帮我写一段unity2d中跳跃的代码
时间: 2023-03-30 10:03:42 浏览: 221
好的,以下是一段unity2d中跳跃的代码:
```
public class PlayerController : MonoBehaviour
{
public float jumpForce = 10f;
public float groundCheckRadius = .2f;
public LayerMask groundLayer;
public Transform groundCheck;
private Rigidbody2D rb;
private bool isGrounded;
void Start()
{
rb = GetComponent<Rigidbody2D>();
}
void FixedUpdate()
{
isGrounded = Physics2D.OverlapCircle(groundCheck.position, groundCheckRadius, groundLayer);
}
void Update()
{
if (isGrounded && Input.GetKeyDown(KeyCode.Space))
{
rb.velocity = new Vector2(rb.velocity.x, jumpForce);
}
}
}
```
这段代码实现了当玩家在地面上并按下空格键时,玩家会跳起来。
相关问题
写一段Unity 类似马里奥的2d移动跳跃代码
以下是一个简单的Unity类似马里奥的2D移动跳跃代码示例:
```csharp
public class PlayerController : MonoBehaviour
{
public float speed = 5.0f; // 玩家移动速度
public float jumpForce = 10.0f; // 玩家跳跃力度
public LayerMask groundLayer; // 用于检测地面的层级
private Rigidbody2D rb;
private Animator animator;
private bool isGrounded = false;
private float horizontalInput = 0.0f;
private bool isJumping = false;
void Start()
{
rb = GetComponent<Rigidbody2D>();
animator = GetComponent<Animator>();
}
void Update()
{
horizontalInput = Input.GetAxisRaw("Horizontal");
if (Input.GetButtonDown("Jump") && isGrounded)
{
isJumping = true;
}
}
void FixedUpdate()
{
// 水平移动
rb.velocity = new Vector2(horizontalInput * speed, rb.velocity.y);
// 检测是否在地面上
RaycastHit2D hit = Physics2D.Raycast(transform.position, Vector2.down, 0.1f, groundLayer);
if (hit.collider != null)
{
isGrounded = true;
}
else
{
isGrounded = false;
}
// 跳跃
if (isJumping)
{
rb.velocity = new Vector2(rb.velocity.x, jumpForce);
isJumping = false;
}
// 动画控制
animator.SetFloat("Speed", Mathf.Abs(rb.velocity.x));
animator.SetBool("IsGrounded", isGrounded);
}
}
```
这个代码实现了玩家的左右移动、跳跃、动画控制以及检测是否在地面上。你可以将它附加到玩家对象上并进行测试。
写一段Unity 2d平台 移动条约代码
以下是一个简单的Unity 2D平台移动条约代码示例:
```csharp
public class PlayerController : MonoBehaviour
{
public float moveSpeed = 5f;
public float jumpForce = 10f;
private bool isGrounded;
private Rigidbody2D rb;
void Start()
{
rb = GetComponent<Rigidbody2D>();
}
void Update()
{
float horizontalInput = Input.GetAxis("Horizontal");
rb.velocity = new Vector2(horizontalInput * moveSpeed, rb.velocity.y);
if (Input.GetButtonDown("Jump") && isGrounded)
{
rb.AddForce(Vector2.up * jumpForce, ForceMode2D.Impulse);
isGrounded = false;
}
}
void OnCollisionEnter2D(Collision2D collision)
{
if (collision.gameObject.CompareTag("Ground"))
{
isGrounded = true;
}
}
}
```
这段代码实现了基本的平台移动和跳跃功能,其中 `moveSpeed` 控制移动速度,`jumpForce` 控制跳跃力度。`Update` 函数中通过 `Input.GetAxis` 获取水平输入,将其乘以移动速度得到水平速度,然后通过 `Rigidbody2D.velocity` 来控制对象的移动。如果玩家按下跳跃键并且当前处于地面上,就会添加一个向上的冲量,然后将 `isGrounded` 标志设置为 `false`。在 `OnCollisionEnter2D` 函数中,如果玩家碰到了地面,就将 `isGrounded` 标志设置为 `true`。
相关推荐
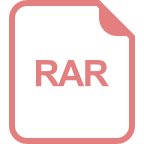









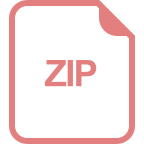
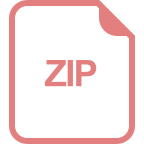
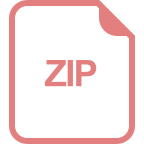