pytorch实现SDNE代码
时间: 2023-02-09 16:37:12 浏览: 147
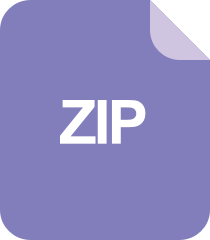
DenseNet pytorch代码
好的,下面是用 PyTorch 实现 SDNE (Structure Deep Network Embedding) 的代码。
首先,我们需要定义一个 SDNE 模型类。
```python
import torch
import torch.nn as nn
class SDNE(nn.Module):
def __init__(self, num_nodes, num_features, hidden_size1, hidden_size2, alpha, beta):
super(SDNE, self).__init__()
self.encoder = nn.Sequential(
nn.Linear(num_features, hidden_size1),
nn.ReLU(),
nn.Linear(hidden_size1, hidden_size2),
nn.ReLU()
)
self.decoder = nn.Sequential(
nn.Linear(hidden_size2, hidden_size1),
nn.ReLU(),
nn.Linear(hidden_size1, num_features),
nn.Sigmoid()
)
self.num_nodes = num_nodes
self.alpha = alpha
self.beta = beta
def forward(self, adjacency_matrix):
embeddings = self.encoder(adjacency_matrix)
reconstructions = self.decoder(embeddings)
# Calculate reconstruction loss
reconstruction_loss = self.alpha * self._reconstruction_loss(adjacency_matrix, reconstructions)
# Calculate regularization loss
regularization_loss = self.beta * self._regularization_loss(embeddings)
return reconstructions, reconstruction_loss, regularization_loss
def _reconstruction_loss(self, input, reconstructions):
reconstruction_loss = nn.MSELoss()(reconstructions, input)
return reconstruction_loss
def _regularization_loss(self, embeddings):
# Calculate the pairwise distance matrix
pairwise_distance = self._pairwise_distance(embeddings)
# Calculate the adjacency matrix of the k-nearest neighbors
adjacency_matrix = self._adjacency_matrix(pairwise_distance)
# Calculate the graph laplacian
laplacian = self._laplacian(adjacency_matrix)
# Calculate the regularization loss
regularization_loss = torch.trace(torch.mm(embeddings, laplacian) @ embeddings.t())
return regularization_loss
def _pairwise_distance(self, embeddings):
# Calculate the pairwise distance matrix
dot_product = torch.mm(embeddings, embeddings.t())
square_norm = torch.diag(dot_product)
pairwise_distance = square_norm.unsqueeze(1) - 2 * dot_product + square_norm.unsqueeze(0)
阅读全文
相关推荐









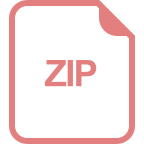
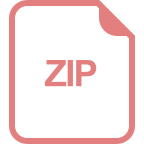