在HAL库环境下使用串口2搭建ECB02串口透传,蓝牙端发送过来的int型数据串口2接收,通过串口1打印串口2接收到的数据
时间: 2023-06-25 11:02:08 浏览: 64
实现这个功能的代码如下:
```c
#include "stm32f10x.h"
#include <stdio.h>
#define BUFFER_SIZE 100
void USART1_Init(void);
void USART2_Init(void);
void USART1_SendChar(char ch);
void USART1_SendString(char *str);
void USART2_SendChar(char ch);
void USART2_SendString(char *str);
uint8_t USART1_ReceiveData(void);
uint8_t USART2_ReceiveData(void);
int main(void)
{
uint8_t buffer[BUFFER_SIZE];
int i, data;
USART1_Init(); // 初始化串口1
USART2_Init(); // 初始化串口2
while(1)
{
// 从串口2接收数据
data = USART2_ReceiveData();
// 如果接收到的数据是整型数据
if(data >= '0' && data <= '9')
{
buffer[0] = data;
i = 1;
// 继续接收后续数据,直到接收到换行符
while(i < BUFFER_SIZE)
{
data = USART2_ReceiveData();
if(data == '\n')
{
buffer[i] = '\0'; // 字符串结尾
break;
}
buffer[i++] = data;
}
// 打印接收到的数据
USART1_SendString("Received Data: ");
USART1_SendString(buffer);
USART1_SendChar('\n');
}
}
}
void USART1_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
// 使能USART1时钟和GPIOA时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE);
// USART1 Tx(PA9)引脚配置
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// USART1 Rx(PA10)引脚配置
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// USART1配置
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Tx | USART_Mode_Rx;
USART_Init(USART1, &USART_InitStructure);
// 使能USART1
USART_Cmd(USART1, ENABLE);
}
void USART2_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
// 使能USART2时钟和GPIOA时钟
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
// USART2 Tx(PA2)引脚配置
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_2;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// USART2 Rx(PA3)引脚配置
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_3;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// USART2配置
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Tx | USART_Mode_Rx;
USART_Init(USART2, &USART_InitStructure);
// 使能USART2
USART_Cmd(USART2, ENABLE);
}
void USART1_SendChar(char ch)
{
while (USART_GetFlagStatus(USART1, USART_FLAG_TC) == RESET);
USART_SendData(USART1, ch);
}
void USART1_SendString(char *str)
{
while(*str)
{
USART1_SendChar(*str++);
}
}
void USART2_SendChar(char ch)
{
while (USART_GetFlagStatus(USART2, USART_FLAG_TC) == RESET);
USART_SendData(USART2, ch);
}
void USART2_SendString(char *str)
{
while(*str)
{
USART2_SendChar(*str++);
}
}
uint8_t USART1_ReceiveData(void)
{
while (USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == RESET);
return USART_ReceiveData(USART1);
}
uint8_t USART2_ReceiveData(void)
{
while (USART_GetFlagStatus(USART2, USART_FLAG_RXNE) == RESET);
return USART_ReceiveData(USART2);
}
```
需要注意的是,本代码使用的是串口1和串口2,如果需要使用其他串口,请根据需要进行修改。同时,本代码中假设接收到的数据都是整型数字,如果接收到其他类型的数据,需要根据具体情况进行处理。
相关推荐
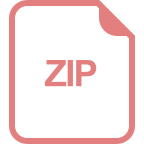














