c语言用openssl
时间: 2023-10-24 10:09:06 浏览: 37
OpenSSL是一个开源的加密库,可以在C语言中使用它来实现各种加密算法,如SSL、TLS、RSA、AES等。
以下是一个使用OpenSSL库在C语言中进行AES加密和解密的示例代码:
```c
#include <openssl/aes.h>
#include <stdio.h>
#include <string.h>
int main(int argc, char **argv)
{
AES_KEY aes_key;
unsigned char key[] = "0123456789abcdef"; // 16字节的AES加密密钥
unsigned char iv[] = "1234567890abcdef"; // 16字节的初始化向量
unsigned char plaintext[128] = "hello world!"; // 待加密的明文
unsigned char ciphertext[128]; // 存储加密后的密文
unsigned char decrypted[128]; // 存储解密后的明文
int plaintext_len = strlen(plaintext); // 明文长度
// 初始化AES密钥
if (AES_set_encrypt_key(key, 128, &aes_key) < 0) {
printf("Error: failed to set encryption key\n");
return -1;
}
// 进行AES加密
AES_cbc_encrypt(plaintext, ciphertext, plaintext_len, &aes_key, iv, AES_ENCRYPT);
printf("Ciphertext: %s\n", ciphertext);
// 初始化AES密钥
if (AES_set_decrypt_key(key, 128, &aes_key) < 0) {
printf("Error: failed to set decryption key\n");
return -1;
}
// 进行AES解密
AES_cbc_encrypt(ciphertext, decrypted, plaintext_len, &aes_key, iv, AES_DECRYPT);
printf("Decrypted text: %s\n", decrypted);
return 0;
}
```
注意:本代码示例只是为了演示如何使用OpenSSL库进行AES加密和解密,实际使用时需要注意安全性和效率问题。
相关推荐
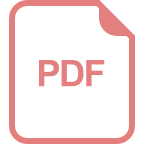
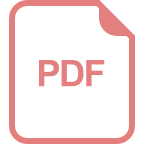
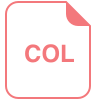
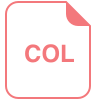
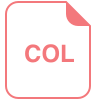
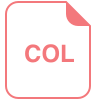
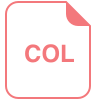









