数据结构(c语言版)任意长的整数加法问题描述:设计一个程序实现两个任意长的整数的求和运算。基本要求:利用双向循环链表,设计一个实现任意长的整数进行加法运算的演示程序。要求输入和输出每四位一组,组间用逗号隔开。如:1,0000,0000,0000,0000。
时间: 2024-03-26 12:41:40 浏览: 108
好的,这是一个关于数据结构的问题,我会尽力回答。这个问题需要使用双向循环链表来实现任意长整数的加法运算。
首先需要定义一个节点结构体,包含前驱指针prev、后继指针next和数据域data。
```c
typedef struct node {
struct node* prev;
struct node* next;
int data;
} Node;
```
然后定义链表结构体,包含头指针head和尾指针tail。
```c
typedef struct list {
Node* head;
Node* tail;
} List;
```
接下来实现链表的初始化和节点的插入操作。
```c
void initList(List* list) {
Node* node = (Node*)malloc(sizeof(Node));
node->prev = NULL;
node->next = NULL;
node->data = 0;
list->head = node;
list->tail = node;
}
void insertNode(List* list, int data) {
Node* node = (Node*)malloc(sizeof(Node));
node->prev = list->tail;
node->next = NULL;
node->data = data;
list->tail->next = node;
list->tail = node;
}
```
接下来实现任意长整数的加法操作。从两个任意长的整数的最低位开始,依次将对应位上的数相加,并将结果加到下一位上,直到处理完两个整数的所有位为止。需要注意的是,相加后要考虑进位。
```c
void add(List* list1, List* list2, List* result) {
Node* node1 = list1->tail;
Node* node2 = list2->tail;
int carry = 0;
while (node1 != list1->head || node2 != list2->head) {
int sum = node1->data + node2->data + carry;
int data = sum % 10000;
carry = sum / 10000;
insertNode(result, data);
node1 = node1->prev;
node2 = node2->prev;
}
if (carry > 0) {
insertNode(result, carry);
}
}
```
最后实现主函数,从标准输入读取两个任意长的整数,并将它们加起来输出。
```c
int main() {
List list1, list2, result;
initList(&list1);
initList(&list2);
initList(&result);
char str1[100000], str2[100000];
scanf("%s %s", str1, str2);
int len1 = strlen(str1);
int len2 = strlen(str2);
for (int i = len1 - 1; i >= 0; i -= 4) {
int data = 0, k = 1;
for (int j = 0; j < 4 && i - j >= 0; j++) {
data += (str1[i - j] - '0') * k;
k *= 10;
}
insertNode(&list1, data);
}
for (int i = len2 - 1; i >= 0; i -= 4) {
int data = 0, k = 1;
for (int j = 0; j < 4 && i - j >= 0; j++) {
data += (str2[i - j] - '0') * k;
k *= 10;
}
insertNode(&list2, data);
}
add(&list1, &list2, &result);
Node* node = result.tail;
printf("%d", node->data);
node = node->prev;
while (node != NULL) {
printf(",%04d", node->data);
node = node->prev;
}
printf("\n");
return 0;
}
```
这样就完成了任意长整数的加法运算,输入输出每四位一组,组间用逗号隔开。
阅读全文
相关推荐
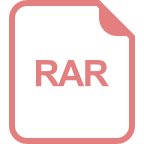
















