解释代码: elif isinstance(m0, nn.Conv2d): idx0 = np.squeeze(np.argwhere(np.asarray(start_mask.cpu().numpy()))) idx1 = np.squeeze(np.argwhere(np.asarray(end_mask.cpu().numpy()))) print('In shape: {:d} Out shape:{:d}'.format(idx0.shape[0], idx1.shape[0])) w = m0.weight.data[:, idx0, :, :].clone() w = w[idx1, :, :, :].clone() m1.weight.data = w.clone()
时间: 2023-06-06 08:06:24 浏览: 62
这是一个 PyTorch 模型中网络层权重剪枝的代码段,主要功能是根据指定的起始和终止剪枝掩码,将卷积层的输入通道和输出通道进行筛选和更新。具体来说,首先从起始和终止掩码中取出相关的通道索引,然后根据这些索引对卷积层的权重张量进行裁剪和更新,最终得到一个新的卷积层。
相关问题
解释一下代码:def _init_weight(self): for m in self.modeles(): if isinstance(m, nn.Conv1d): torch.nn.init.kaiming_normal_(m.weight) elif isinstance(m, nn.Linear): torch.nn.init.kaiming_normal_(m.weight)
这段代码定义了一个名为`_init_weight`的方法,它用于对类中的模型权重进行初始化。下面是这个方法的解释:
1. `for m in self.modeles():`:这行代码使用了一个for循环,遍历了类中的所有模型。这里的`self.modeles()`表示调用类中的`modeles`方法,该方法返回一个包含所有模型的列表。
2. `if isinstance(m, nn.Conv1d):`:这行代码使用了Python中的`isinstance`函数来检查当前模型是否是`nn.Conv1d`类型的模型。如果当前模型是卷积模型,则执行下面的语句。
3. `torch.nn.init.kaiming_normal_(m.weight)`:这行代码使用了PyTorch中的`torch.nn.init.kaiming_normal_`函数,对当前模型的权重进行了初始化。这个函数使用了Kaiming正态分布初始化方法。
4. `elif isinstance(m, nn.Linear):`:这行代码使用了Python中的`isinstance`函数来检查当前模型是否是`nn.Linear`类型的模型。如果当前模型是全连接模型,则执行下面的语句。
5. `torch.nn.init.kaiming_normal_(m.weight)`:这行代码同样使用了PyTorch中的`torch.nn.init.kaiming_normal_`函数,对当前模型的权重进行了初始化。
综上所述,这段代码的作用是对类中所有的卷积模型和全连接模型的权重进行初始化,以提高模型的训练效果。这里使用的是Kaiming正态分布初始化方法,它能够使得模型更加稳定和收敛更快。
for m in self.modules(): if isinstance(m, nn.Conv2d) or isinstance(m, nn.ConvTranspose2d): kaiming_normal_(m.weight, 0.1) if m.bias is not None: constant_(m.bias, 0) elif isinstance(m, nn.BatchNorm2d): constant_(m.weight, 1) constant_(m.bias, 0)
这段代码是模型类StrainNetF中的一个方法,用于初始化模型的重和偏置。
在这个方法中,使用了一个循环遍历型的所有子模块(包括卷积层、转置卷积层和批量归一化层)。
对于卷积层和转置卷积层,使用了kaiming_normal_函数来初始化权重,将权重的标准差设置为0.1。如果存在偏置项,将偏置项初始化为0。
对于批量归一化层,将权重项初始化为1,偏置项初始化为0。
这些初始化操作有助于模型的收敛和训练效果。
如果还有其他问题,请继续提问。
相关推荐
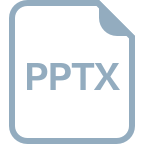
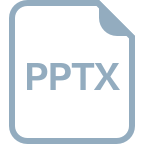













