Java写出计算一棵二叉树深度的算法
时间: 2024-12-12 18:26:16 浏览: 1
在Java中,我们可以使用递归的方式来计算一棵二叉树的深度,也就是树中最远节点到根节点的距离。下面是一个简单的示例:
```java
public class TreeNode {
int val;
TreeNode left;
TreeNode right;
// 构造函数和 getters/setters 省略
}
public class BinaryTreeDepth {
public static int maxDepth(TreeNode root) {
if (root == null) {
return 0; // 如果根节点为空,深度为0
} else {
// 递归计算左子树和右子树的最大深度
int leftDepth = maxDepth(root.left);
int rightDepth = maxDepth(root.right);
// 返回两子树深度较大的那个加1,即当前节点的深度
return Math.max(leftDepth, rightDepth) + 1;
}
}
// 测试代码
public static void main(String[] args) {
TreeNode tree = new TreeNode(1); // 假设这是一棵树的基础结构
// ... 继续构建树
int depth = maxDepth(tree);
System.out.println("The maximum depth of the binary tree is: " + depth);
}
}
```
在这个算法中,如果树为空,则返回0;否则,对左右子树分别递归地求最大深度,最终的结果是左右子树深度较大者加1。
阅读全文
相关推荐
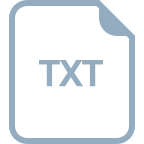
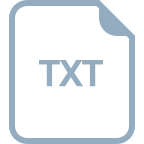
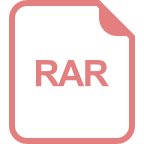





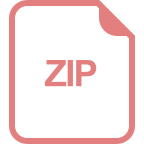
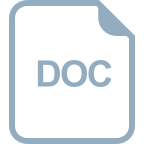
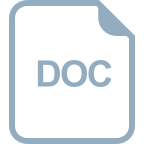
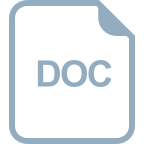
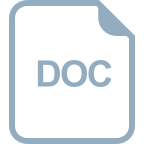
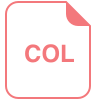
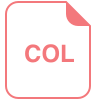


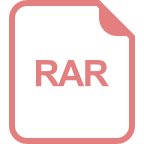