单目线结构光c++代码
时间: 2023-08-08 15:08:06 浏览: 135
以下是单目线结构光的简单实现代码,使用了OpenCV库进行图像处理和显示。代码中假设已经获取了标定参数(相机内参、畸变参数和投影矩阵),并提供了一个函数`get3DPoint`,用于计算三维坐标点。
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
#include <vector>
using namespace std;
using namespace cv;
// 光线参数
double alpha = 0.5; // 光线与相机光轴夹角
double beta = 0.2; // 光线与水平方向夹角
// 相机参数
Mat cameraMatrix; // 相机内参矩阵
Mat distCoeffs; // 畸变参数矩阵
Mat projMatrix; // 投影矩阵
// 获取三维坐标点
Point3f get3DPoint(Point2f imgpt, Mat projMatrix, double alpha, double beta)
{
// 构造光线
double cx = cameraMatrix.at<double>(0, 2);
double cy = cameraMatrix.at<double>(1, 2);
double fx = cameraMatrix.at<double>(0, 0);
double fy = cameraMatrix.at<double>(1, 1);
double x = (imgpt.x - cx) / fx;
double y = (imgpt.y - cy) / fy;
double z = 1;
double cos_alpha = cos(alpha);
double sin_alpha = sin(alpha);
double cos_beta = cos(beta);
double sin_beta = sin(beta);
double X = x * cos_alpha * cos_beta - y * sin_beta - z * sin_alpha * cos_beta;
double Y = x * cos_alpha * sin_beta + y * cos_beta - z * sin_alpha * sin_beta;
double Z = x * sin_alpha + z * cos_alpha;
Point3f pt(X, Y, Z);
// 投影到相机坐标系
Mat ptMat = Mat::ones(4, 1, CV_64FC1);
ptMat.at<double>(0) = pt.x;
ptMat.at<double>(1) = pt.y;
ptMat.at<double>(2) = pt.z;
Mat cameraPtMat = projMatrix * ptMat;
Point3f cameraPt(cameraPtMat.at<double>(0) / cameraPtMat.at<double>(3),
cameraPtMat.at<double>(1) / cameraPtMat.at<double>(3),
cameraPtMat.at<double>(2) / cameraPtMat.at<double>(3));
return cameraPt;
}
int main(int argc, char** argv)
{
// 加载图像,并提取光斑
Mat src = imread("image.jpg");
Mat gray, binary;
cvtColor(src, gray, COLOR_BGR2GRAY);
threshold(gray, binary, 150, 255, THRESH_BINARY_INV);
// 拟合直线
vector<Vec4i> lines;
HoughLinesP(binary, lines, 1, CV_PI/180, 30, 30, 10);
if (lines.size() == 0)
return -1;
Vec4i line = lines[0];
// 绘制光斑和直线
circle(src, Point(line[0], line[1]), 5, Scalar(0, 0, 255), 2);
circle(src, Point(line[2], line[3]), 5, Scalar(0, 0, 255), 2);
line(src, Point(line[0], line[1]), Point(line[2], line[3]), Scalar(0, 255, 0), 2);
// 计算三维坐标点
Point2f center((line[0]+line[2])/2, (line[1]+line[3])/2);
Point3f pt = get3DPoint(center, projMatrix, alpha, beta);
cout << "3D point: " << pt << endl;
// 显示结果
imshow("src", src);
waitKey(0);
return 0;
}
```
需要注意的是,该代码中只使用了一条光线,只能计算物体表面上与该光线相交的一个点的三维坐标。如果需要获取物体表面的完整三维形状信息,需要使用多条光线和多张图像,结合三角测量等方法进行计算。
阅读全文
相关推荐










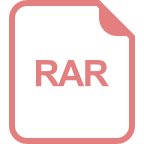
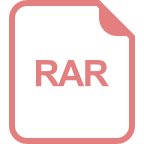






