data = np.array(data) / 255.0 numpy.core._exceptions._ArrayMemoryError: Unable to allocate 71.5 GiB for an array with shape (2400, 1000, 1000, 4) and data type float64这句话显示错误
时间: 2024-02-20 20:58:32 浏览: 105
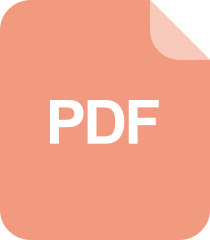
浅谈numpy中np.array()与np.asarray的区别以及.tolist

这个错误提示意味着你的程序尝试分配 71.5 GiB 的内存来存储这个数组,但是你的系统没有这么多可用的内存。因此,你需要对数组进行降维或者使用其他方法来减少内存占用。
一种可行的方法是在读入图片时,不一次性读入所有图片,而是逐个读入并进行处理。以下是修改后的代码:
```python
import os
import numpy as np
from PIL import Image
from keras.layers import Input, Conv2D, MaxPooling2D, UpSampling2D
from keras.models import Model
from sklearn.cluster import KMeans
# 定义卷积自编码器模型
input_img = Input(shape=(1000, 1000, 3))
x = Conv2D(32, (3, 3), activation='relu', padding='same')(input_img)
x = MaxPooling2D((2, 2), padding='same')(x)
x = Conv2D(16, (3, 3), activation='relu', padding='same')(x)
x = MaxPooling2D((2, 2), padding='same')(x)
x = Conv2D(8, (3, 3), activation='relu', padding='same')(x)
encoded = MaxPooling2D((2, 2), padding='same')(x)
x = Conv2D(8, (3, 3), activation='relu', padding='same')(encoded)
x = UpSampling2D((2, 2))(x)
x = Conv2D(16, (3, 3), activation='relu', padding='same')(x)
x = UpSampling2D((2, 2))(x)
x = Conv2D(32, (3, 3), activation='relu')(x)
x = UpSampling2D((2, 2))(x)
decoded = Conv2D(3, (3, 3), activation='sigmoid', padding='same')(x)
autoencoder = Model(input_img, decoded)
autoencoder.compile(optimizer='adam', loss='binary_crossentropy')
# 加载数据集
data = []
for folder in os.listdir("D:/wjd"):
for file in os.listdir("D:/wjd/"+folder):
img = Image.open("D:/wjd/"+folder+"/"+file)
img = img.resize((1000, 1000))
data.append(np.array(img) / 255.0)
# 训练卷积自编码器
autoencoder.fit(np.array(data), np.array(data), epochs=50, batch_size=32)
# 提取特征
encoder = Model(input_img, encoded)
features = encoder.predict(np.array(data))
# 使用KMeans聚类算法将特征分为两类
kmeans = KMeans(n_clusters=2, random_state=0).fit(features)
# 将分类结果保存到对应的文件夹中
for i, label in enumerate(kmeans.labels_):
filename = str(i+1) + ".png"
if label == 0:
os.rename("D:/wjd/"+str(i+1)+".png", "D:/wjd/FCC/"+filename)
else:
os.rename("D:/wjd/"+str(i+1)+".png", "D:/wjd/BCC/"+filename)
```
这个修改后的代码会逐个读入并处理图片,而不是一次性读入所有图片。这样可以减少内存占用,并且在处理大量图片时也可以加快程序的运行速度。
阅读全文
相关推荐
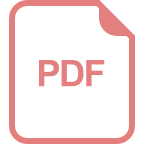
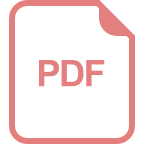
















