weights_initialize = model.get_weights(), model.set_weights(weights_initialize)如何转化为pytorchg格式
时间: 2024-02-19 09:03:23 浏览: 19
在 PyTorch 中,我们可以使用 `state_dict()` 方法获取模型的参数字典,也可以使用 `load_state_dict()` 方法将参数字典加载到模型中。因此,我们可以通过以下代码将 Keras 模型的参数转换为 PyTorch 模型的参数:
```python
import torch
import numpy as np
# 加载 Keras 模型
keras_model = ...
# 获取 Keras 模型参数
keras_weights = keras_model.get_weights()
# 转换参数为 PyTorch 格式
pytorch_weights = []
for i in range(len(keras_weights)):
weight = torch.from_numpy(np.array(keras_weights[i]))
pytorch_weights.append(weight)
# 加载参数到 PyTorch 模型
pytorch_model = ...
pytorch_model.load_state_dict({f'weight_{i}': w for i, w in enumerate(pytorch_weights)})
```
这里假设 PyTorch 模型的权重名称为 `weight_i`,可以根据实际情况进行修改。
相关问题
weights_initialize = model.get_weights(), model.set_weights(weights_initialize)
`weights_initialize = model.get_weights()` 是在 Keras 中获取模型的参数,`model.set_weights(weights_initialize)` 是将参数设置回模型中。
在 PyTorch 中,获取模型的参数可以使用 `state_dict()` 方法,将参数设置回模型可以使用 `load_state_dict()` 方法。
例如,以下代码展示了如何在 PyTorch 中获取模型参数并将其设置回模型中:
```python
import torch
# 加载 PyTorch 模型
pytorch_model = ...
# 获取模型参数
pytorch_weights = pytorch_model.state_dict()
# 将模型参数设置回模型中
pytorch_model.load_state_dict(pytorch_weights)
```
需要注意的是,PyTorch 和 Keras 的模型结构和参数定义方式不同,因此在进行模型转换时需要注意参数的维度和名称,以保证模型转换后的正确性。
weights_initialize = model.get_weights(),model.set_weights(weights_initialize)代码意思
这两行代码是用来获取和设置神经网络模型参数的方法。
`weights_initialize = model.get_weights()`会返回一个包含神经网络模型所有权重和偏置等参数的列表。这个列表中的每个元素都是一个NumPy数组,代表了模型中的每个权重或偏置。
`model.set_weights(weights_initialize)`会将这个列表中的参数设置回神经网络模型中。这个方法可以用于将预训练模型的参数加载到神经网络模型中,或者将一个模型的参数复制到另一个模型中。
总之,`model.get_weights()`和`model.set_weights(weights_initialize)`这两个方法可以帮助我们获取和设置神经网络模型的参数,以便在训练和优化模型时使用。
相关推荐
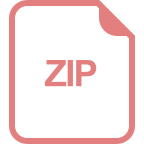
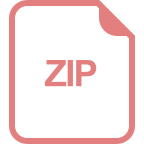













