在Python中,能否提供一个详细的步骤和代码示例来展示如何使用A*算法解决最短路径问题?
时间: 2024-11-17 08:30:26 浏览: 12
当然可以。A*(A-star)算法是一种启发式搜索算法,常用于寻找两点之间的最短路径。在Python中,我们可以使用`heapq`库来实现优先队列,它是A*算法的关键部分。以下是使用Python实现A*算法的一个简化的步骤和代码示例:
首先,假设我们有一个图表示为邻接矩阵(Adjacency Matrix),其中值代表从一个节点到另一个节点的距离或代价。我们将使用`heapq`来存储待探索的节点,并使用F值(F = G + H)作为比较依据,其中G是已知的直接距离,H是估算的启发式距离。
1. 定义图结构和数据结构:
```python
import heapq
def heuristic(a, b):
# 这里是计算两个节点之间距离或代价的启发式函数,例如曼哈顿距离或欧几里得距离
return abs(a[0] - b[0]) + abs(a[1] - b[1])
class Node:
def __init__(self, pos, g=0, h=0, f=0):
self.pos = pos
self.g = g
self.h = h
self.f = f
```
2. A*算法核心部分:
```python
def a_star_search(graph, start, goal):
open_list = []
heapq.heappush(open_list, (start.f, start))
came_from = {}
cost_so_far = {start: 0}
while open_list:
current = heapq.heappop(open_list)[1]
if current == goal:
break
for neighbor in graph[current]:
tentative_g_score = cost_so_far[current] + graph[current][neighbor]
if neighbor not in cost_so_far or tentative_g_score < cost_so_far[neighbor]:
cost_so_far[neighbor] = tentative_g_score
priority = tentative_g_score + heuristic(neighbor, goal)
neighbor_node = Node(neighbor, g=tentative_g_score, h=priority - tentative_g_score, f=priority)
heapq.heappush(open_list, (priority, neighbor_node))
came_from[neighbor_node] = current
path = [goal]
while goal in came_from:
goal = came_from[goal]
path.append(goal)
return path[::-1], cost_so_far[goal]
```
3. 使用邻接矩阵构建图并测试:
```python
# 假设这是一个5x5的二维网格,每个元素为可达节点的成本
grid = [[0, 1, 0, 0, 9],
[8, 0, 0, 0, 0],
[0, 0, 0, 0, 10],
[0, 0, 0, 0, 3],
[5, 0, 9, 11, 0]]
start = Node((0, 0))
goal = Node((4, 4))
path, distance = a_star_search(grid, start, goal)
print(f"Shortest Path: {path}, Distance from Start to Goal: {distance}")
```
这个例子展示了如何在给定的图上使用A*算法找到从起点到终点的最短路径。注意,实际应用中,你可能需要根据实际情况调整启发式函数`heuristic()`,以便更准确地估计路径成本。
阅读全文
相关推荐
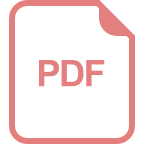
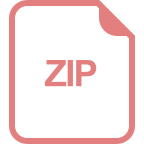
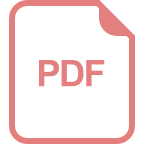









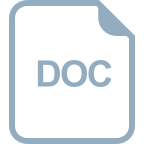





