编写贪吃蛇小游戏,使用JAVA语言,用IntelliJ IDEA软件编写
时间: 2023-06-27 09:08:17 浏览: 59
好的,以下是一个简单的贪吃蛇小游戏的Java代码,你可以在IntelliJ IDEA中创建一个Java项目,将代码复制到Main.java文件中,然后运行该文件来启动游戏。
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.util.Random;
public class Main extends JPanel implements ActionListener {
private static final int SCREEN_WIDTH = 600;
private static final int SCREEN_HEIGHT = 600;
private static final int UNIT_SIZE = 25;
private static final int GAME_UNITS = (SCREEN_WIDTH * SCREEN_HEIGHT) / UNIT_SIZE;
private static final int DELAY = 75;
private final int[] x = new int[GAME_UNITS];
private final int[] y = new int[GAME_UNITS];
private int bodyParts = 6;
private int applesEaten;
private int appleX;
private int appleY;
private char direction = 'R';
private boolean running = false;
private Timer timer;
private Random random;
public Main() {
random = new Random();
setPreferredSize(new Dimension(SCREEN_WIDTH, SCREEN_HEIGHT));
setBackground(Color.BLACK);
setFocusable(true);
addKeyListener(new MyKeyAdapter());
startGame();
}
private void startGame() {
newApple();
running = true;
timer = new Timer(DELAY, this);
timer.start();
}
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
draw(g);
}
private void draw(Graphics g) {
if (running) {
g.setColor(Color.RED);
g.fillOval(appleX, appleY, UNIT_SIZE, UNIT_SIZE);
for (int i = 0; i < bodyParts; i++) {
if (i == 0) {
g.setColor(Color.GREEN);
} else {
g.setColor(new Color(45, 180, 0));
}
g.fillRect(x[i], y[i], UNIT_SIZE, UNIT_SIZE);
}
g.setColor(Color.RED);
g.setFont(new Font("Ink Free", Font.BOLD, 40));
FontMetrics metrics = getFontMetrics(g.getFont());
g.drawString("Score: " + applesEaten, (SCREEN_WIDTH - metrics.stringWidth("Score: " + applesEaten)) / 2, g.getFont().getSize());
} else {
gameOver(g);
}
}
private void newApple() {
appleX = random.nextInt((int) (SCREEN_WIDTH / UNIT_SIZE)) * UNIT_SIZE;
appleY = random.nextInt((int) (SCREEN_HEIGHT / UNIT_SIZE)) * UNIT_SIZE;
}
private void move() {
for (int i = bodyParts; i > 0; i--) {
x[i] = x[i - 1];
y[i] = y[i - 1];
}
switch (direction) {
case 'U':
y[0] = y[0] - UNIT_SIZE;
break;
case 'D':
y[0] = y[0] + UNIT_SIZE;
break;
case 'L':
x[0] = x[0] - UNIT_SIZE;
break;
case 'R':
x[0] = x[0] + UNIT_SIZE;
break;
}
}
private void checkApple() {
if ((x[0] == appleX) && (y[0] == appleY)) {
bodyParts++;
applesEaten++;
newApple();
}
}
private void checkCollisions() {
for (int i = bodyParts; i > 0; i--) {
if ((x[0] == x[i]) && (y[0] == y[i])) {
running = false;
}
}
if (x[0] < 0) {
running = false;
}
if (x[0] > SCREEN_WIDTH) {
running = false;
}
if (y[0] < 0) {
running = false;
}
if (y[0] > SCREEN_HEIGHT) {
running = false;
}
if (!running) {
timer.stop();
}
}
private void gameOver(Graphics g) {
g.setColor(Color.RED);
g.setFont(new Font("Ink Free", Font.BOLD, 75));
FontMetrics metrics = getFontMetrics(g.getFont());
g.drawString("Game Over", (SCREEN_WIDTH - metrics.stringWidth("Game Over")) / 2, SCREEN_HEIGHT / 2);
g.setColor(Color.RED);
g.setFont(new Font("Ink Free", Font.BOLD, 40));
metrics = getFontMetrics(g.getFont());
g.drawString("Score: " + applesEaten, (SCREEN_WIDTH - metrics.stringWidth("Score: " + applesEaten)) / 2, g.getFont().getSize());
}
@Override
public void actionPerformed(ActionEvent e) {
if (running) {
move();
checkApple();
checkCollisions();
}
repaint();
}
private class MyKeyAdapter extends KeyAdapter {
@Override
public void keyPressed(KeyEvent e) {
switch (e.getKeyCode()) {
case KeyEvent.VK_LEFT:
if (direction != 'R') {
direction = 'L';
}
break;
case KeyEvent.VK_RIGHT:
if (direction != 'L') {
direction = 'R';
}
break;
case KeyEvent.VK_UP:
if (direction != 'D') {
direction = 'U';
}
break;
case KeyEvent.VK_DOWN:
if (direction != 'U') {
direction = 'D';
}
break;
}
}
}
public static void main(String[] args) {
JFrame frame = new JFrame("Snake");
frame.setContentPane(new Main());
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
}
```
这段代码使用Java Swing库来创建游戏窗口,并使用键盘监听器来处理用户输入。该游戏使用一个定时器来不断更新贪吃蛇的位置,检查是否吃到苹果或者撞到边界或自己身体,最后在游戏结束时显示得分和Game Over的信息。
相关推荐
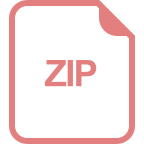
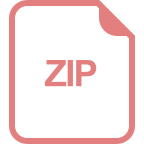
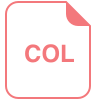
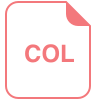
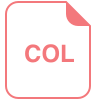
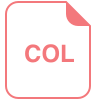
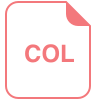









