c语言,输入两个正整数m和n,求其最大公约数和最小公倍数。
时间: 2024-11-20 07:38:39 浏览: 27
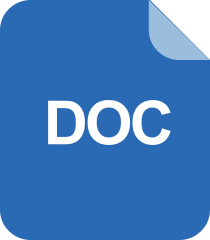
大学经典C语言编程试题.doc
在C语言中,求两个正整数的最大公约数(Greatest Common Divisor, GCD)和最小公倍数(Least Common Multiple, LCM)可以通过欧几里得算法(Euclidean Algorithm)来实现。下面是一个简单的示例程序:
首先,我们需要编写一个函数来计算GCD:
```c
#include <stdio.h>
// Euclidean algorithm to find GCD
int gcd(int a, int b) {
if (b == 0) return a;
return gcd(b, a % b);
}
```
接着,我们可以利用GCD来计算LCM,因为LCM(a, b) = |a * b| / GCD(a, b):
```c
int lcm(int a, int b) {
int temp = gcd(a, b);
return (a * b) / temp; // Since we know that a*b is positive, we don't need the absolute value
}
int main() {
int m, n;
printf("Enter two positive integers: ");
scanf("%d %d", &m, &n);
if (m <= 0 || n <= 0) {
printf("Input must be positive integers.\n");
return 1;
}
int result_gcd = gcd(m, n);
int result_lcm = lcm(m, n);
printf("The GCD of %d and %d is: %d\n", m, n, result_gcd);
printf("The LCM of %d and %d is: %d\n", m, n, result_lcm);
return 0;
}
```
在这个程序中,用户会被提示输入两个正整数,然后程序会计算它们的最大公约数和最小公倍数,并输出结果。
阅读全文
相关推荐
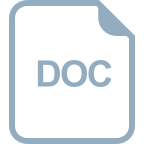
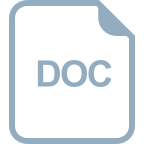















