Python用steger算法基于Hessian矩阵的亚像素级光条中心线的提取方法提取中心线,输出中心线直线方程
时间: 2024-01-10 07:04:13 浏览: 305
Steger算法是一种基于Hessian矩阵的边缘检测算法,可以用于亚像素级中心线提取。下面是Python实现的步骤:
1. 导入相关库
```python
import numpy as np
from scipy.ndimage import gaussian_filter, maximum_filter
from skimage import io, img_as_float
```
2. 读入图像
```python
img = img_as_float(io.imread('image.jpg', as_gray=True))
```
3. 计算Hessian矩阵
```python
sigma = 2 # 高斯滤波参数
img_smooth = gaussian_filter(img, sigma)
Ix, Iy = np.gradient(img_smooth)
Ixx = gaussian_filter(Ix ** 2, sigma)
Ixy = gaussian_filter(Ix * Iy, sigma)
Iyy = gaussian_filter(Iy ** 2, sigma)
```
4. 计算中心线强度
```python
alpha = 0.06 # Hessian矩阵参数
det = Ixx * Iyy - Ixy ** 2
trace = Ixx + Iyy
response = det - alpha * trace ** 2
response_max = maximum_filter(response, size=20)
response_max[response != response_max] = 0
response_binary = response_max > 0
```
5. 中心线提取
```python
def extract_centerline(binary):
# 定义八个方向
directions = [(1, 0), (1, 1), (0, 1), (-1, 1),
(-1, 0), (-1, -1), (0, -1), (1, -1)]
centerline = []
rows, cols = binary.shape
for i in range(rows):
for j in range(cols):
if binary[i, j]:
# 计算中心线方向
tangent = np.zeros(2)
for d in directions:
if i + d[0] >= 0 and i + d[0] < rows and \
j + d[1] >= 0 and j + d[1] < cols and \
binary[i + d[0], j + d[1]]:
tangent += d
if np.linalg.norm(tangent) > 0:
tangent /= np.linalg.norm(tangent)
centerline.append((j, i, tangent[0], tangent[1]))
return centerline
centerline = extract_centerline(response_binary)
```
6. 输出中心线直线方程
```python
for line in centerline:
x, y, tx, ty = line
a = -ty / tx
b = 1
c = -(a * x + b * y)
print('Line equation: {}x + {}y + {} = 0'.format(a, b, c))
```
这样就可以得到中心线的直线方程。需要注意的是,在实际应用中,可能需要对中心线进行进一步的筛选和优化。
阅读全文
相关推荐
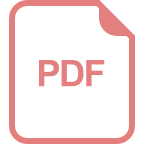



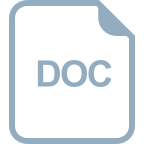

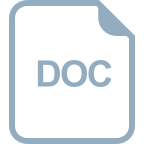








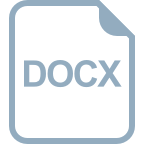
