基于 Python 的八数码问题的深度、广度搜索 与启发式算法分析代码
时间: 2024-04-30 09:20:56 浏览: 110
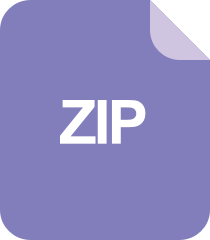
python深度,广度,三种启发式搜索解决八数码问题
八数码问题是一个经典的人工智能问题,可以使用深度搜索、广度搜索和启发式算法等多种方法进行求解。下面给出基于 Python 的八数码问题的深度、广度搜索和启发式算法的代码实现。
首先定义一个表示八数码问题状态的类 `State`,其中 `board` 表示当前状态的棋盘布局,`parent` 表示当前状态的父状态,`action` 表示当前状态到达该状态的操作,`depth` 表示当前状态的深度。
```python
class State:
def __init__(self, board, parent, action, depth):
self.board = board
self.parent = parent
self.action = action
self.depth = depth
```
接下来定义一个函数 `get_blank_pos`,用于获取空格(0)的位置。
```python
def get_blank_pos(board):
for i in range(3):
for j in range(3):
if board[i][j] == 0:
return i, j
```
定义一个函数 `get_successors`,用于获取当前状态的所有合法后继状态。
```python
def get_successors(state):
successors = []
blank_i, blank_j = get_blank_pos(state.board)
for action, (di, dj) in ACTIONS.items():
new_i, new_j = blank_i + di, blank_j + dj
if 0 <= new_i < 3 and 0 <= new_j < 3:
new_board = [row[:] for row in state.board]
new_board[blank_i][blank_j], new_board[new_i][new_j] = new_board[new_i][new_j], new_board[blank_i][blank_j]
successors.append(State(new_board, state, action, state.depth + 1))
return successors
```
定义一个函数 `is_goal`,用于判断当前状态是否为目标状态。
```python
def is_goal(state):
return state.board == GOAL_STATE
```
接下来实现深度搜索算法,定义一个函数 `depth_first_search`,使用栈(Stack)作为数据结构。
```python
def depth_first_search(initial_state):
stack = [initial_state]
visited = set()
while stack:
state = stack.pop()
if is_goal(state):
return state
visited.add(tuple(map(tuple, state.board)))
successors = get_successors(state)
for successor in reversed(successors):
if tuple(map(tuple, successor.board)) not in visited:
stack.append(successor)
return None
```
实现广度搜索算法,定义一个函数 `breadth_first_search`,使用队列(Queue)作为数据结构。
```python
def breadth_first_search(initial_state):
queue = collections.deque([initial_state])
visited = set()
while queue:
state = queue.popleft()
if is_goal(state):
return state
visited.add(tuple(map(tuple, state.board)))
successors = get_successors(state)
for successor in successors:
if tuple(map(tuple, successor.board)) not in visited:
queue.append(successor)
return None
```
接下来实现启发式搜索算法,使用曼哈顿距离作为启发函数,定义一个函数 `manhattan_distance`,用于计算当前状态到目标状态的曼哈顿距离。
```python
def manhattan_distance(board):
distance = 0
for i in range(3):
for j in range(3):
if board[i][j] != 0:
row, col = divmod(board[i][j] - 1, 3)
distance += abs(row - i) + abs(col - j)
return distance
```
定义一个函数 `a_star_search`,使用优先队列(Priority Queue)作为数据结构。
```python
def a_star_search(initial_state):
queue = [(manhattan_distance(initial_state.board), initial_state)]
visited = set()
while queue:
_, state = heappop(queue)
if is_goal(state):
return state
visited.add(tuple(map(tuple, state.board)))
successors = get_successors(state)
for successor in successors:
if tuple(map(tuple, successor.board)) not in visited:
heappush(queue, (manhattan_distance(successor.board) + successor.depth, successor))
return None
```
最后定义一些常量和操作字典。
```python
import collections
from heapq import heappush, heappop
INITIAL_STATE = [
[2, 8, 3],
[1, 6, 4],
[7, 0, 5]
]
GOAL_STATE = [
[1, 2, 3],
[8, 0, 4],
[7, 6, 5]
]
ACTIONS = {
'Up': (-1, 0),
'Down': (1, 0),
'Left': (0, -1),
'Right': (0, 1)
}
```
可以使用以下代码测试以上算法:
```python
initial_state = State(INITIAL_STATE, None, None, 0)
print('Depth First Search:')
result = depth_first_search(initial_state)
if result is not None:
print('Solution found in', result.depth, 'steps')
while result.parent is not None:
print(result.action)
result = result.parent
else:
print('No solution found')
print('Breadth First Search:')
result = breadth_first_search(initial_state)
if result is not None:
print('Solution found in', result.depth, 'steps')
while result.parent is not None:
print(result.action)
result = result.parent
else:
print('No solution found')
print('A* Search:')
result = a_star_search(initial_state)
if result is not None:
print('Solution found in', result.depth, 'steps')
while result.parent is not None:
print(result.action)
result = result.parent
else:
print('No solution found')
```
阅读全文
相关推荐
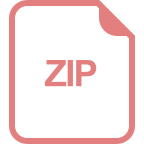
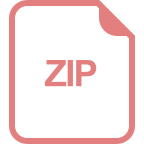















