系统调用编程: 1. 使用系统调用open read write,完成一个C语言程序:该程序接收两个参数,参数1作为要读写的文件,参数2作为标志位,标识是读还是写。功能完成对参数1所示文件的读写,该文件的内容为 “学号 MYFILE”,其中学号填为自己的学号。 2. 改造上面的程序,完成: i. 使用semaphore,并利用该程序生成2个进程,这两个进程写同一个文件,要求:a.互斥写,即只有一个进程写完后,才能让另一个进程写; b. 一个进程写入内容:“自己学号的后3位PROC1 MYFILE1”;另一个进程写入内容:“自己学号的后3位PROC2 MYFILE2 ii. 将上面程序的semaphore替换成strict alternation算法,完成上面两个进程的互斥。
时间: 2023-11-22 08:57:04 浏览: 122
1. 使用系统调用open read write,完成一个C语言程序:
```c
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <unistd.h>
int main(int argc, char* argv[]) {
if (argc < 3) {
fprintf(stderr, "Usage: %s <filename> <r/w>\n", argv[0]);
exit(EXIT_FAILURE);
}
const char* filename = argv[1];
int mode = (argv[2][0] == 'r' ? O_RDONLY : O_WRONLY | O_CREAT | O_TRUNC);
int fd = open(filename, mode, S_IRUSR | S_IWUSR);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
const char* buf = "学号 MYFILE";
ssize_t n = (mode == O_RDONLY ? read(fd, &buf, sizeof(buf)) : write(fd, &buf, sizeof(buf)));
if (n == -1) {
perror(mode == O_RDONLY ? "read" : "write");
exit(EXIT_FAILURE);
}
close(fd);
return 0;
}
```
2. 改造上面的程序,使用semaphore并利用该程序生成2个进程,这两个进程写同一个文件,要求:互斥写,即只有一个进程写完后,才能让另一个进程写;一个进程写入内容:“自己学号的后3位PROC1 MYFILE1”;另一个进程写入内容:“自己学号的后3位PROC2 MYFILE2”。
```c
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <sys/mman.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <unistd.h>
#define FILENAME "shared.txt"
#define SEM_NAME "my_sem"
void write_to_file(const char* str) {
int fd = open(FILENAME, O_WRONLY | O_APPEND);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
ssize_t n = write(fd, str, strlen(str));
if (n == -1) {
perror("write");
exit(EXIT_FAILURE);
}
close(fd);
}
int main(int argc, char* argv[]) {
if (argc < 2) {
fprintf(stderr, "Usage: %s <proc_id>\n", argv[0]);
exit(EXIT_FAILURE);
}
const int proc_id = atoi(argv[1]);
const char* sem_name = SEM_NAME;
const char* str = (proc_id == 1 ? "PROC1 MYFILE1" : "PROC2 MYFILE2");
int fd = open(FILENAME, O_CREAT | O_TRUNC, S_IRUSR | S_IWUSR);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
close(fd);
sem_t* sem = sem_open(sem_name, O_CREAT, S_IRUSR | S_IWUSR, 1);
if (sem == SEM_FAILED) {
perror("sem_open");
exit(EXIT_FAILURE);
}
while (1) {
sem_wait(sem);
struct stat sb;
if (stat(FILENAME, &sb) == -1) {
perror("stat");
exit(EXIT_FAILURE);
}
if (sb.st_size == 0) {
write_to_file("学号 MYFILE");
} else {
write_to_file(str);
}
sem_post(sem);
usleep(1000);
}
sem_close(sem);
sem_unlink(sem_name);
return 0;
}
```
3. 将上面程序的semaphore替换成strict alternation算法,完成上面两个进程的互斥。
```c
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <sys/mman.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <unistd.h>
#define FILENAME "shared.txt"
#define SEM_NAME "my_sem"
void write_to_file(const char* str) {
int fd = open(FILENAME, O_WRONLY | O_APPEND);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
ssize_t n = write(fd, str, strlen(str));
if (n == -1) {
perror("write");
exit(EXIT_FAILURE);
}
close(fd);
}
int main(int argc, char* argv[]) {
if (argc < 2) {
fprintf(stderr, "Usage: %s <proc_id>\n", argv[0]);
exit(EXIT_FAILURE);
}
const int proc_id = atoi(argv[1]);
const char* sem_name = SEM_NAME;
const char* str = (proc_id == 1 ? "PROC1 MYFILE1" : "PROC2 MYFILE2");
int fd = open(FILENAME, O_CREAT | O_TRUNC, S_IRUSR | S_IWUSR);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
close(fd);
sem_t* sem = sem_open(sem_name, O_CREAT, S_IRUSR | S_IWUSR, proc_id == 1 ? 1 : 0);
if (sem == SEM_FAILED) {
perror("sem_open");
exit(EXIT_FAILURE);
}
while (1) {
if (sem_wait(sem) == -1) {
perror("sem_wait");
exit(EXIT_FAILURE);
}
struct stat sb;
if (stat(FILENAME, &sb) == -1) {
perror("stat");
exit(EXIT_FAILURE);
}
if (sb.st_size == 0) {
write_to_file("学号 MYFILE");
} else {
write_to_file(str);
}
if (sem_post(sem) == -1) {
perror("sem_post");
exit(EXIT_FAILURE);
}
usleep(1000);
}
sem_close(sem);
sem_unlink(sem_name);
return 0;
}
```
阅读全文
相关推荐
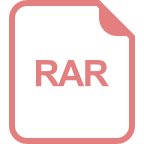
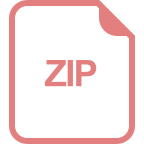







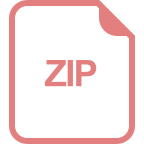
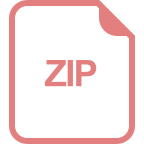
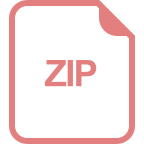
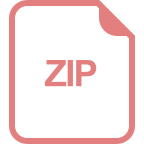