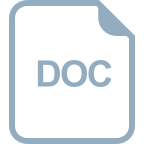
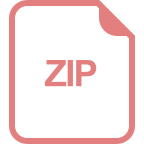
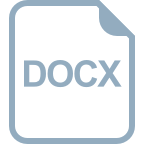
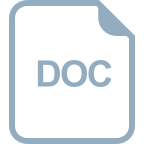
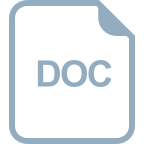