串口波特率设置为4800,89C52接收PC机串口发送的8位数据,进行判断: n 若接收到的8位数据为“1”,通过串口向PC机传送“Y”; n 若接收到的8位数据不为“1”,通过串口向PC机传送“N”
时间: 2024-03-18 19:40:04 浏览: 45
可以使用C语言编写程序实现该功能。以下是一个简单的程序示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <errno.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int set_interface_attribs(int fd, int speed)
{
struct termios tty;
memset(&tty, 0, sizeof tty);
if (tcgetattr(fd, &tty) != 0)
{
printf("error %d from tcgetattr", errno);
return -1;
}
cfsetospeed(&tty, speed);
cfsetispeed(&tty, speed);
tty.c_cflag |= (CLOCAL | CREAD);
tty.c_cflag &= ~CSIZE;
tty.c_cflag |= CS8;
tty.c_cflag &= ~PARENB;
tty.c_cflag &= ~CSTOPB;
tty.c_cflag &= ~CRTSCTS;
if (tcsetattr(fd, TCSANOW, &tty) != 0)
{
printf("error %d from tcsetattr", errno);
return -1;
}
return 0;
}
int main()
{
const char *portname = "/dev/ttyS0"; // 串口设备名称
int fd = open(portname, O_RDWR | O_NOCTTY | O_SYNC);
if (fd < 0)
{
printf("error %d opening %s: %s", errno, portname, strerror(errno));
return -1;
}
set_interface_attribs(fd, B4800); // 设置波特率为4800
while (1)
{
unsigned char buf[8];
int n = read(fd, buf, sizeof buf); // 从串口读取8位数据
if (n < 0)
{
printf("error %d reading from %s: %s", errno, portname, strerror(errno));
break;
}
else if (n == 0)
{
printf("no data available\n");
continue;
}
else
{
if (buf[0] == '1') // 判断接收到的数据是否为1
{
write(fd, "Y", 1); // 发送Y
}
else
{
write(fd, "N", 1); // 发送N
}
}
}
close(fd);
return 0;
}
```
该程序通过串口读取8位数据,并根据数据是否为1来发送Y或N。注意,程序中使用的是Linux系统下的串口设备名称`/dev/ttyS0`,如果你使用的是Windows系统,需要将设备名称修改为COMx的形式。另外,程序中没有进行错误处理,实际应用中需要根据需要添加相应的错误处理。
相关推荐
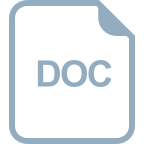

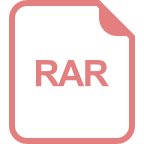
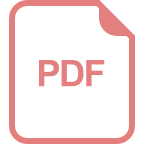









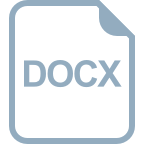
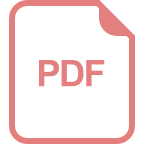
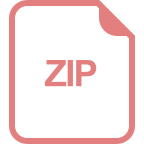