c语言雷霆战机小游戏代码加解释
时间: 2023-10-17 15:29:07 浏览: 63
好的,下面是C语言雷霆战机小游戏的代码及其解释:
```c
#include<stdio.h>
#include<stdlib.h>
#include<conio.h>
#include<windows.h>
#include<time.h>
#define WIDTH 80 // 游戏区域宽度
#define HEIGHT 30 // 游戏区域高度
#define MAX_ENEMY_NUM 5 // 最大敌机数量
#define BULLET_SPEED 2 // 子弹速度
#define ENEMY_SPEED 1 // 敌机速度
#define FPS 30 // 游戏帧率
#define SCORE_PER_ENEMY 10 // 消灭一个敌机的得分
int score = 0; // 玩家得分
int level = 1; // 玩家关卡
int player_x = WIDTH/2; // 玩家飞机的x坐标
int player_y = HEIGHT-2; // 玩家飞机的y坐标
int bullet_x[HEIGHT]; // 子弹的x坐标
int bullet_y[HEIGHT]; // 子弹的y坐标
int bullet_num = 0; // 当前子弹数量
int enemy_x[MAX_ENEMY_NUM]; // 敌机的x坐标
int enemy_y[MAX_ENEMY_NUM]; // 敌机的y坐标
int enemy_num = 0; // 当前敌机数量
void gotoxy(int x, int y) // 光标移动函数
{
COORD pos;
HANDLE hOutput;
pos.X = x;
pos.Y = y;
hOutput = GetStdHandle(STD_OUTPUT_HANDLE);
SetConsoleCursorPosition(hOutput, pos);
}
void hidecursor() // 隐藏光标函数
{
CONSOLE_CURSOR_INFO cursor_info = { 1, 0 };
SetConsoleCursorInfo(GetStdHandle(STD_OUTPUT_HANDLE), &cursor_info);
}
void draw_player() // 绘制玩家飞机函数
{
gotoxy(player_x, player_y);
printf("^");
}
void draw_bullet(int i) // 绘制子弹函数
{
gotoxy(bullet_x[i], bullet_y[i]);
printf("|");
}
void draw_enemy(int i) // 绘制敌机函数
{
gotoxy(enemy_x[i], enemy_y[i]);
printf("v");
}
void update_bullet() // 更新子弹位置函数
{
for(int i=0; i<bullet_num; i++)
{
bullet_y[i] -= BULLET_SPEED;
if(bullet_y[i] <= 0)
{
for(int j=i; j<bullet_num-1; j++)
{
bullet_x[j] = bullet_x[j+1];
bullet_y[j] = bullet_y[j+1];
}
bullet_num--;
i--;
}
}
}
void update_enemy() // 更新敌机位置函数
{
for(int i=0; i<enemy_num; i++)
{
enemy_y[i] += ENEMY_SPEED;
if(enemy_y[i] >= HEIGHT-1)
{
for(int j=i; j<enemy_num-1; j++)
{
enemy_x[j] = enemy_x[j+1];
enemy_y[j] = enemy_y[j+1];
}
enemy_num--;
i--;
}
}
}
void draw_score() // 绘制得分函数
{
gotoxy(0, HEIGHT);
printf("Score: %d", score);
}
void draw_level() // 绘制关卡函数
{
gotoxy(WIDTH-10, HEIGHT);
printf("Level: %d", level);
}
void draw_gameover() // 绘制游戏结束函数
{
gotoxy(WIDTH/2-5, HEIGHT/2);
printf("Game Over!");
}
void generate_enemy() // 生成敌机函数
{
if(enemy_num < MAX_ENEMY_NUM)
{
enemy_x[enemy_num] = rand() % WIDTH;
enemy_y[enemy_num] = 0;
enemy_num++;
}
}
void check_collision() // 检查碰撞函数
{
for(int i=0; i<bullet_num; i++)
{
for(int j=0; j<enemy_num; j++)
{
if(bullet_x[i] == enemy_x[j] && bullet_y[i] == enemy_y[j])
{
score += SCORE_PER_ENEMY;
for(int k=i; k<bullet_num-1; k++)
{
bullet_x[k] = bullet_x[k+1];
bullet_y[k] = bullet_y[k+1];
}
bullet_num--;
for(int k=j; k<enemy_num-1; k++)
{
enemy_x[k] = enemy_x[k+1];
enemy_y[k] = enemy_y[k+1];
}
enemy_num--;
}
}
}
for(int i=0; i<enemy_num; i++)
{
if(enemy_x[i] == player_x && enemy_y[i] == player_y)
{
draw_gameover();
exit(0);
}
}
}
void game_loop() // 游戏循环函数
{
while(1)
{
system("cls");
draw_player();
for(int i=0; i<bullet_num; i++)
{
draw_bullet(i);
}
for(int i=0; i<enemy_num; i++)
{
draw_enemy(i);
}
draw_score();
draw_level();
update_bullet();
update_enemy();
check_collision();
if(rand() % 100 < level)
{
generate_enemy();
}
if(kbhit())
{
char c = getch();
if(c == 'a' && player_x > 0)
{
player_x--;
}
else if(c == 'd' && player_x < WIDTH-1)
{
player_x++;
}
else if(c == ' ')
{
bullet_x[bullet_num] = player_x;
bullet_y[bullet_num] = player_y-1;
bullet_num++;
}
}
Sleep(1000/FPS);
}
}
int main()
{
srand(time(NULL));
hidecursor();
game_loop();
return 0;
}
```
以上是C语言雷霆战机小游戏的代码及其解释,希望能够帮助您了解该游戏的实现和原理。
相关推荐
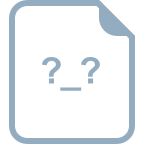
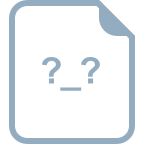















