自定义根号下函数C语言
时间: 2024-09-30 08:08:45 浏览: 3
在C语言中,由于标准库函数并未提供求平方根的内置函数sqrt()用于计算某个数的正平方根,如果你想自定义一个根号下函数,通常会利用数学库中的数学运算来实现,比如使用牛顿法或者泰勒级数展开等数值方法。下面是一个简单的例子,展示如何使用泰勒级数近似法来实现一个阶乘迭代的算法来逼近平方根:
```c
#include <stdio.h>
#include <math.h>
// 自定义的平方根函数,这里仅给出基本思路,实际应用中需要设置精度控制和循环条件
double custom_sqrt(double num) {
double guess = num / 2; // 初始猜测值
double prev_guess = 0;
while (fabs(guess - prev_guess) > 0.00001) { // 设置一个足够小的精度
prev_guess = guess;
guess = (guess + num / guess) / 2; // 使用泰勒级数公式 x = (x + n/x)/2 迭代
}
return guess;
}
int main() {
double number = 16.0;
printf("The square root of %.2f is approximately %.2f\n", number, custom_sqrt(number));
return 0;
}
```
相关问题
c语言根号函数
C语言中可以使用数学库函数 `sqrt()` 来计算一个数的平方根。若要使用该函数,需要在代码中包含头文件 `math.h`,以便能够调用 `sqrt()` 函数。以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <math.h>
int main() {
double x = 16.0;
double result = sqrt(x);
printf("The square root of %f is %f\n", x, result);
return 0;
}
```
运行结果为:
```
The square root of 16.000000 is 4.000000
```
其中,`double` 是 C 语言中的一种数据类型,用于表示双精度浮点数。`sqrt()` 函数的参数和返回值都是 `double` 类型。在上述示例中,我们计算了变量 `x` 的平方根,并将结果存储在变量 `result` 中,最后使用 `printf()` 函数输出结果。
三次根号怎么写c语言
在C语言中,计算一个数的三次根号(也就是立方根)可以通过使用 `pow` 函数实现,该函数在 `math.h` 头文件中声明。`pow` 函数用于计算 x 的 y 次幂,即 x^y。为了计算一个数的三次根号,你需要计算该数的 1/3 次幂,即 `pow(x, 1.0/3.0)`。
下面是一个简单的示例代码,展示如何使用 `pow` 函数在C语言中计算三次根号:
```c
#include <stdio.h>
#include <math.h>
int main() {
double num, result;
// 输入一个数值
printf("请输入一个数,计算它的三次根号: ");
scanf("%lf", &num);
// 计算三次根号
result = pow(num, 1.0/3.0);
// 输出结果
printf("%lf 的三次根号是: %lf\n", num, result);
return 0;
}
```
在这段代码中,首先包含了必要的头文件,然后在 `main` 函数中读取用户输入的数值,并使用 `pow` 函数计算其三次根号,最后将结果输出到控制台。
相关推荐
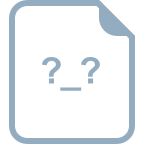
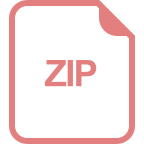
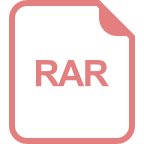












