二元交叉熵损失函数torch
时间: 2023-09-07 21:18:11 浏览: 71
在PyTorch中,可以使用`torch.nn.BCELoss`类来计算二元交叉熵损失函数。BCE表示二元交叉熵(Binary Cross Entropy)。
以下是一个简单的示例代码,展示了如何在PyTorch中使用二元交叉熵损失函数:
```python
import torch
import torch.nn as nn
# 假设有一批二分类任务的预测概率和对应的真实标签
predictions = torch.Tensor([0.2, 0.8, 0.6]) # 预测概率
labels = torch.Tensor([0, 1, 1]) # 真实标签(0表示负样本,1表示正样本)
# 创建损失函数实例
loss_function = nn.BCELoss()
# 计算二元交叉熵损失
loss = loss_function(predictions, labels)
print(loss)
```
输出结果将是一个标量,表示计算得到的二元交叉熵损失值。
注意:在使用`torch.nn.BCELoss`时,输入的预测概率应该在[0, 1]的范围内,并且具有浮点数据类型。真实标签应该是与预测概率相同大小的张量,并且使用0和1表示负样本和正样本。
相关问题
torch的损失函数
torch中常用的损失函数有很多种,以下是其中几种常见的:
1. nn.MSELoss:均方误差损失函数,用于回归问题。
2. nn.CrossEntropyLoss:交叉熵损失函数,用于分类问题。
3. nn.BCELoss:二元交叉熵损失函数,用于二分类问题。
4. nn.NLLLoss:负对数似然损失函数,用于多分类问题。
还有很多其他的损失函数,可以根据具体问题选择合适的损失函数。
使用Pytorch完成逻辑回归问题 1.创建一些随机数据,并将其转换为Tensor类型 随机数据代码: np.random.seed(0) X = np.random.randn(100, 2) Y = np.zeros((100,)) Y[X[:,0] + X[:,1] > 0] = 1 2.定义一个逻辑回归模型(使用nn.Linear作为线性层,使用torch.sigmoid作为激活函数); 3.定义损失函数(使用二元交叉熵损失函数)和优化器(使用随机梯度下降法); 4.将模型训练指定轮数,每轮进行前向传播、反向传播和参数更新,并输出损失函数值; 5.输出模型参数并可视化结果。
好的,我来为您讲解如何使用PyTorch完成逻辑回归问题。
首先,您需要将随机数据转换为Tensor类型,可以使用以下代码:
```python
import numpy as np
import torch
# 创建随机数据
np.random.seed(0)
X = np.random.randn(100, 2)
Y = np.zeros((100,))
Y[X[:,0] + X[:,1] > 0] = 1
# 转换为Tensor类型
X = torch.from_numpy(X).float()
Y = torch.from_numpy(Y).float()
```
接下来,定义一个逻辑回归模型,使用nn.Linear作为线性层,使用torch.sigmoid作为激活函数。
```python
import torch.nn as nn
# 定义逻辑回归模型
class LogisticRegression(nn.Module):
def __init__(self):
super(LogisticRegression, self).__init__()
self.linear = nn.Linear(2, 1)
def forward(self, x):
y_pred = torch.sigmoid(self.linear(x))
return y_pred
model = LogisticRegression()
```
然后,定义损失函数和优化器,使用二元交叉熵损失函数和随机梯度下降法。
```python
# 定义损失函数和优化器
criterion = nn.BCELoss()
optimizer = torch.optim.SGD(model.parameters(), lr=0.01)
```
接下来,将模型训练指定轮数,每轮进行前向传播、反向传播和参数更新,并输出损失函数值。
```python
# 训练模型
num_epochs = 1000
for epoch in range(num_epochs):
# 前向传播
y_pred = model(X)
# 计算损失函数
loss = criterion(y_pred, Y.unsqueeze(1))
# 反向传播和优化
optimizer.zero_grad()
loss.backward()
optimizer.step()
# 输出损失函数值
if (epoch+1) % 100 == 0:
print('Epoch [{}/{}], Loss: {:.4f}'.format(epoch+1, num_epochs, loss.item()))
```
最后,输出模型参数并可视化结果。
```python
# 输出模型参数
print('模型参数: ', list(model.parameters()))
# 可视化结果
import matplotlib.pyplot as plt
plt.scatter(X[:,0], X[:,1], c=Y)
x_min, x_max = X[:, 0].min() - .5, X[:, 0].max() + .5
y_min, y_max = X[:, 1].min() - .5, X[:, 1].max() + .5
xx, yy = np.meshgrid(np.linspace(x_min, x_max, 200), np.linspace(y_min, y_max, 200))
X_test = torch.from_numpy(np.c_[xx.ravel(), yy.ravel()]).float()
Z = model(X_test).detach().numpy()
Z = Z.reshape(xx.shape)
plt.contourf(xx, yy, Z, cmap=plt.cm.Spectral, alpha=0.8)
plt.show()
```
希望这些代码可以帮助您完成逻辑回归问题。
相关推荐
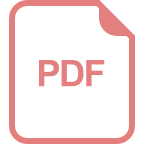
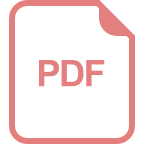
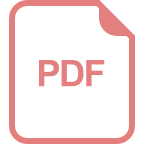











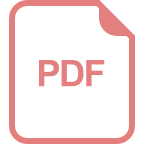
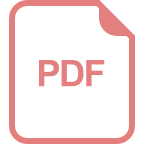