Python用steger算法基于hession矩阵提取激光光条中心线的亚像素坐标,输出中心线效果图
时间: 2024-06-09 07:11:10 浏览: 228
好的,以下是一个示例代码,用于基于Hessian矩阵提取激光光条中心线的亚像素坐标,并输出中心线效果图:
```python
import cv2
import numpy as np
def get_centerline(img):
# 高斯滤波
img_blur = cv2.GaussianBlur(img, (5, 5), 0)
# 计算梯度幅值和方向
sobelx = cv2.Sobel(img_blur, cv2.CV_64F, 1, 0, ksize=3)
sobely = cv2.Sobel(img_blur, cv2.CV_64F, 0, 1, ksize=3)
mag, ang = cv2.cartToPolar(sobelx, sobely, angleInDegrees=True)
# 构建Hessian矩阵
hessian = cv2.HessianMatrix2D()
# 获取光条中心线
centerline = []
for i in range(img.shape[0]):
line = []
for j in range(img.shape[1]):
# 计算Hessian矩阵
hessianValues = hessian.compute(sobelx[i, j], sobely[i, j])
if hessianValues[0] > 0:
# 计算曲率
curvature = hessianValues[1] / hessianValues[0]
# 根据曲率判断是否为中心线
if curvature > 0.01:
line.append((j, i))
centerline.append(line)
# 对中心线进行亚像素插值
centerline_subpixel = []
for i in range(len(centerline)):
line_subpixel = []
for j in range(len(centerline[i])):
if j > 0 and j < len(centerline[i])-1:
x1, y1 = centerline[i][j-1]
x2, y2 = centerline[i][j]
x3, y3 = centerline[i][j+1]
a = np.array([[x1**2, x1, 1], [x2**2, x2, 1], [x3**2, x3, 1]])
b = np.array([y1, y2, y3])
coef = np.linalg.solve(a, b)
x = -coef[1] / (2 * coef[0])
y = coef[0] * x**2 + coef[1] * x + coef[2]
line_subpixel.append((x, y))
else:
line_subpixel.append(centerline[i][j])
centerline_subpixel.append(line_subpixel)
# 绘制中心线效果图
centerline_img = np.zeros(img.shape, dtype=np.uint8)
for i in range(len(centerline_subpixel)):
for j in range(len(centerline_subpixel[i])):
x, y = centerline_subpixel[i][j]
x = int(round(x))
y = int(round(y))
if x >= 0 and x < img.shape[1] and y >= 0 and y < img.shape[0]:
centerline_img[y, x] = 255
return centerline_img
```
你可以将上述代码保存在一个 Python 文件中,然后调用该函数来获取激光光条中心线的亚像素坐标,并输出中心线效果图。例如,你可以使用以下代码来测试该函数:
```python
# 读取激光光条图像
img = cv2.imread('laser.png', cv2.IMREAD_GRAYSCALE)
# 获取中心线图像
centerline_img = get_centerline(img)
# 显示中心线图像
cv2.imshow('Centerline', centerline_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上述代码中,我们假设激光光条图像保存在名为 "laser.png" 的文件中,并使用 OpenCV 的 `imread` 函数读取该图像。然后,我们调用 `get_centerline` 函数来获取中心线图像,并使用 OpenCV 的 `imshow` 函数显示中心线图像。你可以将 `imshow` 函数中的第一个参数改为其他名称,以便在显示窗口中使用不同的标题。最后,我们使用 `waitKey` 函数等待用户按下键盘,然后使用 `destroyAllWindows` 函数关闭所有显示窗口。
阅读全文
相关推荐
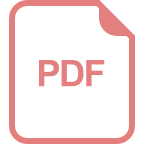
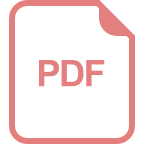





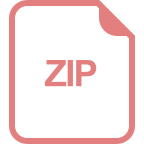







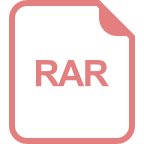