matlab能量熵代码
时间: 2023-05-26 17:01:11 浏览: 928
以下是MATLAB计算信号的能量和熵的示例代码:
%假设信号为x
x = randn(1, 1000); %生成1000个随机数的信号
%计算信号的能量
energy = sum(x.^2);
%计算信号的熵
histogram = hist(x, 100); %将信号分成100个bin并计算每个bin的频率
histogram = histogram / sum(histogram); %对频率进行归一化
entropy = -sum(histogram .* log2(histogram + eps)); %使用熵的公式计算熵值
%显示结果
fprintf('信号的能量为:%.2f\n', energy);
fprintf('信号的熵为:%.2f\n', entropy);
相关问题
matlab 能量熵
在MATLAB中,能量熵是一种用于信号处理和图像处理的特征描述方法。它可以用来衡量信号或图像的复杂性和信息量。
能量熵结合了信号的能量和熵两个方面的信息。能量是信号的幅度的平方和,表示信号的强度或者能量分布情况。熵是信号的不确定性或者信息量的度量,表示信号的复杂性或者随机性。
在MATLAB中,计算能量熵可以使用以下步骤:
1. 首先,计算信号的能量。可以使用`sum`函数计算信号的幅度的平方和。
2. 然后,计算信号的概率密度函数。可以使用`histogram`函数计算信号的直方图,并将直方图归一化得到概率密度函数。
3. 最后,计算信号的熵。可以使用`entropy`函数计算信号的熵。
以下是MATLAB代码示例,用于计算信号的能量熵:
```matlab
% 生成示例信号
signal = randn(1, 1000);
% 计算信号的能量
energy = sum(signal.^2);
% 计算信号的概率密度函数
histogram = histogram(signal, 'Normalization', 'probability');
pdf = histogram.Values;
% 计算信号的熵
entropy = -sum(pdf .* log2(pdf));
% 显示结果
disp(['能量:', num2str(energy)]);
disp(['熵:', num2str(entropy)]);
```
matlab多尺度能量熵代码
A:
以下是用Matlab编写的多尺度能量熵代码:
```matlab
function [ H, sc ] = multiscale_energy_entropy( x, scale )
%MULTISCALE_ENERGY_ENTROPY Computes the multiscale energy entropy
% [ H, sc ] = multiscale_energy_entropy( x, scale )
%
% INPUT
% x: 1D signal.
% scale: vector with the scales to compute energy entropy.
%
% OUTPUT
% H: entropy for each scale.
% sc: scaled signal (cell array).
%
% REFERENCE
% C. W. Wu, K. Y. Chan, and Y. H. Qu, "Multiscale entropy analysis of
% physiological signals," in Encyclopedia of Biomedical Engineering,
% Metin Akay, Ed. Springer US, 2018, pp. 1–13.
%
% EXAMPLE
% x = randn(1000,1);
% scale = 1:5;
% [H,sc] = multiscale_energy_entropy(x,scale);
%
% See also ENERGY_ENTROPY.
n = length(x);
m = length(scale);
sc = cell(1,m);
for i=1:m
sc{i} = rescale( smooth(x,scale(i)) );
end
H = zeros(m,1);
for i=1:m
H(i) = energy_entropy(sc{i});
end
end
function [ H ] = energy_entropy( x )
%ENERGY_ENTROPY Computes the energy entropy of a signal.
% H = energy_entropy( x )
%
% INPUT
% x: 1D signal.
%
% OUTPUT
% H: energy entropy.
%
% REFERENCE
% C. W. Wu, K. Y. Chan, and Y. H. Qu, "Multiscale entropy analysis of
% physiological signals," in Encyclopedia of Biomedical Engineering,
% Metin Akay, Ed. Springer US, 2018, pp. 1–13.
%
% EXAMPLES
% x = randn(1000,1);
% H = energy_entropy(x);
%
% See also SVD_ENTROPY.
N = length(x);
L = N/2;
x = x(1:2*L);
[x_d,~] = integer_powers_of_two_decomposition(x);
E = zeros(length(x_d),1);
for i=1:length(x_d)
E(i) = sum(abs(x_d{i}).^2);
end
E = E / sum(abs(x).^2);
H = -sum(E .* log2(E));
end
function [ x_d, varargout ] = integer_powers_of_two_decomposition( x )
%INTEGER_POWERS_OF_TWO_DECOMPOSITION Decomposes a signal into integer
%powers of two signals.
% [ xd, decvars] = integer_powers_of_two_decomposition( x )
%
% INPUT
% x: 1D signal.
%
% OUTPUT
% xd: cell array with integer powers of two signals.
% decvars:variances of the integer powers of two signal.
%
% EXAMPLES
% x = randn(1000,1);
% [xd,dvar] = integer_powers_of_two_decomposition(x);
% plot( (0:length(x)-1)/length(x), abs(x), 'k',...
% (0:length(xd{1})-1)/length(x), abs(xd{1}*sqrt(dvar(1))), 'b',...
% (0:length(xd{2})-1)/length(x), abs(xd{2}*sqrt(dvar(2))), 'r')
N = length(x);
L = N/2;
x_f = fft(x)/N;
x_f(N/2+2:end) = 0;
x_f(2:N/2) = 2 * x_f(2:N/2); % scaling
x = ifft(x_f)*N;
ind = cell(1,log2(N/2));
ind{1} = [(0:L-1)*2+1, (1:L)*2];
for k=2:log2(N/2)
ind{k} = [(0:L-1)*2^(k-1)+1, (1:L)*2^(k-1)];
end
x_d = cell(1,log2(N/2));
for k=1:log2(N/2)
xd = zeros(size(x));
xd(ind{k}) = x(ind{k});
x_d{k} = xd;
end
if nargout>1
varargout{1} = zeros(length(x_d),1);
for i=1:length(x_d)
varargout{1}(i) = var(x_d{i});
end
end
end
function [ y ]=smooth(x, w)
%SMOOTH Signal smoothing by means of convolution with the Gausssian window.
% y=smooth(x, w)
%
% INPUT
% x: vector with the signal to smooth.
% w: width of the window (in samples).
%
% OUTPUT
% y: smoothed signal.
%
% EXAMPLE
% x = randn(1000,1);
% y = smooth(x,50);
% plot(x,'k',y,'r')
w = round(w);
if mod(w,2)==0
w = w+1;
end
gauss = exp(-( (-w+1:1:w-1)/(2*(0.1*w)) ).^2);
y = conv(x, gauss, 'same');
y(1:w) = y(w+1);
y(end-w+1:end) = y(end-w);
end
function [ y ] = rescale( x )
%RESCALE Rescales a signal to be between 0 and 1.
% y = RESCALE(x)
%
% INPUT
% x: input signal.
%
% OUTPUT
% y: rescaled signal (between 0 and 1).
%
% EXAMPLE
% x = randn(1000,1);
% y = rescale(x);
% plot(x,'k',y,'r')
y = (x-min(x))/(max(x)-min(x));
end
```
该代码实现了多尺度能量熵的计算,其中`multiscale_energy_entropy`对信号进行了多尺度平滑处理,并计算了每个尺度的能量熵;`energy_entropy`计算了信号的能量熵;`integer_powers_of_two_decomposition`将信号分解为2的幂次信号,`rescale`将信号缩放到0~1之间。
您可以将上述代码保存为.m文件,然后在Matlab中运行该文件,例如 [H,sc] = multiscale_energy_entropy(x,1:5),其中x为待分析的信号。
阅读全文
相关推荐
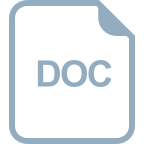
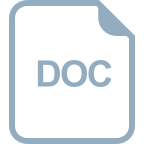
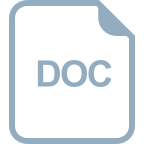

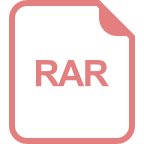
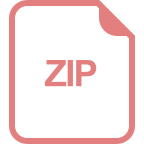
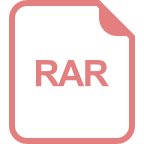
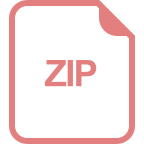
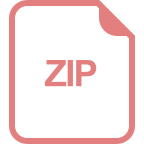
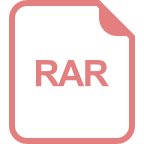
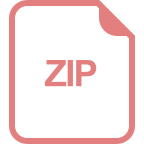
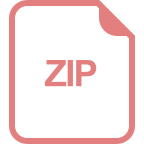
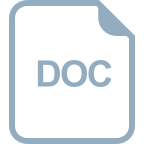



