void FIFO_Agorithm() { int n, len, * save_Frame = NULL, * interview_Array = NULL; Init(&n, &len, save_Frame, interview_Array); //初始化Init 函数 int* in_HereTime = new int[n]; //f for (int i = 0; i < n; i++) in_HereTime[i] = 0; // 初始化都为零 int addr; int cnt = 0; int score = 0; int fail_time = 0; int iter = 0; while (iter < len) { cout << endl << "第" << iter << "轮:"; addr = interview_Array[iter]; iter++; if (cnt < n) { if (Find_Exist(save_Frame, cnt, addr) != -1) { score++; cout << "\"" << addr << "\" 被命中了\t\t------->"; Print_Frame(save_Frame, n); Update_InHereTime(in_HereTime, cnt, -1); } else // 未命中,但有空间 { fail_time++; cout << "未命中," << "\"" << addr << "\" 被装入 \t------->"; save_Frame[cnt] = addr; Print_Frame(save_Frame, n); Update_InHereTime(in_HereTime, cnt, cnt); cnt++; } } else { if (Find_Exist(save_Frame, n, addr) != -1) { score++; cout << "\"" << addr << "\" 被命中了\t\t------->"; Print_Frame(save_Frame, n); Update_InHereTime(in_HereTime, n, -1); } else // 未命中,但没了空间 { fail_time++; int max_Time = 0; int index; for (int i = 0; i < n; i++) { if (in_HereTime[i] > max_Time) { max_Time = in_HereTime[i]; index = i; } } cout << "\"" << addr << "\" 替换了 \"" << save_Frame[index] << "\"\t\t------->"; save_Frame[index] = addr; Print_Frame(save_Frame, n); int ind = Find_Exist(save_Frame, n, addr); Update_InHereTime(in_HereTime, n, ind); } } } cout << endl; cout << "缺页次数为:" << fail_time << endl; cout << "缺页中断率 R = " << Page_Loss_Rate(score, fail_time) << "%" << endl; delete[] save_Frame; delete[] interview_Array; delete[] in_HereTime; return; }
时间: 2024-03-04 17:52:52 浏览: 18
这段代码实现了FIFO(First In First Out)页面置换算法。该算法的思想是,将最先进入内存的页面置换出去,即按照页面进入内存的顺序进行置换。该算法使用了一个保存当前内存中页面的数组save_Frame和一个保存各页面进入内存时间的数组in_HereTime。程序首先通过Init函数进行初始化,然后使用动态内存分配创建一个大小为n的整型数组in_HereTime,并将所有元素初始化为0。程序每次获取一个页面地址,如果当前内存中还有空间,则判断该页面是否已经在内存中,如果在则命中,否则将该页面装入内存中。如果内存已经满了,则需要进行页面置换,找到在内存中已经存在时间最长的页面进行置换。程序最后输出缺页次数和缺页中断率。
需要注意的是,在使用动态内存分配时,需要及时释放内存,避免内存泄漏的问题。程序最后使用delete[]语句释放动态分配的内存。
相关问题
using namespace std; void OPT_Agorithm(); void FIFO_Agorithm(); void LRU_Agorithm(); void LFU_Agorithm(); double Page_Loss_Rate(int, int); int Find_Exist(int*, int, int); int Find_LeastInteviewTime(int, int, int*, int); void Update_InHereTime(int*, int, int); int Find_LeastNotUseTime(int, int, int*); int Find_LeastNotInterviewTime(int, int*); void Print_Frame(int*, int); void Print_Menu();
这段代码定义了一些函数的声明,这些函数包括:
1. OPT_Agorithm:用于实现OPT算法的函数。
2. FIFO_Agorithm:用于实现FIFO算法的函数。
3. LRU_Agorithm:用于实现LRU算法的函数。
4. LFU_Agorithm:用于实现LFU算法的函数。
5. Page_Loss_Rate:用于计算页面失效率的函数,需要传入已经失效的页面数和页面请求数。
6. Find_Exist:用于查找指定页面是否在指定数组中存在,如果存在返回其下标,否则返回-1。
7. Find_LeastInteviewTime:用于查找指定页面在指定页面序列中最后一次被访问的时间,返回其下标。
8. Update_InHereTime:用于更新指定页面在指定数组中的最后一次被访问时间。
9. Find_LeastNotUseTime:用于查找指定页面在指定物理块中最长时间没有被使用的时间,返回其下标。
10. Find_LeastNotInterviewTime:用于查找指定页面在指定页面序列中最长时间没有被访问的时间,返回其下标。
11. Print_Frame:用于打印指定长度的整型数组的函数。
12. Print_Menu:用于打印菜单的函数。
uint32 bluetooth_ch9141_read_buff (uint8 *buff, uint32 len) { uint32 data_len = len; fifo_read_buffer(&bluetooth_ch9141_fifo, buff, &data_len, FIFO_READ_AND_CLEAN); return data_len; } 将该代码改为读取数字
uint32 bluetooth_ch9141_read_buff (uint32 *buff, uint32 len)
{
uint32 data_len = len * sizeof(uint32);
fifo_read_buffer(&bluetooth_ch9141_fifo, (uint8*)buff, &data_len, FIFO_READ_AND_CLEAN);
return data_len / sizeof(uint32);
}
在修改后的代码中,我们将输入缓冲区的类型从 uint8* 更改为 uint32*,以便读取数字。同时,我们还将 data_len 的单位从字节更改为 uint32 的数量,这样它将表示读取的数字数量。最后,我们需要将 buff 强制转换为 uint8* 类型,以便与 fifo_read_buffer 函数的参数类型匹配。
相关推荐
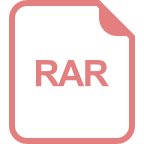
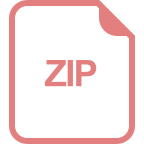












