javafx里面如果点击确认按钮但是输入框为空,会在输入框下面出现一行小字提示不能为空并且程序停止,用fxml怎么写?
时间: 2024-04-20 08:22:45 浏览: 28
使用FXML来创建JavaFX界面可以更好地分离布局和逻辑。你可以按照以下步骤来实现在FXML中显示提示信息并停止程序的功能:
1. 创建一个FXML文件,比如`Main.fxml`,并在文件中添加以下代码:
```xml
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.control.Button?>
<?import javafx.scene.control.Label?>
<?import javafx.scene.control.TextField?>
<?import javafx.scene.layout.VBox?>
<VBox xmlns="http://javafx.com/javafx/16" xmlns:fx="http://javafx.com/fxml/1" fx:controller="sample.Controller">
<children>
<TextField fx:id="textField"/>
<Button text="确认" onAction="#confirmButtonClicked"/>
<Label fx:id="promptLabel" style="-fx-text-fill: red;"/>
</children>
</VBox>
```
2. 创建一个Controller类,比如`Controller.java`,并在类中添加以下代码:
```java
package sample;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
public class Controller {
@FXML
private TextField textField;
@FXML
private Label promptLabel;
@FXML
private void confirmButtonClicked(ActionEvent event) {
String inputText = textField.getText();
if (inputText.isEmpty()) {
promptLabel.setText("输入不能为空!");
System.exit(0); // 停止程序
} else {
promptLabel.setText("");
// 在这里可以进行其他操作,比如保存输入内容或执行其他逻辑
}
}
}
```
3. 创建一个`Main`类作为程序的入口,比如`Main.java`,并在类中添加以下代码:
```java
package sample;
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
Parent root = FXMLLoader.load(getClass().getResource("Main.fxml"));
primaryStage.setTitle("Empty Input Validation with FXML");
primaryStage.setScene(new Scene(root, 200, 150));
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
```
这样,你就使用FXML和Controller类实现了在FXML中显示提示信息并停止程序的功能。在上述代码中,`confirmButtonClicked`方法是确认按钮的点击事件处理方法,它会检查输入框的内容是否为空,如果为空,则在标签中显示提示信息"输入不能为空!",并调用`System.exit(0)`来停止程序。你可以根据需要进行修改和扩展。
相关推荐
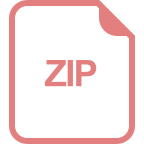














