class Partial_conv3(nn.Module): def __init__(self, dim, n_div, forward): super().__init__() self.dim_conv3 = dim // n_div self.dim_untouched = dim - self.dim_conv3 self.partial_conv3 = nn.Conv2d(self.dim_conv3, self.dim_conv3, 3, 1, 1, bias=False) self.global_pool = GlobalAvgPool2d() if forward == 'slicing': self.forward = self.forward_slicing elif forward == 'split_cat': self.forward = self.forward_split_cat else: raise NotImplementedError def forward_slicing(self, x: Tensor) -> Tensor: # only for inference x = x.clone() # !!! Keep the original input intact for the residual connection later x[:, :self.dim_conv3, :, :] = self.partial_conv3(x[:, :self.dim_conv3, :, :]) return x def forward_split_cat(self, x: Tensor) -> Tensor: x1, x2 = torch.split(x, [self.dim_conv3, self.dim_untouched], dim=1) x1 = self.partial_conv3(x1) x1 = self.global_pool(x1) x = torch.cat((x1, x2), 1) return x在这段代码中插入全局平均池化做通道增强的模块
时间: 2024-02-14 12:15:21 浏览: 192
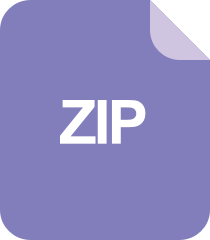
partial_shading_multimodal_success.zip_Success_partial shading_光
你可以在forward_slicing和forward_split_cat的输出结果之前加入一个全局平均池化层进行通道增强,代码如下:
```
class Partial_conv3(nn.Module):
def __init__(self, dim, n_div, forward):
super().__init__()
self.dim_conv3 = dim // n_div
self.dim_untouched = dim - self.dim_conv3
self.partial_conv3 = nn.Conv2d(self.dim_conv3, self.dim_conv3, 3, 1, 1, bias=False)
self.global_pool = GlobalAvgPool2d()
self.channel_enhance = nn.Sequential(
nn.Conv2d(dim, dim, kernel_size=1, stride=1, padding=0, bias=False),
nn.BatchNorm2d(dim),
nn.ReLU(inplace=True),
self.global_pool
)
if forward == 'slicing':
self.forward = self.forward_slicing
elif forward == 'split_cat':
self.forward = self.forward_split_cat
else:
raise NotImplementedError
def forward_slicing(self, x: Tensor) -> Tensor:
# only for inference
x = x.clone() # !!! Keep the original input intact for the residual connection later
x[:, :self.dim_conv3, :, :] = self.partial_conv3(x[:, :self.dim_conv3, :, :])
x = self.channel_enhance(x)
return x
def forward_split_cat(self, x: Tensor) -> Tensor:
x1, x2 = torch.split(x, [self.dim_conv3, self.dim_untouched], dim=1)
x1 = self.partial_conv3(x1)
x1 = self.channel_enhance(x1)
x = torch.cat((x1, x2), 1)
return x
```
这里使用了一个nn.Sequential模块,包含了一个1x1的卷积层、BatchNorm层、ReLU激活层和全局平均池化层,对输入的特征图进行通道增强,从而提高模型的性能。在forward_slicing和forward_split_cat的输出结果之前,将输入特征图通过这个通道增强模块之后再输出。
阅读全文
相关推荐
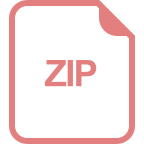
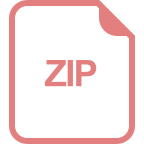




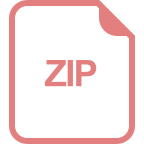






