用verilog写一个RX FIFO代码
时间: 2023-09-22 10:06:22 浏览: 142
### 回答1:
我可以回答这个问题。以下是一个简单的 RX FIFO 的 Verilog 代码:
module rxfifo (
input clk,
input rst,
input rx_data,
input rx_valid,
output reg [7:] data_out,
output reg out_valid
);
reg [7:] fifo [:7];
reg [2:] wr_ptr;
reg [2:] rd_ptr;
reg [2:] count;
always @(posedge clk) begin
if (rst) begin
wr_ptr <= ;
rd_ptr <= ;
count <= ;
out_valid <= ;
end else begin
if (rx_valid) begin
fifo[wr_ptr] <= rx_data;
wr_ptr <= wr_ptr + 1;
count <= count + 1;
end
if (count > ) begin
data_out <= fifo[rd_ptr];
rd_ptr <= rd_ptr + 1;
count <= count - 1;
out_valid <= 1;
end else begin
out_valid <= ;
end
end
end
endmodule
希望这可以帮助你。
### 回答2:
使用Verilog语言编写一个RX FIFO(接收端先进先出)的代码可以实现接收数据的缓存功能,代码如下所示:
```verilog
module RX_FIFO (
input clk,
input reset,
input data_in,
input validate_in,
output reg full,
output reg empty,
output reg data_out
);
parameter SIZE = 8; // 设置FIFO的大小为8
reg [SIZE-1:0] fifo [0:SIZE-1];
reg [2:0] head;
reg [2:0] tail;
always @(posedge clk or posedge reset) begin
if (reset) begin
empty <= 1'b1;
full <= 1'b0;
head <= 0;
tail <= 0;
end else begin
if (validate_in && !full) begin
fifo[tail] <= data_in;
tail <= (tail+1)%SIZE;
empty <= 1'b0;
if ((tail+1) == head || (tail == SIZE-1 && head == 0))
full <= 1'b1;
end
if (validate_in && !empty) begin
data_out <= fifo[head];
head <= (head+1)%SIZE;
full <= 1'b0;
if (head == tail)
empty <= 1'b1;
end
end
end
endmodule
```
这段代码定义了一个RX_FIFO模块,模块内部使用了一个数组`fifo`作为接收缓存,大小为8。其中`head`和`tail`指针分别指向缓存中的队首和队尾。
在每次时钟上升沿或复位信号(reset)发生时,会根据输入信号`validate_in`和缓存状态进行相应的操作。当`validate_in`为高电平且缓存不满时,将输入信号`data_in`存放到缓存中的`tail`位置,并更新`tail`指针。当`validate_in`为高电平且缓存不为空时,从缓存中的`head`位置取出数据,并更新`head`指针。
同时,根据`head`和`tail`指针的关系,判断缓存是否为空或已满,并更新对应的状态信号`empty`和`full`。
这段代码使用Verilog语言编写,通过调用模块,可以在FPGA或ASIC等硬件平台实现接收端的FIFO功能。
### 回答3:
RX FIFO是一种接收(RX)端的先进先出(FIFO)缓冲区,用于暂存从外部设备接收到的数据。下面是一个用Verilog语言编写的RX FIFO代码示例:
```verilog
module rx_fifo (
input wire clk, // 时钟信号
input wire rst, // 复位信号
input wire rx_data, // 接收到的数据信号
input wire rx_valid, // 接收到有效数据的信号
output wire rx_ready, // 接收端准备好的信号
output wire [7:0] rx_out_data // 从FIFO中取出的数据信号
);
parameter WIDTH = 8; // FIFO数据位宽
parameter DEPTH = 16; // FIFO深度
reg [WIDTH-1:0] buffer [0:DEPTH-1]; // FIFO存储buffer
reg [4:0] head = 0; // FIFO头指针
reg [4:0] tail = 0; // FIFO尾指针
reg count = 0; // FIFO中的数据计数
wire full = (count == DEPTH); // FIFO是否已满
wire empty = (count == 0); // FIFO是否为空
// 时钟边沿触发的处理逻辑
always @(posedge clk) begin
if (rst) begin // 复位
for (integer i = 0; i < DEPTH; i = i + 1) begin
buffer[i] <= 0;
end
head <= 0;
tail <= 0;
count <= 0;
end else begin
if (rx_valid && !full) begin // 接收到有效数据且FIFO未满
buffer[tail] <= rx_data; // 存储数据到buffer
count <= count + 1; // 数据计数加1
tail <= tail + 1; // 尾指针递增
end
if (rx_ready && !empty) begin // 接收端准备好且FIFO非空
rx_out_data <= buffer[head]; // 取出数据
count <= count - 1; // 数据计数减1
head <= head + 1; // 头指针递增
end
end
end
// 用于向外部模块表示FIFO的状态
assign rx_ready = !full; // 当FIFO未满时接收端准备好
endmodule
```
该代码使用Verilog语言实现了一个有限深度(16个数据位)的RX FIFO,当接收到有效数据时,数据将存储在一个大小为8bit的buffer数组中。当接收端准备好(rx_ready为高电平)时,从FIFO中取出数据并输出到rx_out_data信号中。复位时,FIFO的buffer数组、头指针(head)、尾指针(tail)和数据计数(count)都将被重置。
阅读全文
相关推荐
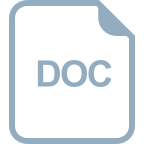
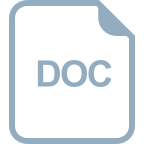
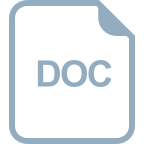

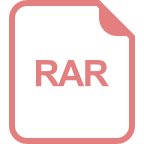
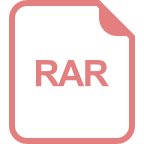
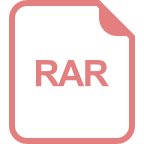


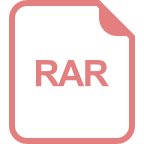
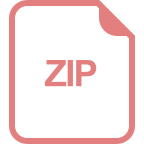
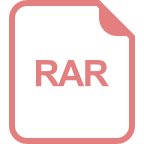






