用c语言将稀疏矩阵转换为三元组表
时间: 2024-11-16 10:19:54 浏览: 54
在C语言中,将稀疏矩阵转换为三元组表通常是为了方便存储和处理那些非零元素较少的矩阵,因为传统的二维数组可能会浪费大量空间。三元组表(也称为“三元组列表”或“coo格式”)通过三个变量分别表示行索引、列索引和值,每个非零元素占据一行。
下面是一个简单的示例,如何使用结构体和循环来完成这个转换:
```c
#include <stdio.h>
// 定义稀疏矩阵的三元组结构体
typedef struct {
int row; // 行索引
int col; // 列索引
double val; // 元素值
} sparse_triplet;
// 函数原型,接收稀疏矩阵和大小
void sparse_to_triplets(int matrix[][size], int size, sparse_triplet* triplets);
int main() {
int sparse_matrix[size][size]; // 稀疏矩阵
int rows, cols;
printf("输入稀疏矩阵的行数和列数:");
scanf("%d %d", &rows, &cols);
// 用户输入稀疏矩阵的非零元素
printf("请输入稀疏矩阵的非零元素(行列值和对应值,直到结束):\n");
for (int i = 0; i < rows * cols; ++i) {
int r, c, v;
scanf("%d %d %lf", &r, &c, &v);
sparse_matrix[r][c] = v;
}
sparse_triplet triplets[rows * cols]; // 存储三元组的数组
int triplet_count = 0;
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
if (sparse_matrix[i][j] != 0) {
triplets[triplet_count].row = i;
triplets[triplet_count].col = j;
triplets[triplet_count].val = sparse_matrix[i][j];
++triplet_count;
}
}
}
// 输出三元组表
printf("稀疏矩阵转换后的三元组表:\n");
for (int i = 0; i < triplet_count; ++i) {
printf("(%d,%d,%lf)\n", triplets[i].row, triplets[i].col, triplets[i].val);
}
return 0;
}
阅读全文
相关推荐
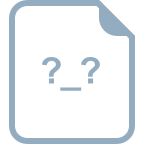
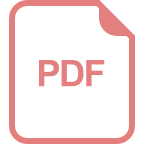
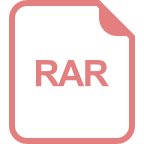















