编写一个学生成绩管理系统。学生的属性包括学号、姓名、年龄等。每个学生要学习若干课程,每门课程有平时成绩、期中考试成绩、实习成绩、期末考试成绩以及总评成绩等多个成绩,其中平时成绩的数目不定(因为不同课程的教师布置的平时作业不同),而总评成绩是其它成绩的平均值。请先设计合理的类存放这些信息,然后设计程序完成以下功能: (1)列出某个学生的所有成绩; (2)列出某门课程每个学生的总评成绩,及所有学生总评成绩的总评分; (3)分区段统计某门课程的学生总评成绩,例如60分以下的学生人数、60至70分的学生人数等。给出完整Java代码并写出设计思路
时间: 2024-02-20 10:58:30 浏览: 21
设计思路:
1. 设计一个Student类,包括学号、姓名、年龄等属性,以及学生所学习的课程列表。在Student类中添加方法addCourse,用于添加课程;getCourse,用于获取某门课程的成绩。
2. 设计一个Course类,包括课程名称、平时成绩、期中考试成绩、实习成绩、期末考试成绩以及总评成绩等属性。在Course类中添加方法calculateTotalScore,用于计算总评成绩。
3. 设计一个GradeManagementSystem类,用于实现程序功能。包括:添加学生、添加课程、查询某个学生的所有成绩、查询某门课程每个学生的总评成绩及所有学生总评成绩的总评分,分区段统计某门课程的学生总评成绩。
Java代码如下:
```
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class Student {
private String id;
private String name;
private int age;
private Map<String, Course> courses;
public Student(String id, String name, int age) {
this.id = id;
this.name = name;
this.age = age;
this.courses = new HashMap<>();
}
public void addCourse(Course course) {
courses.put(course.getName(), course);
}
public List<Course> getAllCourses() {
return new ArrayList<>(courses.values());
}
public String getId() {
return id;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
public class Course {
private String name;
private List<Double> dailyScores;
private double midtermScore;
private double internshipScore;
private double finalScore;
private double totalScore;
public Course(String name, List<Double> dailyScores, double midtermScore, double internshipScore, double finalScore) {
this.name = name;
this.dailyScores = dailyScores;
this.midtermScore = midtermScore;
this.internshipScore = internshipScore;
this.finalScore = finalScore;
this.totalScore = calculateTotalScore();
}
public String getName() {
return name;
}
public double getTotalScore() {
return totalScore;
}
private double calculateTotalScore() {
double sum = midtermScore + internshipScore + finalScore;
for (double score : dailyScores) {
sum += score;
}
return sum / (dailyScores.size() + 3);
}
}
public class GradeManagementSystem {
private List<Student> students;
public GradeManagementSystem() {
this.students = new ArrayList<>();
}
public void addStudent(Student student) {
students.add(student);
}
public void addCourseToStudent(String studentId, Course course) {
for (Student student : students) {
if (student.getId().equals(studentId)) {
student.addCourse(course);
break;
}
}
}
public List<Course> getAllCoursesOfStudent(String studentId) {
for (Student student : students) {
if (student.getId().equals(studentId)) {
return student.getAllCourses();
}
}
return null;
}
public double getAverageTotalScoreOfCourse(String courseName) {
double sum = 0;
int count = 0;
for (Student student : students) {
for (Course course : student.getAllCourses()) {
if (course.getName().equals(courseName)) {
sum += course.getTotalScore();
count++;
}
}
}
if (count == 0) {
return 0;
}
return sum / count;
}
public Map<String, Integer> getSectionCountOfCourse(String courseName, int interval) {
Map<String, Integer> sectionCountMap = new HashMap<>();
for (Student student : students) {
for (Course course : student.getAllCourses()) {
if (course.getName().equals(courseName)) {
int totalScore = (int) course.getTotalScore();
int section = totalScore / interval;
String key = section * interval + "-" + (section + 1) * interval;
sectionCountMap.put(key, sectionCountMap.getOrDefault(key, 0) + 1);
}
}
}
return sectionCountMap;
}
}
```
在main函数中可以进行测试:
```
public static void main(String[] args) {
GradeManagementSystem system = new GradeManagementSystem();
// 添加学生
Student student1 = new Student("001", "张三", 18);
Student student2 = new Student("002", "李四", 19);
system.addStudent(student1);
system.addStudent(student2);
// 添加课程
Course course1 = new Course("Java", Arrays.asList(80.0, 85.0, 90.0), 85.0, 90.0, 95.0);
Course course2 = new Course("Python", Arrays.asList(90.0, 95.0), 90.0, 85.0, 95.0);
system.addCourseToStudent("001", course1);
system.addCourseToStudent("001", course2);
system.addCourseToStudent("002", course1);
// 列出某个学生的所有成绩
List<Course> courses = system.getAllCoursesOfStudent("001");
for (Course course : courses) {
System.out.println(course.getName() + "总评成绩:" + course.getTotalScore());
}
// 列出某门课程每个学生的总评成绩及所有学生总评成绩的总评分
double average = system.getAverageTotalScoreOfCourse("Java");
System.out.println("Java所有学生总评成绩的平均分:" + average);
// 分区段统计某门课程的学生总评成绩
Map<String, Integer> sectionCountMap = system.getSectionCountOfCourse("Java", 10);
for (Map.Entry<String, Integer> entry : sectionCountMap.entrySet()) {
System.out.println(entry.getKey() + "分段的学生人数:" + entry.getValue());
}
}
```
相关推荐
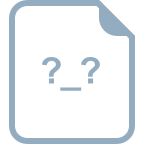
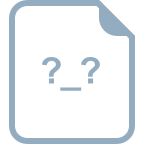
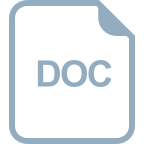














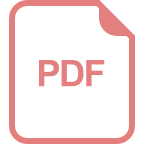
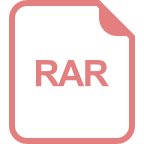