Freertos下基于君正X2000芯片进行uart串口的读写编程
时间: 2024-02-03 16:15:55 浏览: 140
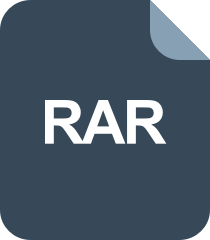
FPGA的UART串口读写
在FreeRTOS下,使用君正X2000芯片进行UART串口的读写编程需要以下步骤:
1. 配置UART串口的GPIO引脚和时钟。
2. 初始化UART串口模块,并设置波特率、数据位数、停止位数等参数。
3. 创建一个空闲任务(Idle Task),该任务会在系统空闲时执行。
4. 在空闲任务中调用UART串口的读写函数,实现数据的读写操作。
下面是一个示例代码,用于在FreeRTOS下实现君正X2000芯片的UART串口读写功能:
```c
#include "FreeRTOS.h"
#include "task.h"
#include "uart.h"
#define UART_RX_BUF_SIZE 128
#define UART_TX_BUF_SIZE 128
static uint8_t uart_rx_buf[UART_RX_BUF_SIZE];
static uint8_t uart_tx_buf[UART_TX_BUF_SIZE];
void uart_task(void *pvParameters)
{
uart_handle_t uart_handle;
uart_config_t uart_config;
uint8_t ch;
// 配置UART串口的GPIO引脚和时钟
uart_gpio_init();
uart_clock_init();
// 初始化UART串口模块,并设置波特率、数据位数、停止位数等参数
uart_config.baud_rate = 115200;
uart_config.data_bits = UART_DATA_8_BITS;
uart_config.stop_bits = UART_STOP_1_BIT;
uart_config.parity = UART_PARITY_DISABLE;
uart_config.flow_ctrl = UART_HW_FLOWCTRL_DISABLE;
uart_handle = uart_init(UART_NUM_0, &uart_config);
while (1) {
// 读取UART串口接收缓冲区中的数据
while (uart_read_bytes(uart_handle, &ch, 1, 0) == 1) {
uart_rx_buf[0] = ch;
// 处理接收到的数据
// ...
}
// 发送UART串口发送缓冲区中的数据
if (uart_get_buffered_data_len(uart_handle) == 0) {
uart_write_bytes(uart_handle, uart_tx_buf, UART_TX_BUF_SIZE);
}
// 延时一段时间
vTaskDelay(10);
}
}
int main(void)
{
// 创建一个空闲任务(Idle Task),该任务会在系统空闲时执行
xTaskCreate(uart_task, "uart_task", configMINIMAL_STACK_SIZE, NULL, tskIDLE_PRIORITY, NULL);
// 启动FreeRTOS调度器
vTaskStartScheduler();
while (1) {
// 不会执行到这里
}
return 0;
}
```
需要注意的是,以上代码中的 `uart_gpio_init()` 和 `uart_clock_init()` 函数需要根据具体的芯片进行实现。此外,还需要根据实际情况调整串口缓冲区大小和延时时间等参数。
阅读全文
相关推荐


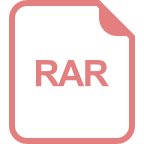
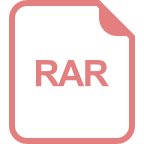
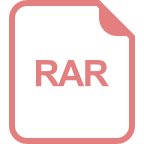
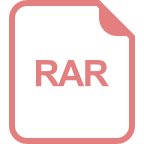
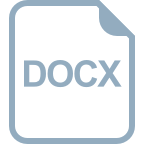
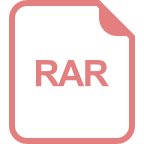
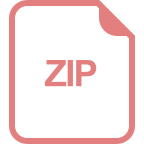
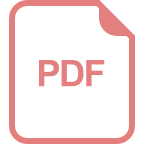
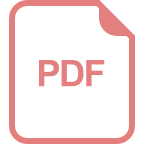
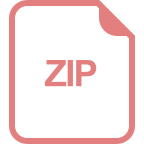
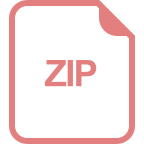
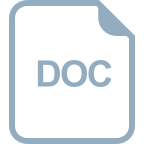
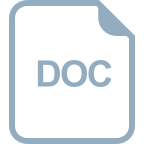