在Linux环境下,使用消息队列实现客户端-服务器应用程序,完成文件传输功能。请详细编写程序
时间: 2023-08-31 09:24:20 浏览: 236
### 回答1:
在Linux环境下,可以使用消息队列来实现客户端-服务器应用程序,完成文件传输功能。程序代码如下:// 服务器端:
#include <stdio.h>
#include <stdlib.h>
#include <sys/msg.h>
#define MAX_LEN 128
struct my_msgbuf {
long mtype;
char mtext[MAX_LEN];
};
int main(void)
{
struct my_msgbuf buf;
int msqid;
key_t key;
// 获取消息队列的key
if ((key = ftok("server.c", 'B')) == -1) {
perror("ftok");
exit(1);
}
// 创建消息队列
if ((msqid = msgget(key, 0644 | IPC_CREAT)) == -1) {
perror("msgget");
exit(1);
}
printf("Server: ready to receive messages, boss.\n");
// 接收消息
for(;;) {
if (msgrcv(msqid, &buf, sizeof(buf.mtext), 0, 0) == -1) {
perror("msgrcv");
exit(1);
}
printf("Server: \"%s\"\n", buf.mtext);
}
return 0;
}
// 客户端:
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MAX_LEN 128
struct my_msgbuf {
long mtype;
char mtext[MAX_LEN];
};
int main(void)
{
struct my_msgbuf buf;
int msqid;
key_t key;
// 获取消息队列的key
if ((key = ftok("server.c", 'B')) == -1) {
perror("ftok");
exit(1);
}
// 连接消息队列
if ((msqid = msgget(key, 0644)) == -1) {
perror("msgget");
exit(1);
}
// 发送消息
printf("Client: Enter lines of text, ^D to quit:\n");
buf.mtype = 1;
while(fgets(buf.mtext, MAX_LEN, stdin) != NULL) {
int len = strlen(buf.mtext);
if (buf.mtext[len-1] == '\n') buf.mtext[len-1] = '\0';
if (msgsnd(msqid, &buf, len+1, 0) == -1) /* +1 for '\0' */
perror("msgsnd");
}
if (msgctl(msqid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(1);
}
return 0;
}
### 回答2:
在Linux环境下,可以使用消息队列来实现客户端-服务器应用程序,完成文件传输功能。下面是一个基于C语言的示例代码:
服务端代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#include <string.h>
#define MAX_SIZE 1024
typedef struct {
long mtype;
char mtext[MAX_SIZE];
} msgbuf;
int main() {
key_t key;
int msgid;
msgbuf msg;
// 生成一个唯一的key值
key = ftok(".", 'a');
if ((msgid = msgget(key, IPC_CREAT | 0666)) == -1) {
perror("msgget");
exit(1);
}
printf("服务端启动\n");
// 从消息队列中读取数据
while (1) {
if (msgrcv(msgid, &msg, sizeof(msg.mtext), 1, 0) == -1) {
perror("msgrcv");
exit(1);
}
// 获取文件名
char filename[MAX_SIZE];
strcpy(filename, msg.mtext);
printf("接收到文件:%s\n", filename);
// 打开文件
FILE* file = fopen(filename, "wb");
if (file == NULL) {
perror("fopen");
exit(1);
}
// 从消息队列中读取文件内容,直到读取到结束标志为止
while (1) {
if (msgrcv(msgid, &msg, sizeof(msg.mtext), 1, 0) == -1) {
perror("msgrcv");
exit(1);
}
if (strcmp(msg.mtext, "结束") == 0) {
break;
}
// 写入文件
fwrite(msg.mtext, sizeof(char), strlen(msg.mtext), file);
}
// 关闭文件
fclose(file);
printf("接收完成\n");
}
return 0;
}
```
客户端代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#include <string.h>
#define MAX_SIZE 1024
typedef struct {
long mtype;
char mtext[MAX_SIZE];
} msgbuf;
int main() {
key_t key;
int msgid;
msgbuf msg;
// 生成一个唯一的key值
key = ftok(".", 'a');
if ((msgid = msgget(key, IPC_CREAT | 0666)) == -1) {
perror("msgget");
exit(1);
}
printf("客户端启动\n");
// 输入文件名
printf("请输入要传输的文件名:");
fgets(msg.mtext, MAX_SIZE, stdin);
msg.mtext[strcspn(msg.mtext, "\n")] = '\0';
// 发送文件名到消息队列
msg.mtype = 1;
if (msgsnd(msgid, &msg, strlen(msg.mtext) + 1, 0) == -1) {
perror("msgsnd");
exit(1);
}
// 打开文件
FILE* file = fopen(msg.mtext, "rb");
if (file == NULL) {
perror("fopen");
exit(1);
}
// 读取文件内容并发送到消息队列
while (1) {
// 读取文件内容
size_t len = fread(msg.mtext, sizeof(char), MAX_SIZE, file);
// 发送文件内容到消息队列
msg.mtype = 1;
if (msgsnd(msgid, &msg, len, 0) == -1) {
perror("msgsnd");
exit(1);
}
if (feof(file)) {
break;
}
}
// 发送结束标志到消息队列
strcpy(msg.mtext, "结束");
msg.mtype = 1;
if (msgsnd(msgid, &msg, strlen(msg.mtext) + 1, 0) == -1) {
perror("msgsnd");
exit(1);
}
// 关闭文件
fclose(file);
printf("发送完成\n");
return 0;
}
```
以上代码实现了一个简单的客户端-服务器应用程序,可以通过消息队列在服务端和客户端之间传输文件。服务端通过消息队列接收客户端发送的文件,并将文件内容保存到指定文件中。客户端通过消息队列将文件内容逐块发送给服务端。在发送文件结束时,客户端发送一个特定的结束标志给服务端,以告知文件已发送完毕。服务端接收到结束标志后,结束文件接收过程。
### 回答3:
在Linux环境下,可以使用消息队列实现客户端-服务器应用程序,完成文件传输功能。
首先,需要创建一个服务器程序和一个客户端程序。
服务器程序的主要功能是监听客户端的请求,并根据请求进行相应的文件传输操作。以下是服务器程序的伪代码:
1. 引入所需的头文件和定义所需的常量和变量。
2. 创建消息队列,用于接收客户端发送的请求。
3. 进入无限循环等待客户端请求的过程。
4. 当接收到请求时,根据请求类型执行相应的操作:
a. 如果是上传文件请求,则接收文件名和文件内容,并将文件内容写入服务器端的指定文件中。
b. 如果是下载文件请求,则读取服务器端指定文件的内容,并将内容发送给客户端。
c. 如果是退出请求,则结束循环并关闭消息队列。
5. 关闭消息队列。
客户端程序的主要功能是向服务器发送请求,并接收服务器返回的文件内容。以下是客户端程序的伪代码:
1. 引入所需的头文件和定义所需的常量和变量。
2. 获取用户输入的命令和文件名。
3. 连接服务器的消息队列。
4. 根据用户输入的命令类型构造请求内容,并将请求内容发送给服务器。
5. 根据请求类型执行相应操作:
a. 如果是上传文件请求,则读取指定文件的内容,并将内容发送给服务器。
b. 如果是下载文件请求,则接收服务器发送的文件内容,并将内容写入本地文件中。
6. 关闭消息队列。
以上伪代码仅为演示目的,实际编写程序时需要根据具体需求做相应的修改和完善。实现客户端-服务器文件传输功能的核心是通过消息队列进行通信,用户可以根据具体需求进行扩展,如添加验证机制、错误处理等。
阅读全文
相关推荐
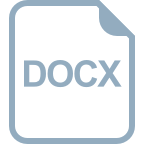
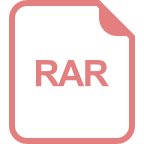
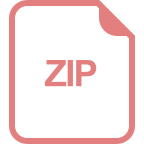











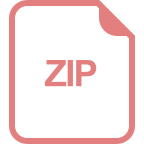
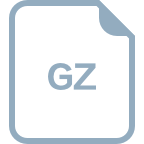
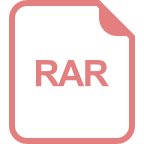
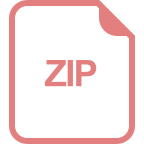