uint32 bluetooth_ch9141_read_buff (uint8 *buff, uint32 len) { uint32 data_len = len; fifo_read_buffer(&bluetooth_ch9141_fifo, buff, &data_len, FIFO_READ_AND_CLEAN); return data_len; }uint32 bluetooth_ch9141_send_buff (uint8 *buff, uint32 len) { uint16 time_count = 0; while(len > 30) { time_count = 0; while(BLUETOOTH_CH9141_RTS_PIN && time_count++ < BLUETOOTH_CH9141_TIMEOUT_COUNT) // 如果RTS为低电平,则继续发送数据 delay_ms(1); if(time_count >= BLUETOOTH_CH9141_TIMEOUT_COUNT) return len; // 模块忙,如果允许当前程序使用while等待 则可以使用后面注释的while等待语句替换本if语句 uart_putbuff(BLUETOOTH_CH9141_INDEX, buff, 30); buff += 30; // 地址偏移 len -= 30; // 数量 } time_count = 0; while(BLUETOOTH_CH9141_RTS_PIN && time_count++ < BLUETOOTH_CH9141_TIMEOUT_COUNT) // 如果RTS为低电平,则继续发送数据 delay_ms(1); if(time_count >= BLUETOOTH_CH9141_TIMEOUT_COUNT) return len; // 模块忙,如果允许当前程序使用while等待 则可以使用后面注释的while等待语句替换本if语句 uart_putbuff(BLUETOOTH_CH9141_INDEX, buff, (uint16)len); // 发送最后的数据 return 0; }uint8 bluetooth_ch9141_init (void) { wireless_type = WIRELESS_CH9141; // 本函数使用的波特率为115200 为蓝牙转串口模块的默认波特率 如需其他波特率请使用上位机修改模块参数 fifo_init(&bluetooth_ch9141_fifo, bluetooth_ch9141_buffer, BLUETOOTH_CH9141_BUFFER_SIZE); uart_init(BLUETOOTH_CH9141_INDEX, BLUETOOTH_CH9141_TX_PIN, BLUETOOTH_CH9141_RX_PIN, BLUETOOTH_CH9141_BUAD_RATE, BLUETOOTH_CH9141_TIMER); return 0; } void bluetooth_ch9141_uart_callback (void) { // 读取无线串口的数据 并且置位接收标志 bluetooth_ch9141_data = BLUETOOTH_CH9141_DATA_BUF; fifo_write_buffer(&bluetooth_ch9141_fifo, &bluetooth_ch9141_data, 1); // 存入 FIFO } static fifo_struct bluetooth_ch9141_fifo; static uint8 bluetooth_ch9141_buffer[BLUETOOTH_CH9141_BUFFER_SIZE]; // 数据存放数组 static uint8 bluetooth_ch9141_data;将以上代码改写一个适合于HC-05的蓝牙代码基于stc3
时间: 2024-04-16 11:23:58 浏览: 12
以下是基于 STC3 的 HC-05 蓝牙模块的修改后代码示例:
```c
#include <reg51.h>
#define BLUETOOTH_HC05_BUFFER_SIZE 64
#define BLUETOOTH_HC05_TIMEOUT_COUNT 1000
typedef struct {
uint8_t buffer[BLUETOOTH_HC05_BUFFER_SIZE];
uint8_t head;
uint8_t tail;
} fifo_struct;
fifo_struct bluetooth_hc05_fifo;
void fifo_init(fifo_struct *fifo)
{
fifo->head = 0;
fifo->tail = 0;
}
void fifo_write_buffer(fifo_struct *fifo, uint8_t *data, uint8_t len)
{
uint8_t i;
for (i = 0; i < len; i++) {
fifo->buffer[fifo->head] = data[i];
fifo->head = (fifo->head + 1) % BLUETOOTH_HC05_BUFFER_SIZE;
}
}
uint8_t fifo_read_buffer(fifo_struct *fifo, uint8_t *data, uint8_t *len)
{
uint8_t i;
for (i = 0; i < *len; i++) {
if (fifo->head == fifo->tail) {
// Buffer is empty
*len = i;
return 1;
}
data[i] = fifo->buffer[fifo->tail];
fifo->tail = (fifo->tail + 1) % BLUETOOTH_HC05_BUFFER_SIZE;
}
return 0;
}
void delay_ms(uint16_t ms)
{
uint16_t i, j;
for (i = 0; i < ms; i++) {
for (j = 0; j < 120; j++) {
// Delay approximately 1ms
}
}
}
void uart_init(uint32_t buad_rate)
{
// UART initialization code for STC3
// Set UART baud rate and other configuration
}
void uart_putbuff(uint8_t *buff, uint8_t len)
{
// UART send buffer code for STC3
// Send the buffer over UART
}
void uart_getbuff(uint8_t *buff, uint8_t len)
{
// UART receive buffer code for STC3
// Receive data into the buffer over UART
}
uint32_t bluetooth_hc05_read_buff(uint8_t *buff, uint32_t len)
{
uint32_t data_len = len;
fifo_read_buffer(&bluetooth_hc05_fifo, buff, &data_len);
return data_len;
}
uint32_t bluetooth_hc05_send_buff(uint8_t *buff, uint32_t len)
{
uint16_t time_count = 0;
while (len > 30) {
time_count = 0;
while (time_count++ < BLUETOOTH_HC05_TIMEOUT_COUNT && BLUETOOTH_HC05_RTS_PIN) {
delay_ms(1);
}
if (time_count >= BLUETOOTH_HC05_TIMEOUT_COUNT) {
return len; // 模块忙,如果允许当前程序使用 while 等待则可以使用后面注释的 while 等待语句替换本 if 语句
}
uart_putbuff(buff, 30);
buff += 30; // 地址偏移
len -= 30; // 数量
}
time_count = 0;
while (time_count++ < BLUETOOTH_HC05_TIMEOUT_COUNT && BLUETOOTH_HC05_RTS_PIN) {
delay_ms(1);
}
if (time_count >= BLUETOOTH_HC05_TIMEOUT_COUNT) {
return len; // 模块忙,如果允许当前程序使用 while 等待则可以使用后面注释的 while 等待语句替换本 if 语句
}
uart_putbuff(buff, (uint16_t)len); // 发送最后的数据
return 0;
}
uint8_t bluetooth_hc05_init(void)
{
// Initialization code for HC-05 on STC3
fifo_init(&bluetooth_hc05_fifo);
uart_init(BLUETOOTH_HC05_BUAD_RATE);
return 0;
}
void bluetooth_hc05_uart_callback(void)
{
uint8_t data = uart_getbuff();
fifo_write_buffer(&bluetooth_hc05_fifo, &data, 1); // 存入 FIFO
}
uint8_t bluetooth_hc05_data;
void main(void)
{
bluetooth_hc05_init();
while (1) {
// Main program loop
// Read and process data from the FIFO buffer
while (bluetooth_hc05_data = fifo_read_buffer(&bluetooth_hc05_fifo)) {
// Process data
}
}
}
```
请注意,上述代码仅为示例,具体的引脚定义和常量值需要根据你的硬件和需求进行修改。另外,需要根据实际情况修改函数中的 UART 初始化、发送和接收函数,以适配 STC3 的硬件平台。
相关推荐
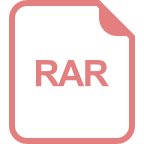
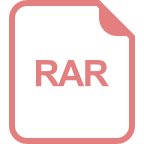















