启发式八数码问题python
时间: 2023-10-28 22:01:57 浏览: 87
对于八数码问题,可以使用启发式搜索算法来求解,其中最常用的启发函数是曼哈顿距离。
以下是一个使用Python实现的启发式八数码问题的示例代码:
```python
import heapq
import numpy as np
# 定义目标状态
goal_state = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 0]])
# 计算曼哈顿距离
def manhattan_distance(state):
distance = 0
for i in range(3):
for j in range(3):
if state[i][j] != 0:
goal_position = np.argwhere(goal_state == state[i][j])[0]
distance += abs(i - goal_position[0]) + abs(j - goal_position[1])
return distance
# 获取移动后的状态
def get_next_state(state, move):
i, j = np.argwhere(state == 0)[0]
if move == 'up' and i > 0:
state[i][j], state[i - 1][j] = state[i - 1][j], state[i][j]
elif move == 'down' and i < 2:
state[i][j], state[i + 1][j] = state[i + 1][j], state[i][j]
elif move == 'left' and j > 0:
state[i][j], state[i][j - 1] = state[i][j - 1], state[i][j]
elif move == 'right' and j < 2:
state[i][j], state[i][j + 1] = state[i][j + 1], state[i][j]
return state
# 启发式搜索算法
def solve_puzzle(start_state):
heap = []
heapq.heappush(heap, (manhattan_distance(start_state), start_state, 0))
visited = set()
while len(heap) > 0:
_, current_state, moves = heapq.heappop(heap)
if np.array_equal(current_state, goal_state):
return moves
visited.add(tuple(map(tuple, current_state)))
for next_move in ['up', 'down', 'left', 'right']:
next_state = get_next_state(current_state.copy(), next_move)
if tuple(map(tuple, next_state)) not in visited:
heapq.heappush(heap, (manhattan_distance(next_state) + moves + 1, next_state, moves + 1))
return -1
# 示例用法
start_state = np.array([[2, 8, 3], [1, 6, 4], [7, 0, 5]])
moves = solve_puzzle(start_state)
print("最少移动步数为:", moves)
```
在上述代码中,我们使用了A*搜索算法来解决八数码问题。通过计算每个状态与目标状态之间的曼哈顿距离,选择启发函数来估计当前状态与目标状态之间的距离。然后,我们使用优先队列来存储待搜索的状态,并按照启发函数值进行排序。在搜索的过程中,我们不断选择最优的状态进行扩展,直到找到目标状态或无解。最后,输出最少移动步数。
请注意,这只是一个简单的示例代码,实际上八数码问题可以应用更多的优化技巧和算法来提高效率。
相关推荐
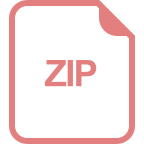
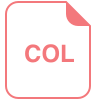













