在Python中,如何结合使用sklearn和matplotlib库来绘制鸢尾花数据集上逻辑回归模型的混淆矩阵,并解释其性能评估的含义?
时间: 2024-11-01 13:12:56 浏览: 61
在Python中绘制混淆矩阵,特别是针对鸢尾花数据集上的逻辑回归模型,可以通过scikit-learn库来实现。首先,你需要导入必要的库,如`numpy`、`sklearn`中的`datasets`、`model_selection`和`metrics`模块,以及`matplotlib.pyplot`用于绘图。以下是详细步骤和代码示例:
参考资源链接:[Python实现与鸢尾花数据集的混淆矩阵可视化](https://wenku.csdn.net/doc/6xr7gganpx?spm=1055.2569.3001.10343)
1. **加载数据集**:使用`sklearn.datasets.load_iris()`函数加载鸢尾花数据集,并将数据集分为特征和标签。
2. **数据分割**:使用`sklearn.model_selection.train_test_split()`函数将数据集分为训练集和测试集,以训练和评估模型。
3. **模型训练**:创建一个`LogisticRegression`实例,并用训练集数据拟合模型。
4. **模型预测**:使用训练好的模型对测试集进行预测,得到预测结果。
5. **生成混淆矩阵**:使用`sklearn.metrics.confusion_matrix()`函数,传入测试集的真实标签和预测标签,得到混淆矩阵。
6. **绘制混淆矩阵**:使用`matplotlib.pyplot`中的`matshow()`函数绘制混淆矩阵,并添加标题、颜色条和标签。
7. **性能评估指标解释**:通过混淆矩阵可以计算出模型的精确度、召回率和F1分数等性能评估指标,并对这些指标的含义进行解释,以评估模型对每个类别的识别能力。
具体代码如下:
```python
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import confusion_matrix
import numpy as np
import matplotlib.pyplot as plt
# 加载数据集
iris = load_iris()
X = iris.data
y = iris.target
# 数据分割
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# 模型训练
model = LogisticRegression(max_iter=200)
model.fit(X_train, y_train)
# 模型预测
y_pred = model.predict(X_test)
# 生成混淆矩阵
conf_matrix = confusion_matrix(y_test, y_pred)
# 绘制混淆矩阵
plt.matshow(conf_matrix)
plt.title('Confusion Matrix')
plt.colorbar()
tick_marks = np.arange(len(iris.target_names))
plt.xticks(tick_marks, iris.target_names, rotation=45)
plt.yticks(tick_marks, iris.target_names)
plt.xlabel('Predicted label')
plt.ylabel('True label')
# 性能评估指标解释
tn, fp, fn, tp = conf_matrix.ravel()
precision = tp / (tp + fp)
recall = tp / (tp + fn)
f1_score = 2 * precision * recall / (precision + recall)
print(f'Precision: {precision}')
print(f'Recall: {recall}')
print(f'F1 Score: {f1_score}')
plt.show()
```
通过上述步骤,你不仅可以绘制出混淆矩阵,还可以深入理解模型在鸢尾花数据集上的分类性能。为了进一步提高你的技能,建议阅读《Python实现与鸢尾花数据集的混淆矩阵可视化》这份资料,它不仅详细解释了上述过程,还提供了更多关于性能评估指标的背景知识和可视化工具的使用技巧。
参考资源链接:[Python实现与鸢尾花数据集的混淆矩阵可视化](https://wenku.csdn.net/doc/6xr7gganpx?spm=1055.2569.3001.10343)
阅读全文
相关推荐
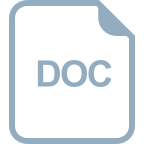
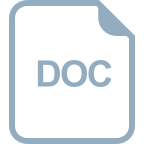
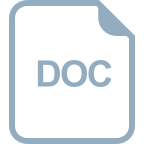

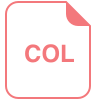













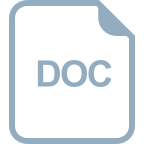