opencv3图像拼接_拼接图像得到全景图
时间: 2023-07-08 07:52:58 浏览: 60
要拼接图像得到全景图,可以使用OpenCV库中的函数。以下是一个简单的示例:
1. 加载两张需要拼接的图像:
```
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
```
2. 提取两张图像的特征点:
```
sift = cv2.xfeatures2d.SIFT_create()
kp1, des1 = sift.detectAndCompute(img1,None)
kp2, des2 = sift.detectAndCompute(img2,None)
```
3. 匹配特征点:
```
bf = cv2.BFMatcher()
matches = bf.knnMatch(des1,des2,k=2)
```
4. 根据匹配结果筛选出合适的特征点:
```
good = []
for m,n in matches:
if m.distance < 0.75*n.distance:
good.append(m)
```
5. 计算透视变换矩阵:
```
if len(good)>MIN_MATCH_COUNT:
src_pts = np.float32([ kp1[m.queryIdx].pt for m in good ]).reshape(-1,1,2)
dst_pts = np.float32([ kp2[m.trainIdx].pt for m in good ]).reshape(-1,1,2)
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC,5.0)
```
6. 对第二张图像进行透视变换:
```
h,w = img1.shape[:2]
img2_aligned = cv2.warpPerspective(img2, M, (w*2,h))
```
7. 将两张图像拼接在一起:
```
result = np.zeros((h,w*2,3), np.uint8)
result[0:h, 0:w] = img1
result[0:h, w:w*2] = img2_aligned[:,w//2:w*3//2]
```
8. 显示结果:
```
cv2.imshow("Result", result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这样就可以拼接两张图像得到全景图了。当然,这只是一个简单的示例,实际上还需要考虑很多细节问题,如图像的对齐、边缘处理等等。
相关推荐
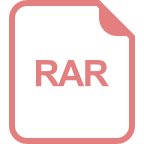
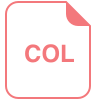
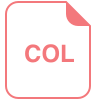

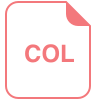
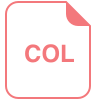









