C语言贪吃蛇程序代码完整
时间: 2024-12-22 20:10:14 浏览: 3
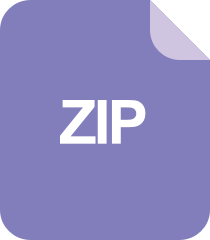
CppGuide-贪吃蛇c语言程序代码
编写一个完整的C语言贪吃蛇游戏代码会比较复杂,因为涉及到图形界面、键盘输入处理、游戏循环以及蛇体动态更新等多个部分。下面是一个简化的文本模式贪吃蛇的示例,仅包含基本的游戏规则,不包括图形化:
```c
#include <stdio.h>
#include <stdlib.h>
#define BOARD_SIZE 10
#define HEAD 0
#define APPLE 1
typedef struct {
int x, y;
} Position;
Position snake[5] = { {0, 0}, {1, 0}, {2, 0}, {1, 1}, {1, 2} };
int apple_x, apple_y;
int score = 0;
int direction = 'D'; // 默认向右
void moveSnake() {
int newX, newY;
switch (direction) {
case 'U':
newY = snake[HEAD].y - 1;
break;
case 'D':
newY = snake[HEAD].y + 1;
break;
case 'L':
newX = snake[HEAD].x - 1;
break;
case 'R':
newX = snake[HEAD].x + 1;
break;
}
if (newX >= 0 && newX < BOARD_SIZE && newY >= 0 && newY < BOARD_SIZE && snake[newY * BOARD_SIZE + newX] != HEAD) {
for (int i = snake.length - 1; i > HEAD; i--) {
snake[i] = snake[i - 1];
}
snake[HEAD].x = newX;
snake[HEAD].y = newY;
if (snake[HEAD].x == apple_x && snake[HEAD].y == apple_y) {
score++;
apple_x = rand() % BOARD_SIZE;
apple_y = rand() % BOARD_SIZE;
} else {
apple_x = snake[HEAD].x;
apple_y = snake[HEAD].y;
}
}
}
int main() {
srand(time(NULL));
apple_x = rand() % BOARD_SIZE;
apple_y = rand() % BOARD_SIZE;
while (1) {
printf("Score: %d\n", score);
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
if (i == snake[HEAD].x && j == snake[HEAD].y)
printf("H");
else if (i == apple_x && j == apple_y)
printf("A");
else
printf(".");
}
printf("\n");
}
moveSnake();
getchar(); // 等待用户按键
direction = getDirection(); // 获取并更新方向
}
return 0;
}
// 用户输入方向函数,实际项目可能需要更复杂的输入处理
char getDirection() {
char input;
scanf("%c", &input);
return input;
}
```
阅读全文
相关推荐
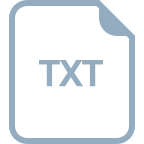
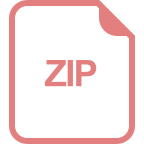















