import pandas as pd import numpy as np import sklearn from sklearn.neural_network import MLPClassifier from sklearn.model_selection import train_test_split df=pd.read_csv("D:/Users/dell/Desktop/taxDetection.csv") data=np.array(df) x=data[:,:11] y=data[:,11] #axis=0表示输出矩阵是1行,也就是求每一列的平均值 x_mean = x.mean(axis =0) x_std = x.std(axis=0) x_scaled = (x-x_mean) / x_std #random_state:int 或RandomState,可选,默认None,随机数生成器的状态或种子。 X_train, X_test, y_train, y_test =train_test_split(x_scaled,y,test_size=0.25,random_state = 0,stratify=y) #hidden_layer_sizes :例如hidden_layer_sizes=(50, 50),表示有两层隐藏层,第一层隐藏层有50个神经元,第二层也有50个神经元 #sgd:随机梯度下降 #max_iter: int,可选,默认200,最大迭代次数 a=MLPClassifier(solver='sgd',hidden_layer_sizes=(50,50,50),max_iter=3000) a.fit(X_train,y_train) print("Accuracy on training set:{:.2f}".format(a.score(X_train,y_train))) print("Accuracy on test set:{:.2f}".format(a.score(X_test,y_test)))在这段代码中加入特征工程
时间: 2023-12-24 11:12:37 浏览: 48
以下是一个可以对给定的代码进行特征工程的示例:
```python
import pandas as pd
import numpy as np
from sklearn.neural_network import MLPClassifier
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
# 加载数据
df = pd.read_csv("D:/Users/dell/Desktop/taxDetection.csv")
data = np.array(df)
x = data[:,:11]
y = data[:,11]
# 特征工程
scaler = StandardScaler()
x_scaled = scaler.fit_transform(x)
# 划分训练集和测试集
X_train, X_test, y_train, y_test =train_test_split(x_scaled, y, test_size=0.25, random_state=0, stratify=y)
# 训练神经网络模型
mlp = MLPClassifier(solver='sgd', hidden_layer_sizes=(50,50,50), max_iter=3000)
mlp.fit(X_train, y_train)
# 输出模型精度
print("Accuracy on training set: {:.2f}".format(mlp.score(X_train, y_train)))
print("Accuracy on test set: {:.2f}".format(mlp.score(X_test, y_test)))
```
这段代码使用了 `StandardScaler` 类进行特征缩放,将原始数据进行标准化处理,使特征数据的均值为0,方差为1。这有助于提高神经网络模型的训练效果。然后,我们使用 `train_test_split` 函数将数据划分成训练集和测试集。最后,我们使用 `MLPClassifier` 类训练神经网络模型,并输出模型在训练集和测试集上的精度。需要注意的是,这里只是一个简单的特征工程示例,实际应用中可能需要更复杂的特征工程处理。
相关推荐
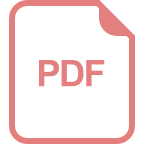














