凸多边形三角剖分伪代码及代码实现
时间: 2023-07-02 12:24:20 浏览: 118
凸多边形三角剖分,可以使用分治法来实现。伪代码如下:
```
function TriangulateConvexPolygon(polygon):
if polygon.size() == 3:
return [polygon];
else:
// 找到多边形的中心点
center = findCenter(polygon);
// 找到离中心点最远的边
farthestEdge = findFarthestEdge(polygon, center);
// 将多边形沿着这条边分成两个子多边形
subPolygons = splitPolygon(polygon, farthestEdge);
// 递归地对子多边形进行三角剖分
leftTriangles = TriangulateConvexPolygon(subPolygons[0]);
rightTriangles = TriangulateConvexPolygon(subPolygons[1]);
// 合并子多边形的三角剖分结果
return mergeTriangles(leftTriangles, rightTriangles);
```
其中,`findCenter` 函数可以找到多边形的中心点;`findFarthestEdge` 函数可以找到离中心点最远的边;`splitPolygon` 函数可以将多边形沿着指定的边分成两个子多边形;`mergeTriangles` 函数可以将两个子多边形的三角剖分结果合并起来。
以下是一个 Python 实现的示例代码:
```
from typing import List
class Point:
def __init__(self, x: float, y: float):
self.x = x
self.y = y
class Edge:
def __init__(self, p1: Point, p2: Point):
self.p1 = p1
self.p2 = p2
class Triangle:
def __init__(self, p1: Point, p2: Point, p3: Point):
self.p1 = p1
self.p2 = p2
self.p3 = p3
def TriangulateConvexPolygon(polygon: List[Point]) -> List[Triangle]:
if len(polygon) == 3:
return [Triangle(polygon[0], polygon[1], polygon[2])]
else:
center = findCenter(polygon)
farthestEdge = findFarthestEdge(polygon, center)
subPolygons = splitPolygon(polygon, farthestEdge)
leftTriangles = TriangulateConvexPolygon(subPolygons[0])
rightTriangles = TriangulateConvexPolygon(subPolygons[1])
return mergeTriangles(leftTriangles, rightTriangles)
def findCenter(polygon: List[Point]) -> Point:
n = len(polygon)
cx = sum(p.x for p in polygon) / n
cy = sum(p.y for p in polygon) / n
return Point(cx, cy)
def findFarthestEdge(polygon: List[Point], center: Point) -> Edge:
farthestEdge = None
maxDist = -1
for i in range(len(polygon)):
j = (i + 1) % len(polygon)
edge = Edge(polygon[i], polygon[j])
dist = distance(center, edge)
if dist > maxDist:
farthestEdge = edge
maxDist = dist
return farthestEdge
def splitPolygon(polygon: List[Point], edge: Edge) -> List[List[Point]]:
i = polygon.index(edge.p1)
j = polygon.index(edge.p2)
if i < j:
subPolygon1 = polygon[i:j+1]
subPolygon2 = polygon[j:] + polygon[:i+1]
else:
subPolygon1 = polygon[j:i+1]
subPolygon2 = polygon[i:] + polygon[:j+1]
return [subPolygon1, subPolygon2]
def distance(point: Point, edge: Edge) -> float:
x1, y1 = edge.p1.x, edge.p1.y
x2, y2 = edge.p2.x, edge.p2.y
x0, y0 = point.x, point.y
dx = x2 - x1
dy = y2 - y1
if dx == 0 and dy == 0:
return ((x1 - x0) ** 2 + (y1 - y0) ** 2) ** 0.5
t = ((x0 - x1) * dx + (y0 - y1) * dy) / (dx * dx + dy * dy)
if t < 0:
return ((x1 - x0) ** 2 + (y1 - y0) ** 2) ** 0.5
elif t > 1:
return ((x2 - x0) ** 2 + (y2 - y0) ** 2) ** 0.5
else:
xp = x1 + t * dx
yp = y1 + t * dy
return ((xp - x0) ** 2 + (yp - y0) ** 2) ** 0.5
def mergeTriangles(triangles1: List[Triangle], triangles2: List[Triangle]) -> List[Triangle]:
mergedTriangles = []
for t in triangles1 + triangles2:
if isInPolygon(t.p1, triangles2) and isInPolygon(t.p2, triangles2) and isInPolygon(t.p3, triangles2):
continue
else:
mergedTriangles.append(t)
return mergedTriangles
def isInPolygon(point: Point, triangles: List[Triangle]) -> bool:
for t in triangles:
if isInTriangle(point, t):
return True
return False
def isInTriangle(point: Point, triangle: Triangle) -> bool:
p1, p2, p3 = triangle.p1, triangle.p2, triangle.p3
d1 = sign(point, p1, p2)
d2 = sign(point, p2, p3)
d3 = sign(point, p3, p1)
has_neg = (d1 < 0) or (d2 < 0) or (d3 < 0)
has_pos = (d1 > 0) or (d2 > 0) or (d3 > 0)
return not (has_neg and has_pos)
def sign(p1: Point, p2: Point, p3: Point) -> float:
return (p1.x - p3.x) * (p2.y - p3.y) - (p2.x - p3.x) * (p1.y - p3.y)
# 示例用法
polygon = [Point(0, 0), Point(1, 0), Point(1, 1), Point(0, 1)]
triangles = TriangulateConvexPolygon(polygon)
for t in triangles:
print(t.p1.x, t.p1.y, t.p2.x, t.p2.y, t.p3.x, t.p3.y)
```
注意,以上实现假设输入的多边形是凸多边形。如果输入的是非凸多边形,则需要先对其进行分解成若干个凸多边形,再分别进行三角剖分。
阅读全文
相关推荐
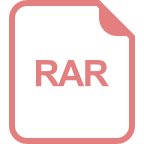

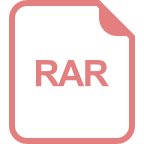
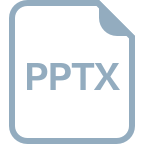
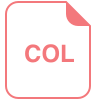
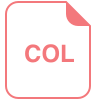
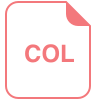
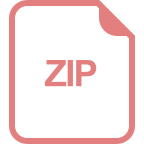
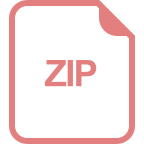
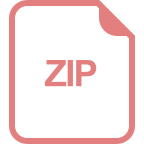
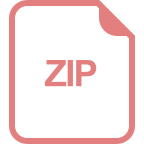