hybrid a*算法 matlab
时间: 2023-07-26 07:03:39 浏览: 59
Hybrid A*算法是一种路径规划算法,是 A* 算法的改进版。它将 A* 算法与连续状态空间规划方法(例如 DDP,Dynamic Programming)相结合,可以在二维或三维空间中规划车辆或机器人的路径。
Matlab 是一款数学软件,可以用于编写 Hybrid A* 算法的程序。下面是一个简单的 Matlab 代码示例,可用于实现 Hybrid A* 算法:
```
function [path, pathcost] = hybrid_a_star(start, goal, obstacles)
% 初始化参数
nodes = [];
nodes(1).x = start(1);
nodes(1).y = start(2);
nodes(1).theta = start(3);
nodes(1).cost = 0;
nodes(1).parent = 0;
nodes(1).f = heuristic_cost_estimate(nodes(1), goal);
closed = [];
opened(1) = nodes(1);
% 开始搜索
while ~isempty(opened)
% 选择最小代价节点
[minf, minfidx] = min([opened.f]);
current = opened(minfidx);
% 到达目标点,返回路径
if sqrt((current.x-goal(1))^2 + (current.y-goal(2))^2) < 0.1
path = [goal(1) goal(2)];
pathcost = current.cost;
while current.parent ~= 0
path = [current.x current.y; path];
current = nodes(current.parent);
end
path = [start(1) start(2); path];
return;
end
% 将节点从开放列表中删除,并加入关闭列表
opened(minfidx) = [];
closed = [closed current];
% 生成子节点
for i=-35:5:35
node.x = current.x + cosd(current.theta+i) * 0.1;
node.y = current.y + sind(current.theta+i) * 0.1;
node.theta = current.theta + i*pi/180;
node.cost = current.cost + 0.1;
node.parent = length(nodes);
node.f = node.cost + heuristic_cost_estimate(node, goal);
if ~collision_check(node, obstacles)
continue;
end
for j=1:length(closed)
if isequal(node, closed(j))
continue;
end
end
for j=1:length(opened)
if isequal(node, opened(j))
if node.cost < opened(j).cost
opened(j) = node;
end
continue;
end
end
nodes = [nodes node];
opened = [opened node];
end
end
% 没有找到路径
path = [];
pathcost = 0;
end
% 估计启发式代价
function h = heuristic_cost_estimate(node, goal)
h = sqrt((node.x-goal(1))^2 + (node.y-goal(2))^2);
end
% 碰撞检查
function flag = collision_check(node, obstacles)
flag = true;
for i=1:size(obstacles,1)
if sqrt((node.x-obstacles(i,1))^2 + (node.y-obstacles(i,2))^2) < obstacles(i,3)
flag = false;
break;
end
end
end
```
在上面的代码中,我们首先定义了一个节点结构体,包含节点的位置、角度、代价、父节点和估计代价等信息。然后,我们初始化了起点节点,将其加入开放列表中。接着,我们在 while 循环中进行搜索,每次选择开放列表中代价最小的节点进行扩展,并将其从开放列表中删除,加入关闭列表中。在生成子节点时,我们将车辆或机器人的运动限制在 -35 到 35 度之间,每次增加 5 度,生成多个子节点。对于每个子节点,我们计算其代价和估计代价,并进行碰撞检查,如果没有碰撞,则将其加入开放列表中。最后,如果找到了目标点,我们将返回路径;否则,返回空路径。
需要注意的是,上述代码仅为示例代码,实际应用中可能需要进行更多的优化和改进,以适应不同的应用场景。
相关推荐
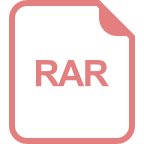
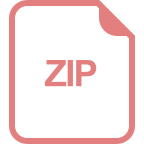
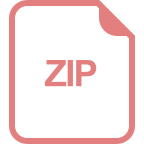














