python pandas对excel的第一个空单元格写入内容
时间: 2024-10-08 12:21:58 浏览: 40
在pandas中,`read_excel()`函数主要用于读取Excel文件,而如果你想向Excel文件中写入数据,可以先创建一个DataFrame,然后使用`to_excel()`函数将DataFrame保存到Excel文件中。如果要写入第一个空单元格,通常指的是在特定列的第一行插入新的数据。这里有一个简单的例子:
```python
# 假设你已经有了一个DataFrame `new_data` 并且你想写入名为 'filePath' 的Excel文件
new_data = pd.DataFrame({'New Column': ['Data to Write']}, index=[0]) # 创建一个新的DataFrame
# 如果文件中不存在该列,直接写入
new_data.to_excel(filePath, startrow=len(student)+1, header=False, mode='a', index=False)
# 或者如果要追加数据到已有列,但不改变原表头
# 使用`loc`选择你要写入的位置
new_row = {'ID': 1, 'Name': 'John Doe', 'Age': 25} # 新的数据行
student.loc[len(student)] = new_row # 在最后一行添加新行
student.to_excel(filePath, index=False)
[^1]: 注意,`startrow`参数指定了从哪一行开始写入,`header=False`表示不写入列名,`mode='a'`表示追加模式。
相关问题
python pandas对excel的指定第一个单元格写入内容,单元格是空的
在Python中使用Pandas操作Excel时,如果要将数据写入一个已存在的空单元格,通常先读取Excel文件,然后根据索引或标签来定位并修改特定单元格。这里是一个简单的步骤:
1. **读取Excel文件**[^1]:
```python
import pandas as pd
df = pd.read_excel('your_file.xlsx', sheet_name='Sheet1') # 假设您的文件名为your_file.xlsx,工作表名为'Sheet1'
```
2. **定位指定单元格**:
要找到第一个单元格(通常默认为A1),您可能需要知道它的位置,因为DataFrame的索引是从0开始的。比如,如果您想要写入A1,索引将是0,列名是'Column1'(假设这是第一列):
```python
row = 0
col = 'Column1'
cell_value = 'Your Data' # 您想要写入的数据
# 对应于A1的DataFrame位置是df.iloc[row, col]
```
3. **写入单元格**:
使用`to_string()`或`.loc`直接设置该位置的值,但请注意,`iloc`适用于数值索引,对于标签(如字母+数字),则使用`.loc`更为直观:
```python
if isinstance(df.index[row], str): # 判断是否为标签索引
df.loc[row, col] = cell_value
else:
df.iat[row, col] = cell_value # iat用于基于整数索引的元素访问
```
4. **写回Excel**:
```python
df.to_excel('output.xlsx', index=False, startrow=row, startcol=col) # 写回到新的Excel文件output.xlsx,startrow和startcol指定开始写入的位置
```
注意:确保路径正确,并根据实际情况调整列名、行号和数据。
python pandas写入excel单个单元格
在Python中,Pandas库提供了一个方便的功能来将数据写入Excel文件,包括单个单元格。假设你有一个DataFrame `df`,想要将某个值写入特定的单元格,比如A1,你可以这样做:
```python
import pandas as pd
# 假设你的DataFrame df有数据
data = {'Name': 'Alice', 'Age': 25}
df = pd.DataFrame(data)
# 将数据写入特定单元格
df.to_excel('output.xlsx', sheet_name='Sheet1', startrow=0, startcol=0, cellmode='overwrite') # 第0行第0列开始写入
# 如果你想更新A1单元格(第一行第一列)
cell_value = 'New Value'
df.loc[0, 'A1'] = cell_value # 使用loc按索引访问并修改特定单元格
# 然后保存整个DataFrame,如果A1已经存在,它会被覆盖
df.to_excel('output.xlsx', engine='openpyxl', index=False) # 使用openpyxl引擎避免默认的写模式冲突
阅读全文
相关推荐
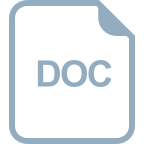
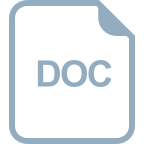
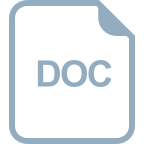













