如何在Python中详细地实现广度优先搜索(BFS)算法来解决最短路径查找问题?
时间: 2024-11-20 13:38:50 浏览: 33
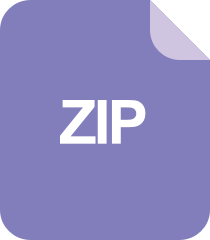
双向广度优先搜索(BBFS)路径规划算法(Python实现)
在Python中,广度优先搜索(BFS,Breadth-First Search)是一种用于寻找图中两点之间最短路径的经典算法。以下是通过列表(队列)实现BFS的基本步骤:
1. **初始化**:创建一个空的队列,用于存储待访问的节点;将起点加入队列。
```python
from collections import deque
def bfs(graph, start):
queue = deque([start])
```
2. **循环直到队列为空**:对于队列中的每个节点,执行以下操作:
a. 提取队首元素。
b. 如果该节点是终点,返回找到的路径。
c. 将当前节点的所有未访问邻居加入队列,并标记它们为已访问。
```python
while queue:
node = queue.popleft()
if node == 'end': # 假设'end'代表目标节点
return [node] # 返回从起点到终点的路径,只包含目标节点
for neighbor in graph[node]: # 图的邻接列表表示法,这里的graph[node]是当前节点的所有邻居
if neighbor not in visited: # visited用于跟踪已访问的节点,防止重复访问
visited.add(neighbor) # 标记邻居为已访问
queue.append(neighbor)
```
3. **无解情况**:如果遍历完所有节点都没有到达终点,说明找不到最短路径,可以返回None 或者抛出异常。
完整代码示例:
```python
visited = set()
def bfs_shortest_path(graph, start, end):
queue = deque([start])
visited.add(start)
while queue:
node = queue.popleft()
if node == end:
return [node]
for neighbor in graph[node]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
return None # 无解,表示无法从start到达end
# 使用示例:
graph = {'A': ['B', 'C'],
'B': ['D', 'E'],
'C': ['F'],
'D': [],
'E': ['F'],
'F': []}
print(bfs_shortest_path(graph, 'A', 'F')) # 输出:['A', 'C', 'F']
```
阅读全文
相关推荐
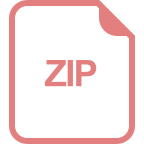
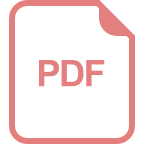
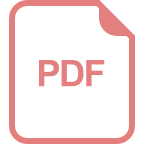
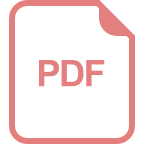
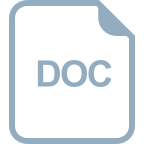
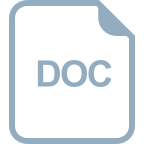
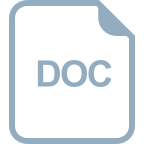



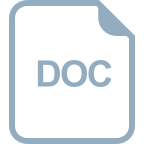
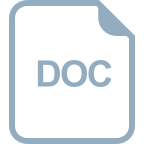




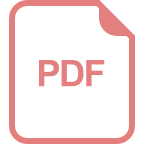
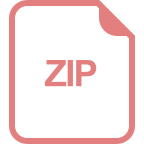