使用系统调用open read write,完成一个C语言程序:该程序接收两个参数,参数1作为要读写的文件,参数2作为标志位,标识是读还是写。功能完成对参数1所示文件的读写,该文件的内容为 “21009201267MYFILE”.
时间: 2024-02-03 22:13:33 浏览: 156
以下是一个简单的实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
int main(int argc, char *argv[]) {
if (argc != 3) {
printf("Usage: %s <filename> <r/w>\n", argv[0]);
exit(1);
}
char *filename = argv[1];
char mode = argv[2][0];
int fd = open(filename, mode == 'r' ? O_RDONLY : O_WRONLY | O_TRUNC | O_CREAT, 0644);
if (fd == -1) {
perror("open");
exit(1);
}
char buf[256];
int n;
if (mode == 'r') {
n = read(fd, buf, sizeof(buf));
write(STDOUT_FILENO, buf, n);
} else if (mode == 'w') {
n = write(fd, "21009201267MYFILE", 16);
printf("%d bytes written\n", n);
} else {
printf("Invalid mode: %c\n", mode);
exit(1);
}
close(fd);
return 0;
}
```
该程序接受两个参数,第一个参数为文件名,第二个参数为'r'或'w',标识读或写操作。程序根据标志位打开文件,并进行相应的读写操作。如果参数不正确或文件打开失败,程序会输出错误信息并退出。
阅读全文
相关推荐







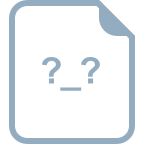
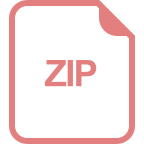
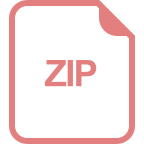
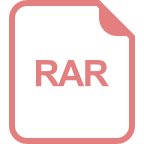
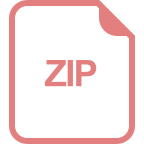
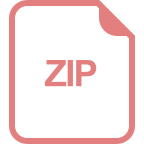