用c语言写两个有序链表列的交集
时间: 2024-10-23 18:09:03 浏览: 37
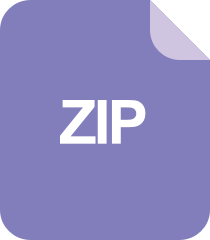
两个有序链表序列的合并_C语言_

在C语言中,可以使用哈希集合(如`unordered_set`)或者双指针法来找到两个有序链表的交集。以下是使用哈希集合的一个简单示例:
```c
#include <stdio.h>
#include <stdbool.h>
#include <stdlib.h>
typedef struct ListNode {
int val;
struct ListNode *next;
} ListNode;
// 创建一个新的链表节点
ListNode* createNode(int val) {
ListNode* node = (ListNode*)malloc(sizeof(ListNode));
if (node != NULL) {
node->val = val;
node->next = NULL;
}
return node;
}
// 将一个链表添加到哈希集合中
void addToListToHashSet(ListNode* head, unordered_set<int>& set) {
ListNode* current = head;
while (current != NULL) {
set.insert(current->val);
current = current->next;
}
}
// 查找两个链表的交集
ListNode* findIntersection(ListNode* list1, ListNode* list2) {
unordered_set<int> set1;
unordered_set<int> set2;
// 遍历第一个链表并插入哈希集合
addToListToHashSet(list1, set1);
// 同时遍历两个链表,如果当前值在set1中,则找到了交集
ListNode* intersection = NULL;
current1 = list1;
current2 = list2;
while (current1 != NULL && current2 != NULL) {
if (set1.find(current2->val) != set1.end()) {
intersection = current2;
break;
}
current1 = current1->next;
current2 = current2->next;
}
return intersection;
}
int main() {
// 测试代码,这里假设你已经创建了list1和list2,并设置了它们的内容
ListNode* list1Intersection = findIntersection(list1, list2);
if (list1Intersection != NULL) {
printf("Intersection found at value: %d\n", list1Intersection->val);
} else {
printf("No intersection found.\n");
}
return 0;
}
```
阅读全文
相关推荐
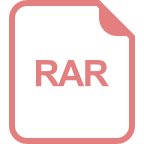
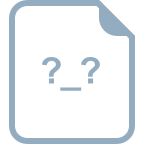







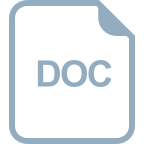







