QML如何调用setData函数更新值,完整示例
时间: 2024-02-28 16:53:23 浏览: 262
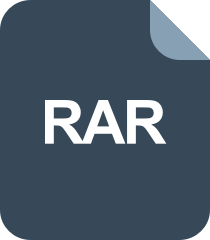
qt qml和c++的互相调用方式
以下是一个完整的示例,演示了如何在 QML 中使用自定义的 C++ 模型,并使用 `setData()` 函数来更新数据。
首先,我们需要创建一个自定义的 C++ 模型。假设我们的模型继承自 QAbstractListModel,用于存储字符串列表。
mylistmodel.h:
```
#ifndef MYLISTMODEL_H
#define MYLISTMODEL_H
#include <QAbstractListModel>
class MyListModel : public QAbstractListModel
{
Q_OBJECT
public:
explicit MyListModel(QObject *parent = nullptr);
// 重写基类虚函数
int rowCount(const QModelIndex &parent = QModelIndex()) const override;
QVariant data(const QModelIndex &index, int role = Qt::DisplayRole) const override;
bool setData(const QModelIndex &index, const QVariant &value, int role = Qt::EditRole) override;
private:
QStringList m_data;
};
#endif // MYLISTMODEL_H
```
mylistmodel.cpp:
```
#include "mylistmodel.h"
MyListModel::MyListModel(QObject *parent)
: QAbstractListModel(parent)
{
m_data << "Apple" << "Banana" << "Cherry" << "Durian";
}
int MyListModel::rowCount(const QModelIndex &parent) const
{
Q_UNUSED(parent)
return m_data.count();
}
QVariant MyListModel::data(const QModelIndex &index, int role) const
{
if (!index.isValid() || index.row() >= m_data.count())
return QVariant();
if (role == Qt::DisplayRole)
return m_data.at(index.row());
return QVariant();
}
bool MyListModel::setData(const QModelIndex &index, const QVariant &value, int role)
{
if (index.isValid() && role == Qt::EditRole) {
m_data[index.row()] = value.toString();
emit dataChanged(index, index);
return true;
}
return false;
}
```
接下来,在 main.cpp 中注册模型到 QML 上下文中:
```
#include <QGuiApplication>
#include <QQmlApplicationEngine>
#include "mylistmodel.h"
int main(int argc, char *argv[])
{
QCoreApplication::setAttribute(Qt::AA_EnableHighDpiScaling);
QGuiApplication app(argc, argv);
// 注册模型到 QML 上下文中
qmlRegisterType<MyListModel>("com.example", 1, 0, "MyListModel");
QQmlApplicationEngine engine;
const QUrl url(QStringLiteral("qrc:/main.qml"));
QObject::connect(&engine, &QQmlApplicationEngine::objectCreated,
&app, [url](QObject *obj, const QUrl &objUrl) {
if (!obj && url == objUrl)
QCoreApplication::exit(-1);
}, Qt::QueuedConnection);
engine.load(url);
return app.exec();
}
```
最后,在 QML 中使用该模型,并调用 `setData()` 函数来更新数据:
```
import QtQuick 2.14
import QtQuick.Window 2.14
import com.example 1.0
Window {
visible: true
width: 400
height: 400
MyListModel {
id: myModel
}
ListView {
anchors.fill: parent
model: myModel
delegate: Text {
text: model.display
MouseArea {
anchors.fill: parent
onClicked: {
// 调用 setData() 函数更新数据
myModel.setData(index, "new value", Qt.EditRole);
}
}
}
}
}
```
在这个示例中,我们创建了一个 ListView,使用自定义的 MyListModel 作为数据源。每个列表项都是一个 Text 控件,用于显示字符串。当用户点击某个列表项时,我们调用 `setData()` 函数来更新该项的值。注意,我们需要传递 Qt.EditRole 作为第三个参数,以指定要更新的角色。
阅读全文
相关推荐
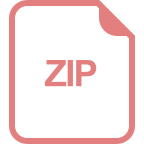
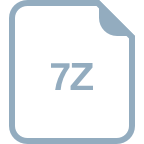






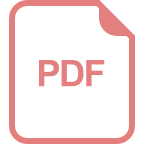
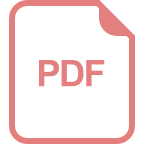
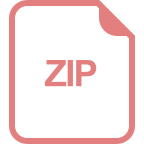
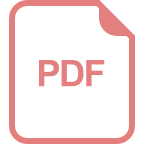
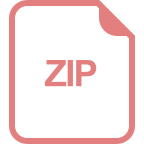
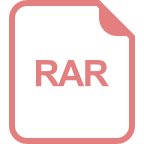
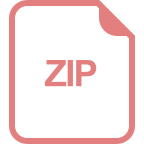